Please create a short Pac-Man game using gui. Do not use the one on the internet as I am a beginner. Please add a button to start, and a button where user can exit. Please also had and instructions manual into the code
Please create a short Pac-Man game using gui. Do not use the one on the internet as I am a beginner. Please add a button to start, and a button where user can exit. Please also had and instructions manual into the code
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help me with this using java

Transcribed Image Text:Please create a short Pac-Man game using
gui. Do not use the one on the internet as I
am a beginner. Please add a button to start,
and a button where user can exit. Please also
had and instructions manual into the code
where the user can learn how to play the
game
Expert Solution

Step 1
package com.zetcode; import java.awt.BasicStroke; import java.awt.Color; import java.awt.Dimension; import java.awt.Event; import java.awt.Font; import java.awt.FontMetrics; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Image; import java.awt.Toolkit; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyAdapter; import java.awt.event.KeyEvent; import javax.swing.ImageIcon; import javax.swing.JPanel; import javax.swing.Timer; public class Board extends JPanel implements ActionListener { private Dimension d; private final Font smallfont = new Font("Helvetica", Font.BOLD, 14); private Image ii; private final Color dotcolor = new Color(192, 192, 0); private Color mazecolor; private boolean ingame = false; private boolean dying = false; private final int blocksize = 24; private final int nrofblocks = 15; private final int scrsize = nrofblocks * blocksize; private final int pacanimdelay = 2; private final int pacmananimcount = 4; private final int maxghosts = 12; private final int pacmanspeed = 6; private int pacanimcount = pacanimdelay; private int pacanimdir = 1; private int pacmananimpos = 0; private int nrofghosts = 6; private int pacsleft, score; private int[] dx, dy; private int[] ghostx, ghosty, ghostdx, ghostdy, ghostspeed; private Image ghost; private Image pacman1, pacman2up, pacman2left, pacman2right, pacman2down; private Image pacman3up, pacman3down, pacman3left, pacman3right; private Image pacman4up, pacman4down, pacman4left, pacman4right; private int pacmanx, pacmany, pacmandx, pacmandy; private int reqdx, reqdy, viewdx, viewdy; private final short leveldata[] = { 19, 26, 26, 26, 18, 18, 18, 18, 18, 18, 18, 18, 18, 18, 22, 21, 0, 0, 0, 17, 16, 16, 16, 16, 16, 16, 16, 16, 16, 20, 21, 0, 0, 0, 17, 16, 16, 16, 16, 16, 16, 16, 16, 16, 20, 21, 0, 0, 0, 17, 16, 16, 24, 16, 16, 16, 16, 16, 16, 20, 17, 18, 18, 18, 16, 16, 20, 0, 17, 16, 16, 16, 16, 16, 20, 17, 16, 16, 16, 16, 16, 20, 0, 17, 16, 16, 16, 16, 24, 20, 25, 16, 16, 16, 24, 24, 28, 0, 25, 24, 24, 16, 20, 0, 21, 1, 17, 16, 20, 0, 0, 0, 0, 0, 0, 0, 17, 20, 0, 21, 1, 17, 16, 16, 18, 18, 22, 0, 19, 18, 18, 16, 20, 0, 21, 1, 17, 16, 16, 16, 16, 20, 0, 17, 16, 16, 16, 20, 0, 21, 1, 17, 16, 16, 16, 16, 20, 0, 17, 16, 16, 16, 20, 0, 21, 1, 17, 16, 16, 16, 16, 16, 18, 16, 16, 16, 16, 20, 0, 21, 1, 17, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 20, 0, 21, 1, 25, 24, 24, 24, 24, 24, 24, 24, 24, 16, 16, 16, 18, 20, 9, 8, 8, 8, 8, 8, 8, 8, 8, 8, 25, 24, 24, 24, 28 }; private final int validspeeds[] = {1, 2, 3, 4, 6, 8}; private final int maxspeed = 6; private int currentspeed = 3; private short[] screendata; private Timer timer; public Board() { loadImages(); initVariables(); addKeyListener(new TAdapter()); setFocusable(true); setBackground(Color.black); setDoubleBuffered(true); } private void