Please enter the first number: 128 Please enter the second number: 100 Result: 102 105 188 111 114 117 120 123 126 Question three Write a program that receives a string from the user and prints the longest word in that string and its length. If there are multiple words with the same maximum length you must print only the first one. Enter the string: I would like to fly to the moon Result: would 5 Another example: Enter the string:
Please enter the first number: 128 Please enter the second number: 100 Result: 102 105 188 111 114 117 120 123 126 Question three Write a program that receives a string from the user and prints the longest word in that string and its length. If there are multiple words with the same maximum length you must print only the first one. Enter the string: I would like to fly to the moon Result: would 5 Another example: Enter the string:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Please enter the first number:
128
Please enter the second number:
100
Result: 102 105 188 111 114 117 120 123 126
Question three
Write a program that receives a string from the user and prints the longest word in that string
and its length. If there are multiple words with the same maximum length you must print only
the first one.
Enter the string:
I would like to fly to the moon
Result: would 5
Another example:
Enter the string:
When did you have your dinner?
Result: dinner 6
jote: If there is a punctuation mark at the end of the string it should be ignored. There will not
de any punctuation marks in the beginning or middle of the string.
Question four
Write a function that receives a list as its only parameter. Inside the body of the function remove
all the duplicate items from the list and returns the new list. For this question, you may not use
Python's dictionary (data type).
Call the function with a simple input – we will change this input while grading.
Example:
If we call the function with ["hey", "swim", "day", "night", "swim"] as its input, the returned value
should be ["hey", "swim", "day", "night"). The order of the items inside the returned list does not
matter.
Question five
Write a function that receives a list of strings as its only parameter. Inside the body of the function
find the first palindromic word and return it. If there is no such word, return an empty string.
Note: A string is palindromic if it reads the same forward and backward ("mom", "noon", and
"civic" are palindromic).
Call the function with a simple input – we will change this input while grading.
Example:
If we call the function with ["abc", "hello", "car", "level", "mom"), the returned value should be
"level". Note that "mom" is also a palindrome, but it is not the first one.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9f8b27a7-8bb5-4735-ae7b-1d9f5ff09ff4%2Fbb77463b-b0e6-46a2-b16f-7001457e0694%2Fyaijp0u_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Please enter the first number:
128
Please enter the second number:
100
Result: 102 105 188 111 114 117 120 123 126
Question three
Write a program that receives a string from the user and prints the longest word in that string
and its length. If there are multiple words with the same maximum length you must print only
the first one.
Enter the string:
I would like to fly to the moon
Result: would 5
Another example:
Enter the string:
When did you have your dinner?
Result: dinner 6
jote: If there is a punctuation mark at the end of the string it should be ignored. There will not
de any punctuation marks in the beginning or middle of the string.
Question four
Write a function that receives a list as its only parameter. Inside the body of the function remove
all the duplicate items from the list and returns the new list. For this question, you may not use
Python's dictionary (data type).
Call the function with a simple input – we will change this input while grading.
Example:
If we call the function with ["hey", "swim", "day", "night", "swim"] as its input, the returned value
should be ["hey", "swim", "day", "night"). The order of the items inside the returned list does not
matter.
Question five
Write a function that receives a list of strings as its only parameter. Inside the body of the function
find the first palindromic word and return it. If there is no such word, return an empty string.
Note: A string is palindromic if it reads the same forward and backward ("mom", "noon", and
"civic" are palindromic).
Call the function with a simple input – we will change this input while grading.
Example:
If we call the function with ["abc", "hello", "car", "level", "mom"), the returned value should be
"level". Note that "mom" is also a palindrome, but it is not the first one.

Transcribed Image Text:Programming Exercises
As mentioned before, you will be answering all the following questions in one single python file
and the person running your file will be prompted to choose which one of these programming
exercises they wish to run.
A good approach to design this file is to have a main function for each of the questions (main1,
main2, etc.). These functions will be called when the user chooses each of the questions (if the
user chooses 1, mainl will be executed and so on). You can call different functions in each of your
mains if you wish.
Below are the programming exercises. The questions are in all levels, some of them are very easy,
and some are more challenging. Start with the questions that are easier for you and then try to
answer as many questions as possible. For grading, we will be looking at the codes, so even if you
have not completely answered a question, make sure you still include your code in the submitted
file as you may receive a partial grade for it.
Note: all user inputs should be entered in a new line after the prompted message.
Question one
Write a program that receives a sequence of numbers from user and prints the number of
appearances for each digit in the sequence. Below is an example of what the program should
look like, Please note that the numbers that have not appeared in the sequence (such as 9) are
not printed. For this question, you may not use Python's dictionary (data type) or the counter
function.
Please enter your sequence:
318047170471
In this sequence we saw:
"g": 2 time(s)
"1": 3 time(s)
"3": 1 time(s)
"A": 2 time(s)
"7": 3 time(s)
"g": 1 time(s)
Question two
Write a program that receives two numbers from the user and prints all the numbers that are
multiplications of three (0, 3, 6, 90, etc.) which are within these two numbers (not inclusive). The
user can enter these two numbers in any order. Here are some examples of the output for such
program.
Please enter the first number:
-4
Please enter the second number:
10
Result: -3@369
Another example:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
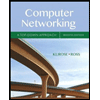
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
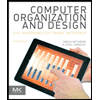
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
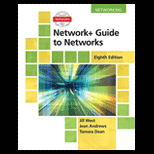
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
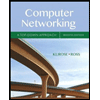
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
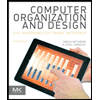
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
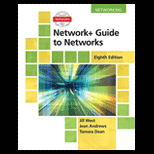
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
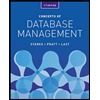
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
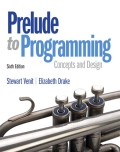
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
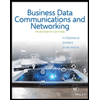
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY