Please write "get" and "set" methods for the other 3 class fields of the SimplePerson class, for example getAge() and setAge() package version; public class SimplePerson { // These are parameters or class fields private String firstName; private String lastName; private String nationality; private int age; //This is a special type of method called a class constructor public SimplePerson(String firstNam, String lastNam, String nation, int howOld) { firstName = firstNam; lastName = lastNam; nationality = nation; age = howOld; } public String getFirstName() { // accessor method return firstName; } public void setFirstName(String newFirstName) { // mutator method firstName = newFirstName; } // Homework Task: Create set and get (accessor and mutator) methods //for all class fields } ---------------------------------------- package version; public class SimplePersonApp { public static void main(String[] args) { // Declaration and Initialization of Person objects SimplePerson wahedRajal = new SimplePerson("Ahmed", "Beloushi", "Emirati", 25); System.out.println("The person's first name is " + wahedRajal.getFirstName()); //Let us change the first name of this SimplePerson object wahedRajal.setFirstName("Yousuf"); System.out.println("The person's first name is changed to " + wahedRajal.getFirstName()); } }
Please write "get" and "set" methods for the other 3 class fields of the SimplePerson class, for example getAge() and setAge()
package version;
public class SimplePerson {
// These are parameters or class fields
private String firstName;
private String lastName;
private String nationality;
private int age;
//This is a special type of method called a class constructor
public SimplePerson(String firstNam, String lastNam, String nation, int howOld)
{
firstName = firstNam;
lastName = lastNam;
nationality = nation;
age = howOld;
}
public String getFirstName() { // accessor method
return firstName;
}
public void setFirstName(String newFirstName) { // mutator method
firstName = newFirstName;
}
// Homework Task: Create set and get (accessor and mutator) methods
//for all class fields
}
----------------------------------------
package version;
public class SimplePersonApp {
public static void main(String[] args) {
// Declaration and Initialization of Person objects
SimplePerson wahedRajal = new SimplePerson("Ahmed", "Beloushi", "Emirati", 25);
System.out.println("The person's first name is " + wahedRajal.getFirstName());
//Let us change the first name of this SimplePerson object
wahedRajal.setFirstName("Yousuf");
System.out.println("The person's first name is changed to "
+ wahedRajal.getFirstName());
}
}

Step by step
Solved in 3 steps

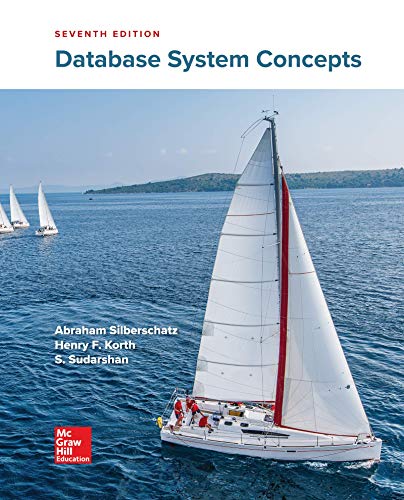
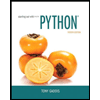
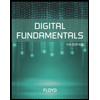
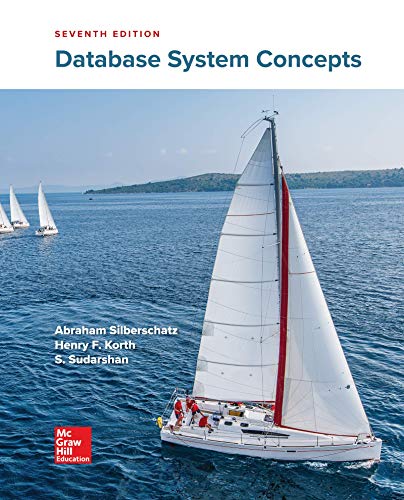
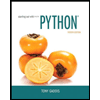
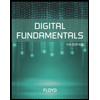
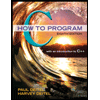
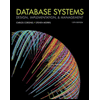
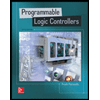