Part B - Searching and Sorting 1. Create a search method in your program that allows the user to find a tax payer and display their information to the user. Change your program to prompt the user for a name to find. Have the main method call this method. 2. Add code to your program to sort the information according to salary in ascending order. Save the sorted information into a new file.
Please help how would incorporate what is asked below into the java program below. Please read the quest carefully. I have posted both the description of what to do and the output that my program bring out which is a ceos full name, there company, salary and tax owed
java program is below
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Scanner;
public class Demo{
//Method to calculate the tax
static public double calcTax(double salary , double taxRate){
double taxOwed = 0;
//Calclate and return the tax
taxOwed = (salary * taxRate) / 100;
//return the value
return taxOwed;
}
//Main method
public static void main(String[] args) throws IOException{
//Create three array to add the data
ArrayList<Double> salary = new ArrayList<Double>();
ArrayList<String> name = new ArrayList<String>();
ArrayList<String> company_name = new ArrayList<String>();
//to count the lines
int lines = 1;
try {
//Open the file
File myFile = new File("taxpayer.txt");
//to read the file
Scanner fileReader = new Scanner(myFile);
//Check if file has next line
while (fileReader.hasNextLine()) {
//get the line into the string data
String data = fileReader.nextLine();
//Check the line number before adding that into the array
if(lines % 3 == 1){
//FOr the lines 1 , 4 , 7 add them into the name array
name.add(data);
}else if(lines % 3 == 2 ){
//for line 2 , 5 , 8 add them into the company_name array
company_name.add(data);
}else{
//FOr lines 3, 6 , 9 add them into the salary array
//First parse the value into double before adding
double sal =Double.parseDouble(data);
salary.add(sal);
}
//Increment the number of line
lines++;
}
//Close the file
fileReader.close();
//Check if any exceptions is there
} catch (FileNotFoundException e) {
System.out.println("File with given name not found");
e.printStackTrace();
}
try (//Now we have to add the records into the new File
//Create the my file writer class object to write into the file
FileWriter myWriter = new FileWriter("taxInfo.txt",true)) {
System.out.println("Records started in file .....");
//Iterate over the list to add the data into the file
for (int i = 0 ; i < name.size() ; i++){
//Get the data to add into the file
String nameP = name.get(i);
String companyName = company_name.get(i);
double salaryP = salary.get(i);
double taxRate = 0 , taxOwed = 0;
//Decide the tax rate
if(salary.get(i) <= 10000000){
taxRate = 40;
}else{
taxRate = 53;
}
//Calculate the taxOwed
taxOwed = calcTax(salary.get(i), taxRate);
//Convert to string
String tax =String.valueOf(taxOwed);
//Write to file
myWriter.write(nameP+"\n"+companyName+"\n"+salaryP+"\n"+tax+"\n");
System.out.println(nameP+"\n"+companyName+"\n"+salaryP+"\n"+tax+"\n");
}
}
}
}



Step by step
Solved in 2 steps with 1 images

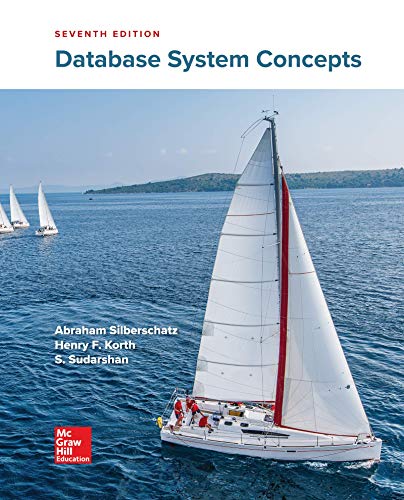
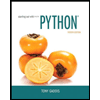
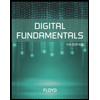
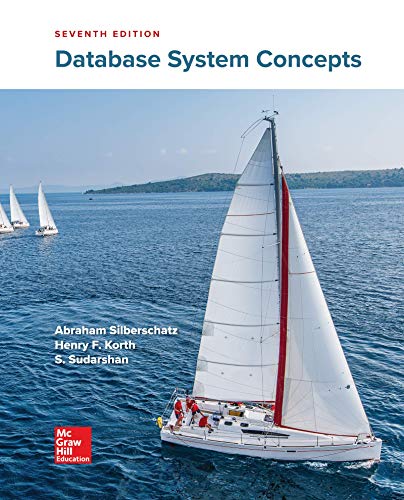
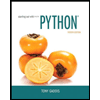
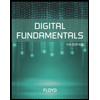
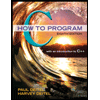
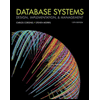
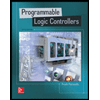