Problem 17 (Unsigned integer radix sort - ): Write a C program for sorting 32-bit unsigned integers using radix sort with a group of 4 bits. Use the variables listed below. Assume 1st is initialized with n numbers. #define N 1048576 #define BIN 256 #define MAXBIT 32 #define LST 1 #define BUF @ int n, group, bin; int flag; /* to show which one holds numbers: 1st or buf */ int 1st [N],buf[N]; int count [BIN], map[BIN], tmap [BIN] int main(int argc, char **argv) { int i; flag = LST; initialize(); /* initialize 1st with n random floats */ for (i=0;i
Problem 17 (Unsigned integer radix sort - ): Write a C program for sorting 32-bit unsigned integers using radix sort with a group of 4 bits. Use the variables listed below. Assume 1st is initialized with n numbers. #define N 1048576 #define BIN 256 #define MAXBIT 32 #define LST 1 #define BUF @ int n, group, bin; int flag; /* to show which one holds numbers: 1st or buf */ int 1st [N],buf[N]; int count [BIN], map[BIN], tmap [BIN] int main(int argc, char **argv) { int i; flag = LST; initialize(); /* initialize 1st with n random floats */ for (i=0;i
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
please fill 1 to 5 and lable it which one is map move count and all
![### Problem 17: Unsigned Integer Radix Sort
**Objective:** Write a C program for sorting 32-bit unsigned integers using radix sort with a group of 4 bits. Use the variables listed below. Assume `lst` is initialized with n numbers.
```c
#define N 1048576
#define BIN 256
#define MAXBIT 32
#define LST 1
#define BUF 0
int n, group, bin;
int flag; /* to show which one holds numbers: lst or buf */
int lst[N], buf[N];
int count[BIN], map[BIN], tmap[BIN];
int main(int argc, char **argv) {
int i;
flag = LST;
initialize(); /* initialize lst with n random floats */
for (i = 0; i < MAXBIT; i += group) radix_sort(i); /* move lst to buf or buf to lst depending on the iteration number */
correct(); /* sorted numbers must be in lst */
}
void radix_sort(int idx) {
int j, k, mask; /* initialize mask for lifting the 4 least significant bits. */
int *src_p, *dst_p; /* cast lst and buf to int pointers to treat lst/buf as int's */
/* set src_p and dst_p */
_____1_____
/* count */
_____2_____
/* map */
_____3_____
/* move */
_____4_____
}
void correct() {
_____5_____
}
```
### Explanation
1. **Definitions and Initialization:**
- `#define N 1048576`: Number of elements in the list.
- `#define BIN 256`: Number of bins used for sorting.
- `#define MAXBIT 32`: Maximum number of bits (for 32-bit integers).
- `#define LST 1`, `#define BUF 0`: Flags to indicate which array (lst or buf) currently holds the numbers.
- Variables `n`, `group`, and `bin` manage sorting and grouping operations.
- `lst` and `buf` are the arrays holding the numbers to be sorted.
- `count`, `map`, and `tmap` are auxiliary arrays for counting and mapping during the sort.
2. **Main Function:**
- Initializes the sorting process.
-](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5bad4e48-dad8-4710-a64b-b24b80d1efcf%2F7576cf54-f267-45b0-9883-7d0fce3ad3a7%2Fj59l4uq_processed.png&w=3840&q=75)
Transcribed Image Text:### Problem 17: Unsigned Integer Radix Sort
**Objective:** Write a C program for sorting 32-bit unsigned integers using radix sort with a group of 4 bits. Use the variables listed below. Assume `lst` is initialized with n numbers.
```c
#define N 1048576
#define BIN 256
#define MAXBIT 32
#define LST 1
#define BUF 0
int n, group, bin;
int flag; /* to show which one holds numbers: lst or buf */
int lst[N], buf[N];
int count[BIN], map[BIN], tmap[BIN];
int main(int argc, char **argv) {
int i;
flag = LST;
initialize(); /* initialize lst with n random floats */
for (i = 0; i < MAXBIT; i += group) radix_sort(i); /* move lst to buf or buf to lst depending on the iteration number */
correct(); /* sorted numbers must be in lst */
}
void radix_sort(int idx) {
int j, k, mask; /* initialize mask for lifting the 4 least significant bits. */
int *src_p, *dst_p; /* cast lst and buf to int pointers to treat lst/buf as int's */
/* set src_p and dst_p */
_____1_____
/* count */
_____2_____
/* map */
_____3_____
/* move */
_____4_____
}
void correct() {
_____5_____
}
```
### Explanation
1. **Definitions and Initialization:**
- `#define N 1048576`: Number of elements in the list.
- `#define BIN 256`: Number of bins used for sorting.
- `#define MAXBIT 32`: Maximum number of bits (for 32-bit integers).
- `#define LST 1`, `#define BUF 0`: Flags to indicate which array (lst or buf) currently holds the numbers.
- Variables `n`, `group`, and `bin` manage sorting and grouping operations.
- `lst` and `buf` are the arrays holding the numbers to be sorted.
- `count`, `map`, and `tmap` are auxiliary arrays for counting and mapping during the sort.
2. **Main Function:**
- Initializes the sorting process.
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
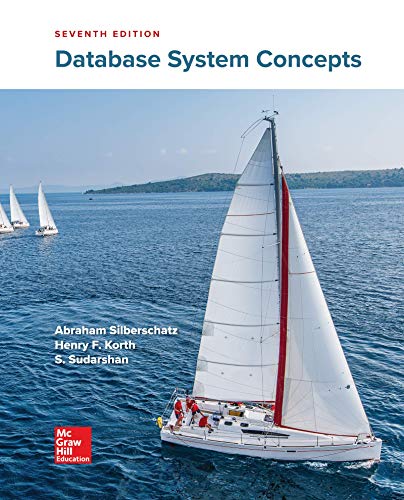
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
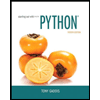
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
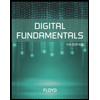
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
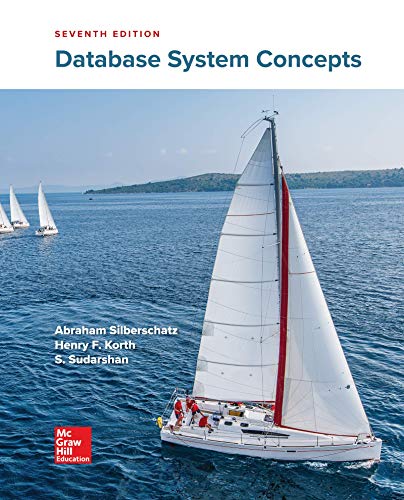
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
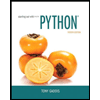
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
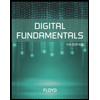
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
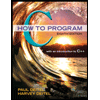
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
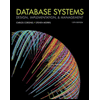
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
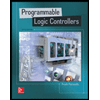
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education