PLEASE CREATE A FLOWCHART FOR THIS PROGRAM: user-defined methods is performed. Sample output is attached. 4 functionalities of an Automated Teller Machine(Withdraw, Deposit, Transfer, Balance Check) import java.util.Scanner; public class ATM { double balance = 0, withdrawn, deposit; static Scanner sc = new Scanner(System.in);// creating scanner object public void Withdraw() {// method withdraw System.out.println("Enter the amount to be withdrawn"); withdrawn = sc.nextDouble();// read the amount to be withdrawn balance = balance - withdrawn;// calculating balance if (balance < withdrawn) {// check sufficient balance in account System.out.println("Insufficient Balance");// if not,print insufficient balance if (balance < 0) { balance = 0; } } else {// otherwise,print collect money. System.out.println("collect your money.."); } System.out.println("Check Balance 1.Yes 2.No");// to check the balance in account int check = sc.nextInt();// if want to check,then read the yes if (check == 1) {// if yes,then print balance System.out.println("Balance := " + balance); } else {// otherwise return to calling function return; } } public void Deposit() {// method deposit System.out.println("Enter amount to be deposited :"); deposit = sc.nextDouble();// read the amount to be deposited balance = balance + deposit;// add the deposited balance to balance System.out.println("Check Balance 1.Yes 2.No"); int check = sc.nextInt();// check whether want to display the balance if (check == 1) {// if 1,then want to check the balance. System.out.println("Balance := " + balance); } else {// otherwise return to calling function return; } } public void Transfer() { System.out.println("Enter account number :"); double account_number = sc.nextDouble();// read the account number System.out.println("Ente amount :"); double moneysent = sc.nextDouble();// read the amount to be transferred balance = balance-moneysent; } public void CheckBalance() { System.out.println("Balance = " + balance);// Display the balance } public static void main(String[] args) { ATM atm = new ATM();// creating object of class. while (true) { System.out.println("--------ATM--------\n1.Withdraw\n2.Deposit\n3.Transfer\n4.check Balance\n5.EXIT");// display the // menu int choice; choice = sc.nextInt();// read the choice switch (choice) { case 1:// if 1,call method withdraw atm.Withdraw(); break; case 2:// if 2,call deposit atm.Deposit(); break; case 3:// if 3,call checkbalance atm.Transfer(); case 4:// if 3,then transfer atm.CheckBalance(); break; case 5: //if 5,then exit from loop. System.exit(0); } } } }
PLEASE CREATE A FLOWCHART FOR THIS PROGRAM:
user-defined methods is performed. Sample output is attached.
4 functionalities of an Automated Teller
Machine(Withdraw, Deposit, Transfer, Balance Check)
import java.util.Scanner;
public class ATM {
double balance = 0, withdrawn, deposit;
static Scanner sc = new Scanner(System.in);// creating scanner object
public void Withdraw() {// method withdraw
System.out.println("Enter the amount to be withdrawn");
withdrawn = sc.nextDouble();// read the amount to be withdrawn
balance = balance - withdrawn;// calculating balance
if (balance < withdrawn) {// check sufficient balance in account
System.out.println("Insufficient Balance");// if not,print insufficient balance
if (balance < 0) {
balance = 0;
}
} else {// otherwise,print collect money.
System.out.println("collect your money..");
}
System.out.println("Check Balance 1.Yes 2.No");// to check the balance in account
int check = sc.nextInt();// if want to check,then read the yes
if (check == 1) {// if yes,then print balance
System.out.println("Balance := " + balance);
} else {// otherwise return to calling function
return;
}
}
public void Deposit() {// method deposit
System.out.println("Enter amount to be deposited :");
deposit = sc.nextDouble();// read the amount to be deposited
balance = balance + deposit;// add the deposited balance to balance
System.out.println("Check Balance 1.Yes 2.No");
int check = sc.nextInt();// check whether want to display the balance
if (check == 1) {// if 1,then want to check the balance.
System.out.println("Balance := " + balance);
} else {// otherwise return to calling function
return;
}
}
public void Transfer()
{
System.out.println("Enter account number :");
double account_number = sc.nextDouble();// read the account number
System.out.println("Ente amount :");
double moneysent = sc.nextDouble();// read the amount to be transferred
balance = balance-moneysent;
}
public void CheckBalance() {
System.out.println("Balance = " + balance);// Display the balance
}
public static void main(String[] args) {
ATM atm = new ATM();// creating object of class.
while (true) {
System.out.println("--------ATM--------\n1.Withdraw\n2.Deposit\n3.Transfer\n4.check Balance\n5.EXIT");// display the
// menu
int choice;
choice = sc.nextInt();// read the choice
switch (choice) {
case 1:// if 1,call method withdraw
atm.Withdraw();
break;
case 2:// if 2,call deposit
atm.Deposit();
break;
case 3:// if 3,call checkbalance
atm.Transfer();
case 4:// if 3,then transfer
atm.CheckBalance();
break;
case 5: //if 5,then exit from loop.
System.exit(0);
}
}
}
}


Step by step
Solved in 2 steps with 1 images

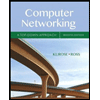
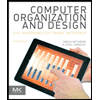
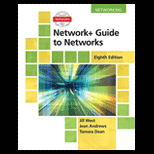
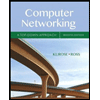
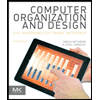
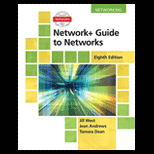
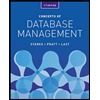
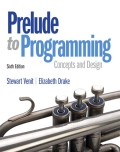
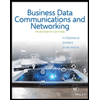