Please help me fix the errors in this java. It keeps showing hello world import java.util.*; class Food // This is the food class { double price; int fat,carbs,fiber; // Declairing varables Food(double price , int fat , int carbs , int fiber) { this.price = price; this.fat = fat; this.carbs = carbs; this.fiber = fiber; } public double getPrice() // Getting price using getter { return price; } public int getFat() // Getting Fat using getter { return fat; } public int getCarbs() // Getting Carbs using getter { return carbs; } public int getFiber() // Getting Fiber using getter { return fiber; } } class LunchOrder { public static void main(String args[]) //main method { Scanner s = new Scanner(System.in); //Taking user Input System.out.print("Enter the number of hamburgers: "); //Asking Enter number of hamburgers: int ham = s.nextInt(); //creating object of food class Food f = new Food(1.85,9,33,1); System.out.println("Each hamburger has "+f.getFat()+"g of fat, "+f.getCarbs()+"g of carbs, "+"and "+f.getFiber()+"g of fiber."); System.out.println(); System.out.print("Enter the number of salads: "); int sal = s.nextInt(); //creating object of food class Food f1 = new Food(2.00,1,11,5); System.out.println("Each salad has "+f1.getFat()+"g of fat, "+f1.getCarbs()+"g of carbs, "+"and "+f1.getFiber()+"g of fiber."); System.out.println(); System.out.print("Enter the number of French Fries: "); int fre = s.nextInt(); //creating object of food class Food f2 = new Food(1.30,11,36,4); System.out.println("French Fries has "+f2.getFat()+"g of fat, "+f2.getCarbs()+"g of carbs, "+"and "+f2.getFiber()+"g of fiber."); System.out.println(); System.out.print("Enter the number of sodas: "); int sod = s.nextInt(); //creating object of food class Food f3 = new Food(0.95,0,38,0); System.out.println("Each soda has "+f3.getFat()+"g of fat, "+f3.getCarbs()+"g of carbs, "+"and "+f3.getFiber()+"g of fiber."); System.out.println(); float total = (float)((ham*f.getPrice()) + (sal*f1.getPrice()) + (fre*f2.getPrice()) + (sod*f3.getPrice())) ; System.out.println("Your order comes to: $"+total); } }
Please help me fix the errors in this java. It keeps showing hello world import java.util.*; class Food // This is the food class { double price; int fat,carbs,fiber; // Declairing varables Food(double price , int fat , int carbs , int fiber) { this.price = price; this.fat = fat; this.carbs = carbs; this.fiber = fiber; } public double getPrice() // Getting price using getter { return price; } public int getFat() // Getting Fat using getter { return fat; } public int getCarbs() // Getting Carbs using getter { return carbs; } public int getFiber() // Getting Fiber using getter { return fiber; } } class LunchOrder { public static void main(String args[]) //main method { Scanner s = new Scanner(System.in); //Taking user Input System.out.print("Enter the number of hamburgers: "); //Asking Enter number of hamburgers: int ham = s.nextInt(); //creating object of food class Food f = new Food(1.85,9,33,1); System.out.println("Each hamburger has "+f.getFat()+"g of fat, "+f.getCarbs()+"g of carbs, "+"and "+f.getFiber()+"g of fiber."); System.out.println(); System.out.print("Enter the number of salads: "); int sal = s.nextInt(); //creating object of food class Food f1 = new Food(2.00,1,11,5); System.out.println("Each salad has "+f1.getFat()+"g of fat, "+f1.getCarbs()+"g of carbs, "+"and "+f1.getFiber()+"g of fiber."); System.out.println(); System.out.print("Enter the number of French Fries: "); int fre = s.nextInt(); //creating object of food class Food f2 = new Food(1.30,11,36,4); System.out.println("French Fries