Part (1): In this program, you are going to write a program to simulate the customer flows in the supermarket. The following is the function prototype which is given: int act(int type, int NumCustomers); This function will do the following jobs: if act is called with type=1, print out “a custom enters the supermarket”, NumCustomers++, return NumCustomers; if act is called with type=-1, that means a custom left the supermarket, if there are still customers in the supermarket, do: NumCustomers--; otherwise, print out “No Customers”; return NumCustomers The following is the given main function, please complete the program. #include using namespace std; //functions prototype int main() { int NumCustomers=30; NumCustomers = act(1, NumCustomers); cout<< NumCustomers <
Part (1):
In this program, you are going to write a program to simulate the customer flows in the supermarket. The following is the function prototype which is given:
int act(int type, int NumCustomers);
This function will do the following jobs:
if act is called with type=1, print out “a custom enters the supermarket”, NumCustomers++, return NumCustomers;
if act is called with type=-1, that means a custom left the supermarket, if there are still customers in the supermarket, do: NumCustomers--; otherwise, print out “No Customers”; return NumCustomers
The following is the given main function, please complete the program.
#include<iostream>
using namespace std;
//functions prototype
int main()
{
int NumCustomers=30;
NumCustomers = act(1, NumCustomers);
cout<< NumCustomers <<endl;
NumCustomers = act(1, NumCustomers);
NumCustomers = act(1, NumCustomers);
cout<< NumCustomers <<endl;
NumCustomers = act(-1, NumCustomers);
NumCustomers = act(-1, NumCustomers);
NumCustomers = act(1, NumCustomers);
cout<< NumCustomers <<endl;
return 0;
}
//Complete the functions here:
Part (2)
Modify your function prototype to the following:
void act(int type, int& num);
- This function will do the same jobs as Part (1) except that no value will be returned since it is a void function.
- Change your main function properly to implement the same task.
Part (3)
In your program of Part (2), modify your function prototype to the following:
void act(int type, int num);
keep the main function same as Part (2), then run your program again, is there any difference in your output? Why does this happen?
Part (4) Now you will implement this program by using global variable. The steps are as follows:
- Declare a global variable NumCustomers.
- Modify your function act, and only keep one parameter which is type as follows.
void act(int type);
- Modify your main function as follows, add global variable NumCustomers, and then run the program and verify your solution.
#include<iostream>
using namespace std;
//declare global variable NumCustomers here:
//function prototype
int main()
{
act(1);
cout<< NumCustomers <<endl;
act(1);
act(1);
cout<< NumCustomers <<endl;
act(-1);
act(-1);
act(1);
cout<< NumCustomers <<endl;
return 0;
}
//Complete function definition here
void act(int type)
{
//if act is called with type=1, that means a custom entered the supermarket, NumCustomers++;
//if act is called with type=-1, that means a custom left the supermarket, if there are still customers in the supermarket, do: NumCustomers--; otherwise, print out “No Customers”;
}
Part (5) Now Modify your program without using any global variable: you will implement this program by using static variable. The steps are as follows:
- Modify your function act as follows.
int act(int type)
{
//declare NumCustomers as a static variable
//if act is called with type=1, that means a custom entered the supermarket, NumCustomers++;
//if act is called with type=-1, that means a custom left the supermarket, if there are still customers in the supermarket, do: NumCustomers--; otherwise, print out “No Customers”;
// return NumCustomers
}
- Modify your main function as follows, and then run the program and verify your solution.
#include<iostream>
using namespace std;
//function prototype
int main()
{
act(1);
cout<< act(0) <<endl;
act(1);
act(1);
cout<< act(0) <<endl;
act(-1);
act(-1);
act(1);
cout<< act(0) <<endl;
return 0;
}
//function definition
//please answer in c++

Step by step
Solved in 4 steps with 3 images

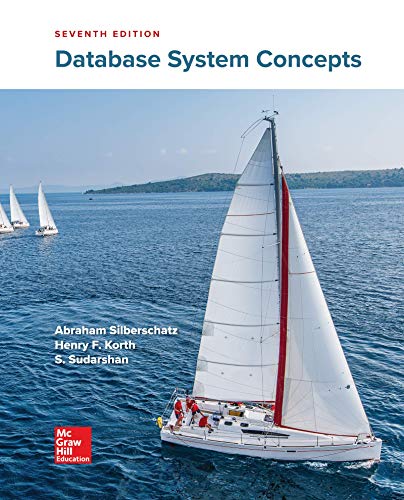
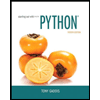
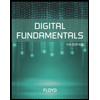
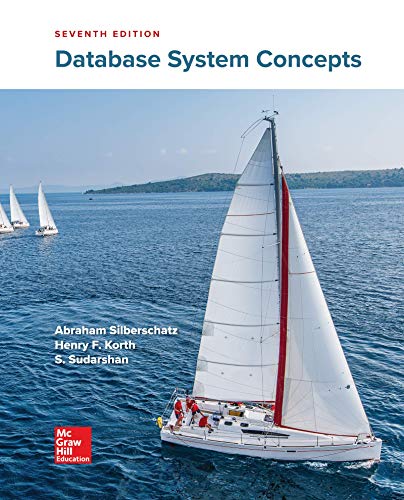
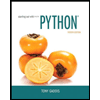
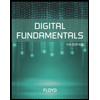
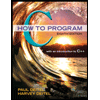
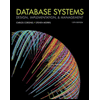
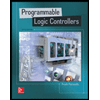