Palindrome Algorithm 1. Make sure to keep the original string. 2. Remove all punctuation, whitespace and special characters. 3. Convert the string to lower case.
Palindrome Algorithm 1. Make sure to keep the original string. 2. Remove all punctuation, whitespace and special characters. 3. Convert the string to lower case.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The code must be in c++. The template is below alongside the instructions.

Transcribed Image Text:Sample Program Output:
Enter your palindrome or type quit:
A man,
a plan, a canal, Panama.
Enter your palindrome or type quit:
Be at a time I emit a beat
Enter your palindrome or type quit:
Racecar
Enter your palindrome or type quit:
A lad named E. Mandala
Enter your palindrome or type quit:
A Toyota's a Toyota
Enter your palindrome or type quit:
Race and tell a dancer
Enter your palindrome or type quit:
quit
Palindromes:
A man,
a plan, a canal, Panama.
Racecar
A lad named E. Mandala
A Toyota's a Toyota
NOT Palindromes:
Be at a time I emit a beat
Race and tell a dancer
The space is created using a tab character.
Palindrome Algorithm
1. Make sure to keep the original string.
2. Remove all punctuation, whitespace and special characters.
3. Convert the string to lower case.
4. Create a reverse copy of the string.
5. Compare the clean version to the reverse version.
If the clean string equals the reverse, it is a palindrome
Otherwise it is not...
Make sure not to change the original string!
Program Functions
This prgram requires 6 functions:
boolean isPalindrome (string palindrome);
// isPalindrome passed the input string and calls all of the other
functions to prepare the string.
// Once the string is cleaned, it tests if it's a palindrome.
// @param string the string to check.
// @returns true if it's a palindrome and false if not.
string removePunctuation (string sentence);
// Create a copy of the string and remove punctuation from the copy
leaving the original alone.
// Use the cctype functions such as bool ispunct (char) and bool
isspace (char)
// @param sentence is the string to clean
// @returns string is the cleaned string.
string convertToLower(string mixedcase);
// Returns a lowercase version of the mixedcase string.
// @param mixedcase 1S
the

Transcribed Image Text:string convertToLower (string mixedcase);
// Returns a lowercase version of the mixedcase string.
// @param mixedcase is the string to convert.
// @returns a lowercase string.
string reverse (string input);
// Returns a
reverse version of the input string.
// @param input is the string to reverse
// @returns a string with the contents that's the reverse of the
input.
void display (vector<string> vstrings);
// Display the strings. Use a tab character to create the space seen
on Mimir.
// @param a vector of strings to display
// Precondition: a string to be tested
Remember to add necessary include files.
Main Program
int main() {
// TODO: Declare two vectors of strings to save the input
strings: palindromes, not_palindromes.
// ToDo: Implement a loop to read in the palindrome strings using
getline until the user quits.
// ToDo: In the loop, call the isPalindrome function on the input
string and store it in the
// palindromes vector if true and the notPalindromes vector if
false.
// ToDo: After exiting the loop, print the list of palindromes
under a Palindrome heading and
// the list that are not palindromes under a Not Palindrome
heading.
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
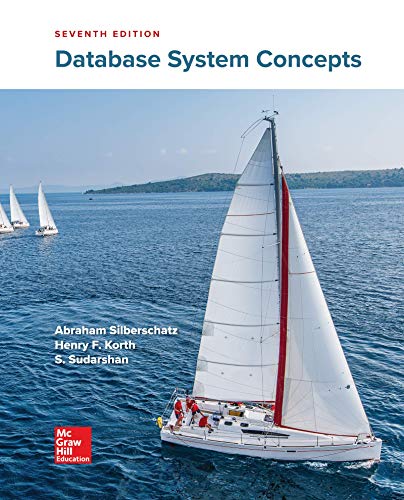
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
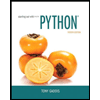
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
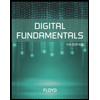
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
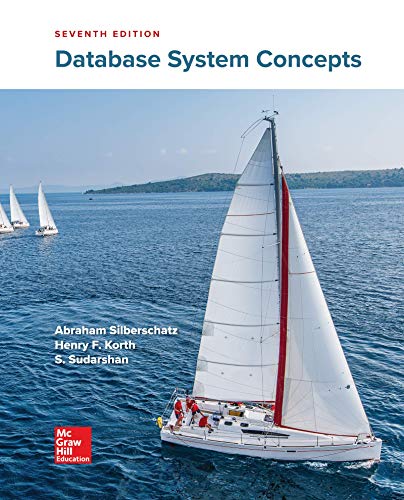
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
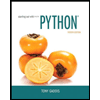
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
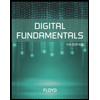
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
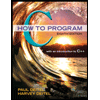
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
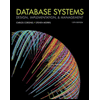
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
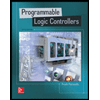
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education