has "+f2.getFat()+"g of fat, "+f2.getCarbs()+"g of carbs, "+"and "+f2.getFiber()+"g of fiber."); System.out.println(); System.out.print("Enter the number of sodas: "); int sod = s.nextInt(); //creating object of food class Food f3 = new Food(0.95,0,38,0); System.out.println("Each soda has "+f3.getFat()+"g of fat, "+f3.getCarbs()+"g of carbs, "+"and "+f3.getFiber()+"g of fiber."); System.out.println(); float total = (float)((ham*f.getPrice()) + (sal*f1.getPrice()) + (fre*f2.getPrice()) + (sod*f3.getPrice())) ; System.out.println("Your order comes to: $"+total); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help me fix the errors in this java. It keeps showing hello world
import java.util.*;
class Food // This is the food class
{
double price;
int fat,carbs,fiber; // Declairing varables
Food(double price , int fat , int carbs , int fiber)
{
this.price = price;
this.fat = fat;
this.carbs = carbs;
this.fiber = fiber;
}
public double getPrice() // Getting price using getter
{
return price;
}
public int getFat() // Getting Fat using getter
{
return fat;
}
public int getCarbs() // Getting Carbs using getter
{
return carbs;
}
public int getFiber() // Getting Fiber using getter
{
return fiber;
}
}
class LunchOrder
{
public static void main(String args[]) //main method
{
Scanner s = new Scanner(System.in); //Taking user Input
System.out.print("Enter the number of hamburgers: "); //Asking Enter number of hamburgers:
int ham = s.nextInt();
//creating object of food class
Food f = new Food(1.85,9,33,1);
System.out.println("Each hamburger has "+f.getFat()+"g of fat, "+f.getCarbs()+"g of carbs, "+"and "+f.getFiber()+"g of fiber.");
System.out.println();
System.out.print("Enter the number of salads: ");
int sal = s.nextInt();
//creating object of food class
Food f1 = new Food(2.00,1,11,5);
System.out.println("Each salad has "+f1.getFat()+"g of fat, "+f1.getCarbs()+"g of carbs, "+"and "+f1.getFiber()+"g of fiber.");
System.out.println();
System.out.print("Enter the number of French Fries: ");
int fre = s.nextInt();
//creating object of food class
Food f2 = new Food(1.30,11,36,4);
System.out.println("French Fries has "+f2.getFat()+"g of fat, "+f2.getCarbs()+"g of carbs, "+"and "+f2.getFiber()+"g of fiber.");
System.out.println();
System.out.print("Enter the number of sodas: ");
int sod = s.nextInt();
//creating object of food class
Food f3 = new Food(0.95,0,38,0);
System.out.println("Each soda has "+f3.getFat()+"g of fat, "+f3.getCarbs()+"g of carbs, "+"and "+f3.getFiber()+"g of fiber.");
System.out.println();
float total = (float)((ham*f.getPrice()) + (sal*f1.getPrice()) + (fre*f2.getPrice()) + (sod*f3.getPrice())) ;
System.out.println("Your order comes to: $"+total);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
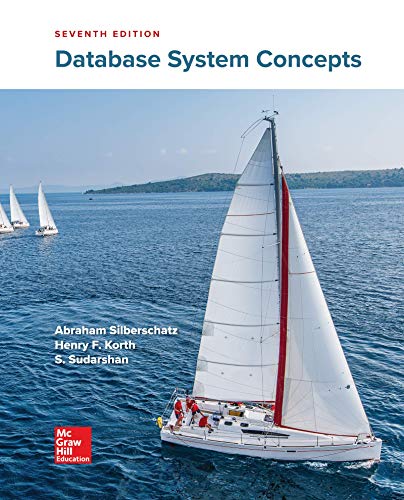
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
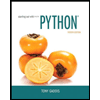
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
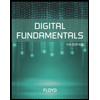
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
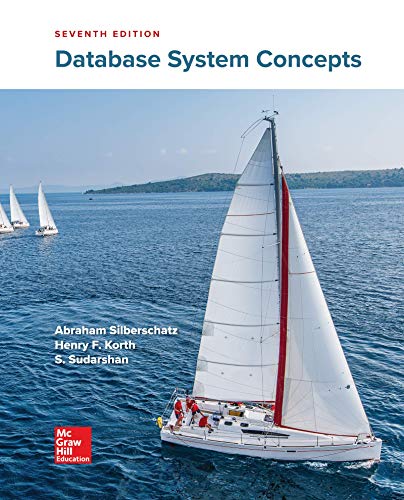
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
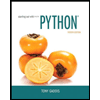
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
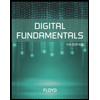
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
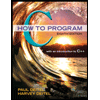
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
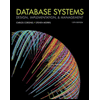
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
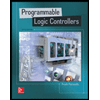
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education