I need to figure out how to call a method that is located in a different class. For example, I am trying to make a tax calculator that multiplies a payers income by a corporate rate. However, I cannot figure out how to call the income method. Nothing I have tried is working. Is there any chance you can guide me? I am just trying to make this equation work. Is there something else that is stopping this from working?
I need to figure out how to call a method that is located in a different class. For example, I am trying to make a tax calculator that multiplies a payers income by a corporate rate. However, I cannot figure out how to call the income method. Nothing I have tried is working. Is there any chance you can guide me? I am just trying to make this equation work. Is there something else that is stopping this from working?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need to figure out how to call a method that is located in a different class. For example, I am trying to make a tax calculator that multiplies a payers income by a corporate rate. However, I cannot figure out how to call the income method. Nothing I have tried is working. Is there any chance you can guide me? I am just trying to make this equation work. Is there something else that is stopping this from working?

Transcribed Image Text:```java
public class TaxCalculator {
double tax;
/**
* Creates a TaxCalculator using 2021-2022 tax rates;
*/
public TaxCalculator() {
}
/**
* Calculates a tax payer's tax assuming they are a corporation.
*
* @precondition payer != null AND payer.isCorporation()==true
* @postcondition none
*
* @param payer the tax payer
*/
public static final double corporaterate = 0.21;
public double calculateCorporateIncomeTax(TaxPayer payer) {
tax = corporaterate * income; // Calculate corporate tax based on income
if (tax < 0) {
tax = 0; // Ensure tax is not negative
return tax;
}
return tax;
}
}
```
### Explanation:
This code defines a `TaxCalculator` class designed to calculate taxes for corporate entities using a specified tax rate.
- **Attributes and Methods**:
- `double tax;` - A variable to store the calculated tax.
- `TaxCalculator()` - Constructor for initializing the tax calculator.
- **Corporate Tax Calculation**:
- `public static final double corporaterate = 0.21;` - Sets a constant tax rate of 21%.
- `calculateCorporateIncomeTax(TaxPayer payer)` - Method to compute the corporate tax based on income.
- **Preconditions and Logic**:
- Preconditions specify that the `payer` must not be null and must represent a corporation.
- The method computes tax as a product of the `corporaterate` and `income`.
- If the calculated tax is less than zero, it is reset to zero to prevent negative taxes.
The code snippet highlights a missing variable definition for `income`, indicating a potential area that needs clarification or correction.

Transcribed Image Text:The code snippet provided is a definition of a `TaxPayer` class in Java. This class stores and manages information related to taxpayers, which could be either individuals or businesses.
### Code Breakdown
1. **Attributes:**
- `private String name;` - Holds the name of the person or business.
- `private int age;` - Stores the age of the taxpayer. For businesses, this could be the number of years since establishment.
- `private boolean isCorporation;` - Indicates whether the taxpayer is a business (`true`) or an individual (`false`).
- `private double income;` - Stores the annual income of the taxpayer.
2. **Constructor:**
- `public TaxPayer(String name, int age, double income, boolean isCorporation)`:
- Initializes a new instance of the `TaxPayer` class.
- Preconditions ensure that the `name` is not null or empty, and the `age` is non-negative.
- Sets the class attributes based on the passed parameters.
3. **Methods:**
- `public String getName()`:
- Returns the name of the taxpayer.
- `public int getAge()`:
- Returns the age of the taxpayer.
- `public double getIncome()`:
- Returns the annual income of the taxpayer.
Each method includes JavaDoc style comments documenting its purpose and return value. The class design encapsulates taxpayer information efficiently, providing clear access to each attribute through getter methods.
This structure is part of a typical object-oriented programming design, promoting modularity and reusability.
Expert Solution

Step 1
Access modifiers:
- Private: The data members specified as private are only accessible within the class and not outside it.
- Protected: The data members specified as protected members can be accessed within the class and from the derived class.
- Public: The data members specified as public are accessible everywhere in the program.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
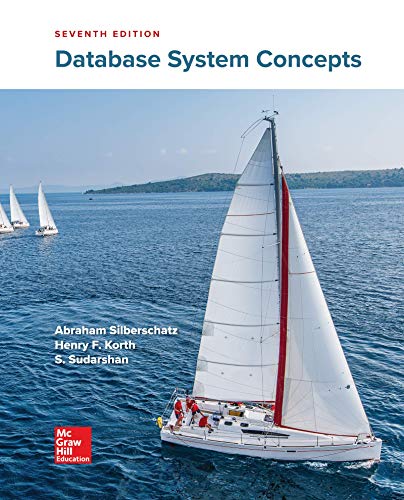
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
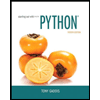
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
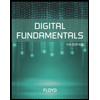
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
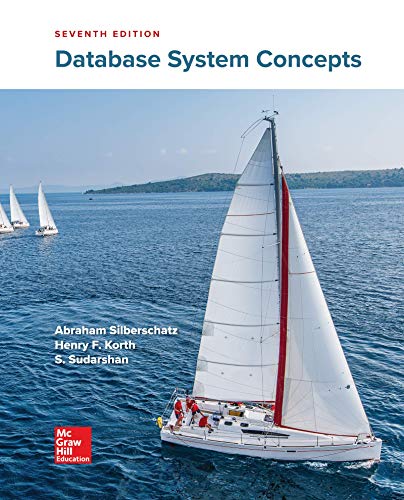
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
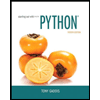
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
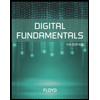
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
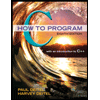
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
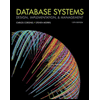
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
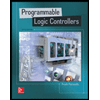
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education