Please correct the answer for column Ea%.For 1st iteration it will display "---" For 2nd iteration, 1.0333-1/1.0333 times 100 = 3.22, For 3rd iteration, 1.0641-1.0333/1.0641 times 100 = 2.89. As observed for Ea the values used are from the previous row. The output table at inputs 1,5,4 is: The code: #include #include #include #include #include using namespace std::chrono; using namespace std; static double function(double x); // function f(x) void menu(); int main() { double a; // Lower Guess or beginning of interval double b; // Upper Guess or end of interval double c = 0; // variable for midpoint double terms; double newc; // Taking Input cout << "Enter Xn-1: "; cin >> a; cout << "\nEnter Xn: "; cin >> b; cout << "\nEnter number of iterations: "; cin >> terms; // Check for opposite sign (Intermediate Value Theorem) if (function(a) * function(b) > 0.0f) { cout << "\nFunction has same signs at ends of interval"; return -1; } int iter = 1; cout << setw(3) << "\n\n N (iterations)" << setw(9) << "Xn-1" << setw(17) << "Xn" << setw(18) << "Xn+1" << " Ea% " << endl; auto start = high_resolution_clock::now(); while (terms >= iter) // terminating condition { double newC = a-(function(a)*(b-a))/(function(b)-function(a)); if (c == 0) { cout << setprecision(4) << setw(3) << iter << setw(19) << a << setw(17) << b << setw(18) << newC << " " << "----" << endl; } else { double ea = (c - a) / c ; cout << setprecision(4) << setw(3) << iter << setw(19) << a << setw(17) << b << setw(18) << newC << " " << fixed << setprecision(4) <<100*ea << endl; } c = a-(function(a)*(b-a))/(function(b)-function(a)); // check for opposite sign if (function(a) * function(c) < 0.0f) { b = c; } else { a = c; } iter++; } auto stop = high_resolution_clock::now(); auto duration = duration_cast(stop - start); return 0; } static double function(double x) { return pow(x, 3) - x - 1; }
Please correct the answer for column Ea%.For 1st iteration it will display "---" For 2nd iteration, 1.0333-1/1.0333 times 100 = 3.22, For 3rd iteration, 1.0641-1.0333/1.0641 times 100 = 2.89. As observed for Ea the values used are from the previous row. The output table at inputs 1,5,4 is:
The code:
#include <iostream> #include <math.h> #include<iomanip> #include<chrono> #include <cstdlib> using namespace std::chrono; using namespace std; static double function(double x); // function f(x) void menu(); int main() { double a; // Lower Guess or beginning of interval double b; // Upper Guess or end of interval double c = 0; // variable for midpoint double terms; double newc; // Taking Input cout << "Enter Xn-1: "; cin >> a; cout << "\nEnter Xn: "; cin >> b; cout << "\nEnter number of iterations: "; cin >> terms; // Check for opposite sign (Intermediate Value Theorem) if (function(a) * function(b) > 0.0f) { cout << "\nFunction has same signs at ends of interval"; return -1; } int iter = 1; cout << setw(3) << "\n\n N (iterations)" << setw(9) << "Xn-1" << setw(17) << "Xn" << setw(18) << "Xn+1" << " Ea% " << endl; auto start = high_resolution_clock::now(); while (terms >= iter) // terminating condition { double newC = a-(function(a)*(b-a))/(function(b)-function(a)); if (c == 0) { cout << setprecision(4) << setw(3) << iter << setw(19) << a << setw(17) << b << setw(18) << newC << " " << "----" << endl; } else { double ea = (c - a) / c ; cout << setprecision(4) << setw(3) << iter << setw(19) << a << setw(17) << b << setw(18) << newC << " " << fixed << setprecision(4) <<100*ea << endl; } c = a-(function(a)*(b-a))/(function(b)-function(a)); // check for opposite sign if (function(a) * function(c) < 0.0f) { b = c; } else { a = c; } iter++; } auto stop = high_resolution_clock::now(); auto duration = duration_cast<microseconds>(stop - start); return 0; } static double function(double x) { return pow(x, 3) - x - 1; }


Step by step
Solved in 3 steps with 1 images

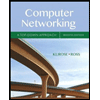
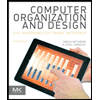
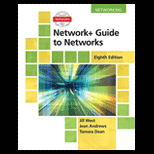
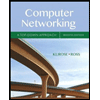
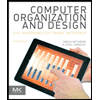
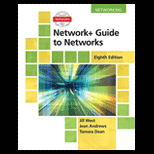
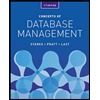
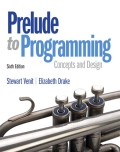
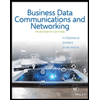