Person and Employee class are already in this activity. No need to add those classes to your code. For example: Test Result CommissionEmployee c = new
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Make Java Abstract CommissionEmployee class
- provide the setters and getters of your data members
- implement abstract method
- Override the toString and equals of Employee
Note:
Person and Employee class are already in this activity. No need to add those classes to your code.
For example:
Test | Result |
---|---|
CommissionEmployee c = new CommissionEmployee ("Yule Josef Ato","Cebu City",23,"Accenture",40000.0f, 550, 0.10f); System.out.println(c); System.out.println("\nEarnings: "+c.earnings()); |
Name: Yule Josef Ato Address: Cebu City Age: 23 Company name: Accenture Regular salary: 40000.0 Item sold: 550 Commission rate: 0.1 Earnings: 40055.0 |
CommissionEmployee c = new CommissionEmployee ("Yule Josef Ato","Cebu City",23,"Accenture",40000.0f, 550, 0.10f); CommissionEmployee c2 = new CommissionEmployee ("Yule Josef Ato","Cebu City",23,"Accenture",40000.0f, 550, 0.10f); c.display(); System.out.println(c.equals(c2)); |
Name : Yule Josef Ato Address : Cebu City Age : 23 Company Name: Accenture true |
Complete the code:
class CommissionEmployee extends Employee {
private float regularSalary; //fixed monthly salary
private int itemSold; //total number of items sold
private float commissionRate; //rate per item (in decimal form)
public CommissionEmployee(String name,String address,
int age,String cName, float regSal, int nItems,
float commission) {
regularSalary = regSal;
itemSold = nItems;
commissionRate = commission;
}
public CommissionEmployee() {
}
// provide the setters and getters of your data members
//the total earnings of a commission employee is the sum of
//the monthly salary plus the commission.
//commission will be based on the total number of items sold
//times the commission rate per item.
// override the toString() and equals() methods
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

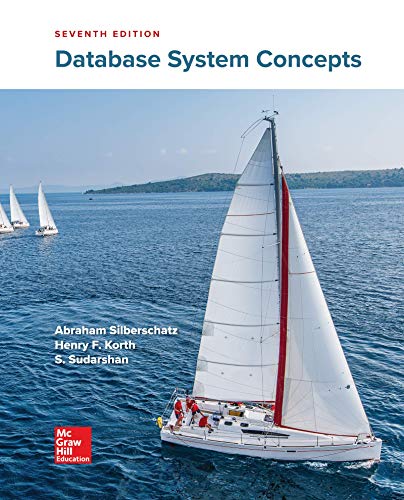
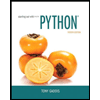
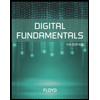
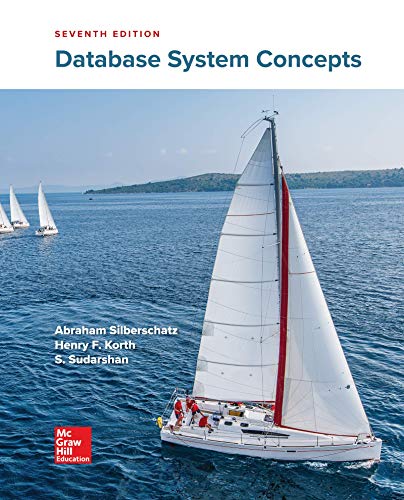
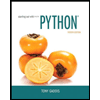
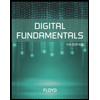
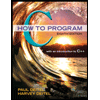
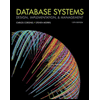
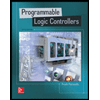