Pretend we work for a company that leases tractors. Let us create a class for tractors that might be used as part of a fleet management system. All of your classes should be in separate files within your project package. Name your package testtractor. Create a class named Tractor. It will have instance variables for the Vehicle ID, Rental Rate, Rental Days. Be sure to make them all private. It will have an method for calculating RentalProfit(). Which will be rental days times rental rate. Create getter and setter methods for the Tractor’s instance variables. Make sure the setter perform reasonable validation. Create a zero parameter constructor and a fully parameterized constructor for the class. Override the toString() method to return the class name, VIN, Rental Days and Rental Rate in a well formatted string. Create an application class named testTractor.java to test drive the tractor class. In your test driver’s main() method create a Tractor object using the zero parameter constructor. Use the setters to assign values to the tractor. Use the toString() to display information about the Tractor. Also create a Tractor using the parameterized constructor. Use its getter methods to display information about the Tractor. Create a third tractor. Use the methods and try to set invalid values. Display the results. Nullify the third tractor object.
Pretend we work for a company that leases tractors. Let us create a class for tractors that might be used as part of a fleet management system. All of your classes should be in separate files within your project package. Name your package testtractor.
Create a class named Tractor. It will have instance variables for the Vehicle ID, Rental Rate, Rental Days. Be sure to make them all private. It will have an method for calculating RentalProfit(). Which will be rental days times rental rate.
Create getter and setter methods for the Tractor’s instance variables. Make sure the setter perform reasonable validation.
Create a zero parameter constructor and a fully parameterized constructor for the class.
Override the toString() method to return the class name, VIN, Rental Days and Rental Rate in a well formatted string.
Create an application class named testTractor.java to test drive the tractor class. In your test driver’s main() method create a Tractor object using the zero parameter constructor. Use the setters to assign values to the tractor. Use the toString() to display information about the Tractor.
Also create a Tractor using the parameterized constructor. Use its getter methods to display information about the Tractor.
Create a third tractor. Use the methods and try to set invalid values. Display the results.
Nullify the third tractor object.

Required Java code is implemented below:
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

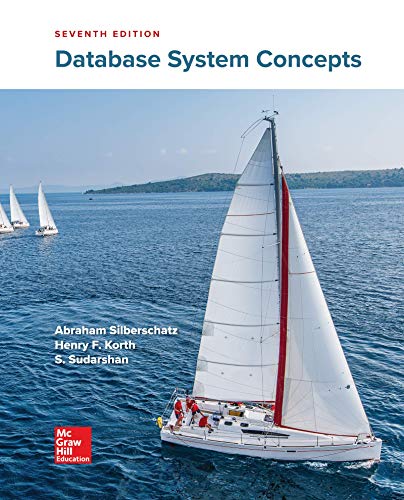
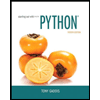
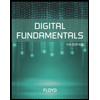
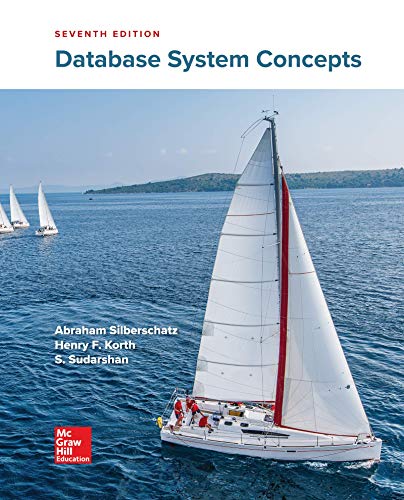
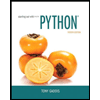
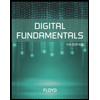
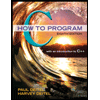
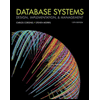
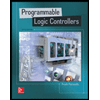