(1) Prompt the user for a string that contains two strings separated by a comma. Examples of strings that can be accepted: Jill, Allen Jill , Allen Jill,Allen Ex: Enter input string: Jill, Allen (2) Report an error if the input string does not contain a comma. Continue to prompt until a valid string is entered. Note: If the input contains a comma, then assume that the input also contains two strings. Ex: Enter input string: Jill Allen Error: No comma in string. Enter input string: Jill, Allen (3) Extract the two words from the input string and remove any spaces. Store the strings in two separate variables and output the strings. Ex: Enter input string: Jill, Allen First word: Jill Second word: Allen (4) Using a loop, extend the program to handle multiple lines of input. Continue until the user enters q to quit. Ex: Enter input string: Jill, Allen First word: Jill Second word: Allen Enter input string: Golden , Monkey First word: Golden Second word: Monkey Enter input string: Washington,DC First word: Washington Second word: DC Enter input string: q
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
(1) Prompt the user for a string that contains two strings separated by a comma.
- Examples of strings that can be accepted:
- Jill, Allen
- Jill , Allen
- Jill,Allen
Ex:
Enter input string: Jill, Allen
(2) Report an error if the input string does not contain a comma. Continue to prompt until a valid string is entered. Note: If the input contains a comma, then assume that the input also contains two strings.
Ex:
Enter input string: Jill Allen Error: No comma in string. Enter input string: Jill, Allen
(3) Extract the two words from the input string and remove any spaces. Store the strings in two separate variables and output the strings.
Ex:
Enter input string: Jill, Allen First word: Jill Second word: Allen
(4) Using a loop, extend the program to handle multiple lines of input. Continue until the user enters q to quit.
Ex:
Enter input string: Jill, Allen First word: Jill Second word: Allen Enter input string: Golden , Monkey First word: Golden Second word: Monkey Enter input string: Washington,DC First word: Washington Second word: DC Enter input string: q
![**Lab Activity: Warm up - Parsing Strings**
**File: ParseStrings.java**
```java
import java.util.Scanner;
public class ParseStrings {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
/* Type your code here. */
}
}
```
**Description:**
This Java program sets up the basic structure to parse strings using the `Scanner` class. The `Scanner` is a utility provided in the `java.util` package, which allows the reading of input from various sources, including user input from the console.
- **Import Statement:**
`import java.util.Scanner;`
This line imports the `Scanner` class, which is necessary to read user input in Java.
- **Class Declaration:**
`public class ParseStrings { ... }`
This declares the class named `ParseStrings`. All the logic for parsing strings will reside within this class.
- **Main Method:**
`public static void main(String[] args) { ... }`
This is the entry point for any Java application. The `main` method runs the code necessary for the program's execution.
- **Scanner Initialization:**
`Scanner scnr = new Scanner(System.in);`
This line initializes a new `Scanner` object named `scnr` to read input from the standard input stream, typically the keyboard (System.in).
- **Code Placeholder:**
`/* Type your code here. */`
A comment indicating where the user should write additional code to perform the desired task, such as parsing input strings.
This example sets up the framework necessary to process user input and perform operations on strings as the lab activity progresses.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9e6fa127-a003-469d-9a51-b1d4610918ed%2F960a3adc-5204-4b16-8885-fab8f227e41e%2F8u12mhb_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

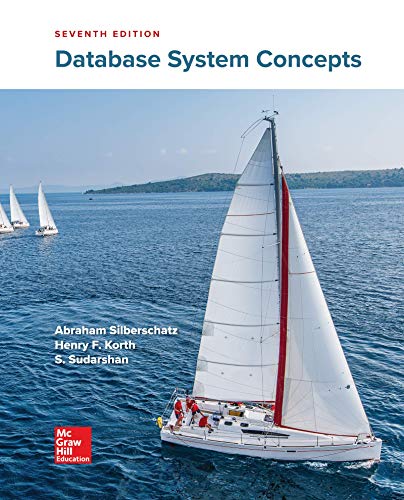
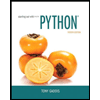
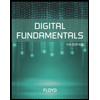
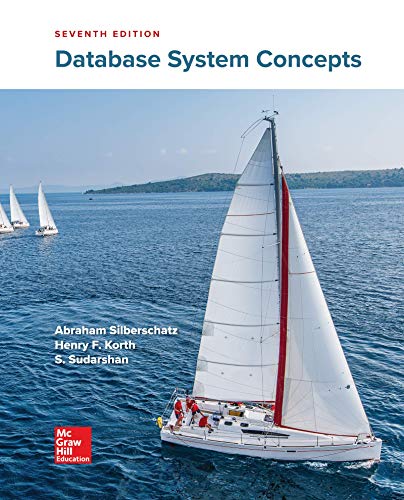
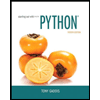
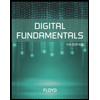
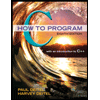
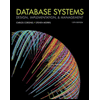
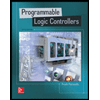