initVariables() { screendata = new short[nrofblocks * nrofblocks]; mazecolor = new Color(5, 100, 5); d = new Dimension(400, 400); ghostx = new int[maxghosts]; ghostdx = new int[maxghosts]; ghosty = new int[maxghosts]; ghostdy = new int[maxghosts]; ghostspeed = new int[maxghosts]; dx = new int[4]; dy = new int[4]; timer = new Timer(40, this); timer.start(); } @Override public void addNotify() { super.addNotify(); initGame(); } private void doAnim() { pacanimcount--; if (pacanimcount <= 0) { pacanimcount = pacanimdelay; pacmananimpos = pacmananimpos + pacanimdir; if (pacmananimpos == (pacmananimcount - 1) || pacmananimpos == 0) { pacanimdir = -pacanimdir; } } } private void playGame(Graphics2D g2d) { if (dying) { death(); } else { movePacman(); drawPacman(g2d); moveGhosts(g2d); checkMaze(); } } private void showIntroScreen(Graphics2D g2d) { g2d.setColor(new Color(0, 32, 48)); g2d.fillRect(50, scrsize / 2 - 30, scrsize - 100, 50); g2d.setColor(Color.white); g2d.drawRect(50, scrsize / 2 - 30, scrsize - 100, 50); String s = "Press s to start."; Font small = new Font("Helvetica", Font.BOLD, 14); FontMetrics metr = this.getFontMetrics(small); g2d.setColor(Color.white); g2d.setFont(small); g2d.drawString(s, (scrsize - metr.stringWidth(s)) / 2, scrsize / 2); } private void drawScore(Graphics2D g) { int i; String s; g.setFont(smallfont); g.setColor(new Color(96, 128, 255)); s = "Score: " + score; g.drawString(s, scrsize / 2 + 96, scrsize + 16); for (i = 0; i < pacsleft; i++) { g.drawImage(pacman3left, i * 28 + 8, scrsize + 1, this); } } private void checkMaze() { short i = 0; boolean finished = true; while (i < nrofblocks * nrofblocks && finished) { if ((screendata[i] & 48) != 0) { finished = false; } i++; } if (finished) { score += 50; if (nrofghosts < maxghosts) { nrofghosts++; } if (currentspeed < maxspeed) { currentspeed++; } initLevel(); } } private void death() { pacsleft--; if (pacsleft == 0) { ingame = false; } continueLevel(); } private void moveGhosts(Graphics2D g2d) { short i; int pos; int count; for (i = 0; i < nrofghosts; i++) { if (ghostx[i] % blocksize == 0 && ghosty[i] % blocksize == 0) { pos = ghostx[i] / blocksize + nrofblocks * (int) (ghosty[i] / blocksize); count = 0; if ((screendata[pos] & 1) == 0 && ghostdx[i] != 1) { dx[count] = -1; dy[count] = 0; count++; } if ((screendata[pos] & 2) == 0 && ghostdy[i] != 1) { dx[count] = 0; dy[count] = -1; count++; } if ((screendata[pos] & 4) == 0 && ghostdx[i] != -1) { dx[count] = 1; dy[count] = 0; count++; } if ((screendata[pos] & 8) == 0 && ghostdy[i] != -1) { dx[count] = 0; dy[count] = 1; count++; } if (count == 0) { if ((screendata[pos] & 15) == 15) { ghostdx[i] = 0; ghostdy[i] = 0; } else { ghostdx[i] = -ghostdx[i]; ghostdy[i] = -ghostdy[i]; } } else { count = (int) (Math.random() * count); if (count > 3) { count = 3; } ghostdx[i] = dx[count]; ghostdy[i] = dy[count]; } } ghostx[i] = ghostx[i] + (ghostdx[i] * ghostspeed[i]); ghosty[i] = ghosty[i] + (ghostdy[i] * ghostspeed[i]); drawGhost(g2d, ghostx[i] + 1, ghosty[i] + 1); if (pacmanx > (ghostx[i] - 12) && pacmanx < (ghostx[i] + 12) && pacmany > (ghosty[i] - 12) && pacmany < (ghosty[i] + 12) && ingame) { dying = true; } } } private void drawGhost(Graphics2D g2d, int x, int y) { g2d.drawImage(ghost, x, y, this); } private void movePacman() { int pos; short ch; if (reqdx == -pacmandx && reqdy == -pacmandy) { pacmandx = reqdx; pacmandy = reqdy; viewdx = pacmandx; viewdy = pacmandy; } if (pacmanx % blocksize == 0 && pacmany % blocksize == 0) { pos = pacmanx / blocksize + nrofblocks * (int) (pacmany / blocksize); ch = screendata[pos]; if ((ch & 16) != 0) { screendata[pos] = (short) (ch & 15); score++; } if (reqdx != 0 || reqdy != 0) { if (!((reqdx == -1 && reqdy == 0 && (ch & 1) != 0) || (reqdx == 1 && reqdy == 0 && (ch & 4) != 0) || (reqdx == 0 && reqdy == -1 && (ch & 2) != 0) || (reqdx == 0 && reqdy == 1 && (ch & 8) != 0))) { pacmandx = reqdx; pacmandy = reqdy; viewdx = pacmandx; viewdy = pacmandy; } } // Check for standstill if ((pacmandx == -1 && pacmandy == 0 && (ch & 1) != 0) || (pacmandx == 1 && pacmandy == 0 && (ch & 4) != 0) || (pacmandx == 0 && pacmandy == -1 && (ch & 2) != 0) || (pacmandx == 0 && pacmandy == 1 && (ch & 8) != 0)) { pacmandx = 0; pacmandy = 0; } } pacmanx = pacmanx + pacmanspeed * pacmandx; pacmany = pacmany + pacmanspeed * pacmandy; } private void drawPacman(Graphics2D g2d) { if (viewdx == -1) { drawPacnanLeft(g2d); } else if (viewdx == 1) { drawPacmanRight(g2d); } else if (viewdy == -1) { drawPacmanUp(g2d); } else { drawPacmanDown(g2d); } } private void drawPacmanUp(Graphics2D g2d) { switch (pacmananimpos) { case 1: g2d.drawImage(pacman2up, pacmanx + 1, pacmany + 1, this); break; case 2: g2d.drawImage(pacman3up, pacmanx + 1, pacmany + 1, this); break; case 3: g2d.drawImage(pacman4up, pacmanx + 1, pacmany + 1, this); break; default: g2d.drawImage(pacman1, pacmanx + 1, pacmany + 1, this); break; } } private void drawPacmanDown(Graphics2D g2d) { switch (pacmananimpos) { case 1: g2d.drawImage(pacman2down, pacmanx + 1, pacmany + 1, this); break; case 2: g2d.drawImage(pacman3down, pacmanx + 1, pacmany + 1, this); break; case 3: g2d.drawImage(pacman4down, pacmanx + 1, pacmany + 1, this); break; default: g2d.drawImage(pacman1, pacmanx + 1, pacmany + 1, this); break; } } private void drawPacnanLeft(Graphics2D g2d) { switch (pacmananimpos) { case 1: g2d.drawImage(pacman2left, pacmanx + 1, pacmany + 1, this); break; case 2: g2d.drawImage(pacman3left, pacmanx + 1, pacmany + 1, this); break; case 3: g2d.drawImage(pacman4left, pacmanx + 1, pacmany + 1, this); break; default: g2d.drawImage(pacman1, pacmanx + 1, pacmany + 1, this); break; } } private void drawPacmanRight(Graphics2D g2d) { switch (pacmananimpos) { case 1: g2d.drawImage(pacman2right, pacmanx + 1, pacmany + 1, this); break; case 2: g2d.drawImage(pacman3right, pacmanx + 1, pacmany + 1, this); break; case 3: g2d.drawImage(pacman4right, pacmanx + 1, pacmany + 1, this); break; default: g2d.drawImage(pacman1, pacmanx + 1, pacmany + 1, this); break; } } private void drawMaze(Graphics2D g2d) { short i = 0; int x, y; for (y = 0; y < scrsize; y += blocksize) { for (x = 0; x < scrsize; x += blocksize) { g2d.setColor(mazecolor); g2d.setStroke(new BasicStroke(2)); if ((screendata[i] & 1) != 0) { g2d.drawLine(x, y, x, y + blocksize - 1); } if ((screendata[i] & 2) != 0) { g2d.drawLine(x, y, x + blocksize - 1, y); } if ((screendata[i] & 4) != 0) { g2d.drawLine(x + blocksize - 1, y, x + blocksize - 1, y + blocksize - 1); } if ((screendata[i] & 8) != 0) { g2d.drawLine(x, y + blocksize - 1, x + blocksize - 1, y + blocksize - 1); } if ((screendata[i] & 16) != 0) { g2d.setColor(dotcolor); g2d.fillRect(x + 11, y + 11, 2, 2); } i++; } } } private void initGame() { pacsleft = 3; score = 0; initLevel(); nrofghosts = 6; currentspeed = 3; } private void initLevel() { int i; for (i = 0; i < nrofblocks * nrofblocks; i++) { screendata[i] = leveldata[i]; } continueLevel(); } private void continueLevel() { short i; int dx = 1; int random; for (i = 0; i < nrofghosts; i++) { ghosty[i] = 4 * blocksize; ghostx[i] = 4 * blocksize; ghostdy[i] = 0; ghostdx[i] = dx; dx = -dx; random = (int) (Math.random() * (currentspeed + 1)); if (random > currentspeed) { random = currentspeed; } ghostspeed[i] = validspeeds[random]; } pacmanx = 7 * blocksize; pacmany = 11 * blocksize; pacmandx = 0; pacmandy = 0; reqdx = 0; reqdy = 0; viewdx = -1; viewdy = 0; dying = false; } private void loadImages() { ghost = new ImageIcon("images/ghost.png").getImage(); pacman1 = new ImageIcon("images/pacman.png").getImage(); pacman2up = new ImageIcon("images/up1.png").getImage(); pacman3up = new ImageIcon("images/up2.png").getImage(); pacman4up = new ImageIcon("images/up3.png").getImage(); pacman2down = new ImageIcon("images/down1.png").getImage(); pacman3down = new ImageIcon("images/down2.png").getImage(); pacman4down = new ImageIcon("images/down3.png").getImage(); pacman2left = new ImageIcon("images/left1.png").getImage(); pacman3left = new ImageIcon("images/left2.png").getImage(); pacman4left = new ImageIcon("images/left3.png").getImage(); pacman2right = new ImageIcon("images/right1.png").getImage(); pacman3right = new ImageIcon("images/right2.png").getImage(); pacman4right = new ImageIcon("images/right3.png").getImage(); } @Override public void paintComponent(Graphics g) { super.paintComponent(g); doDrawing(g); } private void doDrawing(Graphics g) { Graphics2D g2d = (Graphics2D) g; g2d.setColor(Color.black); g2d.fillRect(0, 0, d.width, d.height); drawMaze(g2d); drawScore(g2d); doAnim(); if (ingame) { playGame(g2d); } else { showIntroScreen(g2d); } g2d.drawImage(ii, 5, 5, this); Toolkit.getDefaultToolkit().sync(); g2d.dispose(); } class TAdapter extends KeyAdapter { @Override public void keyPressed(KeyEvent e) { int key = e.getKeyCode(); if (ingame) { if (key == KeyEvent.VK_LEFT) { reqdx = -1; reqdy = 0; } else if (key == KeyEvent.VK_RIGHT) { reqdx = 1; reqdy = 0; } else if (key == KeyEvent.VK_UP) { reqdx = 0; reqdy = -1; } else if (key == KeyEvent.VK_DOWN) { reqdx = 0; reqdy = 1; } else if (key == KeyEvent.VK_ESCAPE && timer.isRunning()) { ingame = false; } else if (key == KeyEvent.VK_PAUSE) { if (timer.isRunning()) { timer.stop(); } else { timer.start(); } } } else { if (key == 's' || key == 'S') { ingame = true; initGame(); } } } @Override public void keyReleased(KeyEvent e) { int key = e.getKeyCode(); if (key == Event.LEFT || key == Event.RIGHT || key == Event.UP || key == Event.DOWN) { reqdx = 0; reqdy = 0; } } } @Override public void actionPerformed(ActionEvent e) { repaint(); } }
Was this answer helpful?
0
0
More Answers
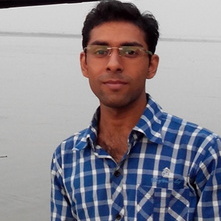
Anonymous answered this123 answers
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ /* * keyPressedClassUI.java * * Created on Sep 29, 2010, 8:05:53 AM */ package pacManPlan; import java.awt.BasicStroke; import java.awt.Color; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Polygon; import java.awt.Rectangle; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.awt.geom.Ellipse2D; /** * * @author Administrator */ public class pacManPlanUI extends javax.swing.JFrame implements KeyListener, Runnable { Graphics draw; int x = 50, y = 110; int start = 40, angle = 280; boolean okMove = false;//thread control flag long delay = 10; //fps int xMove = 0; int yMove = 0; Thread move = null; long time;// = System.currentTimeMillis(); Graphics2D draw2d; Polygon borderLeft = new Polygon(); Polygon borderRight= new Polygon(); Polygon borderbot = new Polygon(); Polygon triangle = new Polygon(); // int xOffset, yOffset; BasicStroke wallStroke = new BasicStroke(10, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND); //fix the tunnelling problem int pressed, clipDir; //dots Ellipse2D dots[] = new Ellipse2D[10]; Ellipse2D.Double ghost = new Ellipse2D.Double(); Rectangle ghostRect = new Rectangle(); Rectangle ghostRect2 = new Rectangle();//draw ghost int gx = 100; int gy = 40; int