Predict the output? #include using namespace std; class ClassA { public: ClassA(int ii = 0) : i(ii) {} void show() { cout << "i = " << i << endl;} private: int i; }; class ClassB { public: ClassB(int xx) : x(xx) {} operator ClassA() const { return ClassA(x); } private: int x; }; void g(ClassA a) { a.show(); } int main() { ClassB b(10); g(b); g(20); getchar(); return 0; }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
3. Predict the output?
#include<iostream>
using namespace std;
class ClassA {
public:
ClassA(int ii = 0) : i(ii) {}
void show() { cout << "i = " << i << endl;}
private:
int i;
};
class ClassB {
public:
ClassB(int xx) : x(xx) {}
operator ClassA() const { return ClassA(x); }
private:
int x;
};
void g(ClassA a)
{ a.show(); }
int main() {
ClassB b(10);
g(b);
g(20);
getchar();
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

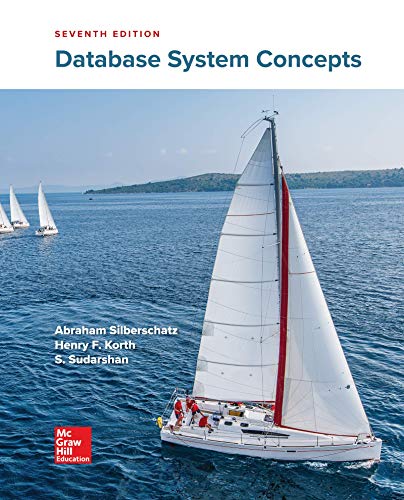
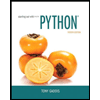
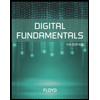
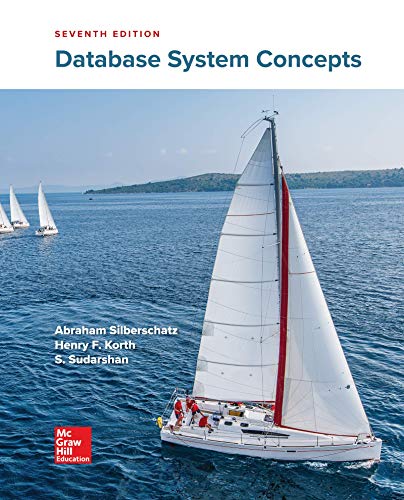
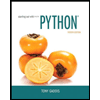
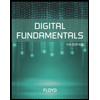
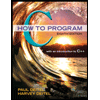
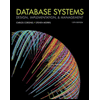
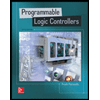