gxm = -1; //ghost movement int gym = -1; public void setDots() { for (int x = 0; x < dots.length - 1; x++) { dots[x] = new Ellipse2D.Double(); //subclass dots[x].setFrame(x * 30, 40, 10, 10); } } public void setMaze() { //left border borderLeft.addPoint(0, 0); borderLeft.addPoint(0, 600); borderLeft.addPoint(10, 600); borderLeft.addPoint(10, 0); borderLeft.addPoint(0, 0);//back to starting point borderRight.addPoint(600,600); borderRight.addPoint(600,0); borderRight.addPoint(600,10); borderRight.addPoint(600,600); borderbot.addPoint(0, 600); borderbot.addPoint(10, 600); //draw.fillRect(x, y, WIDTH, start); triangle.addPoint(50, 50); triangle.addPoint(25, 100); triangle.addPoint(75, 100); triangle.addPoint(50, 50); } public void clipMaze() { Rectangle pacRec = new Rectangle(x - 5, y - 5, 25, 25); //clipDir is a flag clipDir = -1; if (borderLeft.intersects(pacRec)) { xMove = 0; yMove = 0; clipDir = pressed; //set the direction of travel when clipping } if (triangle.intersects(pacRec)) { xMove = 0; yMove = 0; clipDir = pressed; } //determine if ghost clips //determine which direction to go //random - bad idea //move in an intelligent direction //bounce back if clip switch (clipDir) { case 37: case 65: x -= 2; okMove = false; //break; case 38: y -= 2; okMove = false; // break; case 39: x += 2; okMove = false; // break; case 40: y += 2; okMove = false; } for (int x = 0; x < dots.length - 1; x++) { if (dots[x].intersects(pacRec)) { draw2d.setColor(Color.black); draw2d.fill(dots[x]); dots[x].setFrame(-100, -100, 0, 0); } } } /** Creates new form keyPressedClassUI */ public pacManPlanUI() { initComponents(); draw = this.jPanel1.getGraphics(); addKeyListener(this); move = new Thread(this); move.start(); draw2d = (Graphics2D) draw; ghostRect.setSize(30, 30); ghostRect2.setSize(20, 20); setMaze(); setDots(); } public void paint(Graphics g) { super.paint(g); //kill flicker g.setColor(Color.black); g.fillRect(0, 0, jPanel1.getWidth(), jPanel1.getHeight()); update(draw); //update reduces flicker in animation } public void update(Graphics g) { //erase pac g.setColor(Color.black); g.fillOval(x - 3, y - 3, 25, 25); g.setColor(Color.yellow); g.fillArc(x, y, 20, 20, start, angle); g.setColor(Color.red); draw2d.setStroke(wallStroke); draw2d.drawPolygon(triangle); draw2d.drawPolygon(borderLeft); draw2d.drawPolygon(borderRight); //draw ghost draw2d.setColor(Color.black); draw2d.fillOval(gx-2, gy -2, 35,35); draw2d.setColor(Color.pink); ghostRect.setLocation(gx, gy); ghost.setFrame(ghostRect); draw2d.fill(ghost); //ghost2 draw2d.setColor(Color.black); draw2d.fillOval(gx-2, gy -2, 35,35); draw2d.setColor(Color.green); ghostRect.setLocation(150, 25); ghost.setFrame(ghostRect2); draw2d.fill(ghost); draw2d.setColor(Color.white); //draw dots for (int x = 0; x < dots.length - 1; x++) { draw2d.fill(dots[x]); } // clipMaze(); } /** This method is called from within the constructor to * initialize the form. * WARNING: Do NOT modify this code. The content of this method is * always regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { jPanel1 = new javax.swing.JPanel(); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); jPanel1.setMaximumSize(new java.awt.Dimension(600, 600)); jPanel1.setMinimumSize(new java.awt.Dimension(600, 600)); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGap(0, 808, Short.MAX_VALUE) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGap(0, 600, Short.MAX_VALUE) ); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) ); pack(); }// </editor-fold> /** * @param args the command line arguments */ public static void main(String args[]) { //java.awt.EventQueue.invokeLater(new Runnable() { // public void run() { new pacManPlanUI().setVisible(true); // } //}); } public void keyTyped(KeyEvent e) { int pressed = e.getKeyCode(); String keyP = String.valueOf(pressed); } public void keyPressed(KeyEvent e) { int pressed = e.getKeyCode(); String keyP = String.valueOf(pressed); //this.setTitle(okMove + " "); } public void keyReleased(KeyEvent e) { pressed = e.getKeyCode(); String keyP = String.valueOf(pressed); //okMove = true; // draw.clearRect(0,0,100,100); // draw.drawString(keyP + " pressed", 10, 30); // draw.clearRect(0,0,100,100); // draw.drawString(keyP + " released", 10,50); //37 = left = 100 number pad 4 = 65 A //38 = up //39 = right //40 = down //arrow keys //start the thread // if(move == null) //{ //stop(); //move.stop(); //requires a Runnable frame //requires the run method // } // okMove = false; this.setTitle(pressed + " "); if (pressed == 37 || pressed == 100 || pressed == 65) { //draw.drawString("went left", 10, 70); //x-=2; //redraw the screen start = 220; //repaint(); xMove = -2; yMove = 0; okMove = true; } if (pressed == 39) { start = 40; // x += 2; // repaint(); xMove = 2; yMove = 0; okMove = true; } //up if (pressed == 38) { start = 130; // y -= 2; // repaint(); xMove = 0; yMove = -2; okMove = true; } //down if (pressed == 40) { start = 310; // y += 2; // repaint(); xMove = 0; yMove = 2; okMove = true; } if (pressed == 32) { okMove = false; } if (okMove) { time = System.currentTimeMillis(); //reset when the movement starts } } // Variables declaration - do not modify private javax.swing.JPanel jPanel1; // End of variables declaration public void run() { //throw new UnsupportedOperationException("Not supported yet."); //long time = System.currentTimeMillis(); //get the time the thread is started long sleepInterval = delay; //set to fps //this.setTitle(okMove + "in run"); //int counter = 0; while (true) { if (okMove) { clipMaze(); x += xMove; y += yMove; // this.setTitle("in run"); //calculate the sleep interval time += delay; sleepInterval = Math.max(delay, time - System.currentTimeMillis()); //this.setTitle(sleepInterval + " "); try { move.sleep(sleepInterval * 2); } catch (InterruptedException ex) { //Logger.getLogger(keyPressedClassUI.class.getName()).log(Level.SEVERE, null, ex); } } //chase pacman if(x < gx) { gxm = -1;//move ghost to left } else if(x > gx) { gxm = 1; } else if(x == gx) { gxm = 0; } if(y < gy) { gym = -1;//move ghost to left } else if(y > gy) { gym = 1; } else if(y == gy) { gym = 0; } //move the ghost //clipghost here gx += gxm; gy += gym; try { move.sleep(sleepInterval * 2); } catch (InterruptedException ex) { //Logger.getLogger(keyPressedClassUI.class.getName()).log(Level.SEVERE, null, ex); } update(draw); }//end of while } }
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
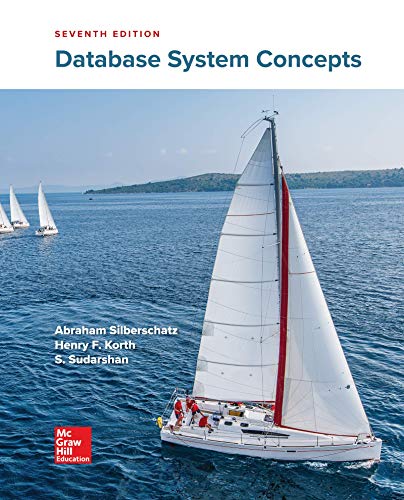
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
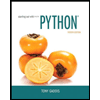
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
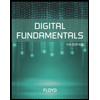
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
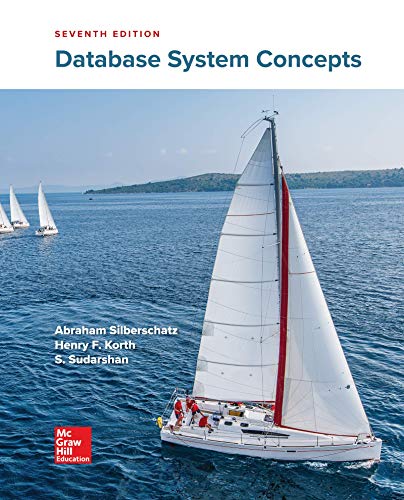
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
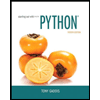
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
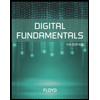
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
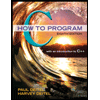
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
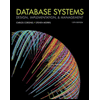
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
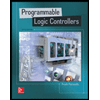
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education