OU ONLY NEED TO EDIT date.h, dateOFYear.h, and dateOFYear.cpp date.h | date.cpp | dateOfYear.h | dateOfYear.cpp | dateTest.cpp
C++
YOU ONLY NEED TO EDIT date.h, dateOFYear.h, and dateOFYear.cpp
date.h | date.cpp | dateOfYear.h | dateOfYear.cpp | dateTest.cpp
You have been provided a Date class. Each date contains day and month parameters, a default and a custom constructor, mutator and accessor functions for the parameters, and input and output functions called within operator >> and <<.
You will be writing a DateOfYear class, which expands on the Date class by adding a year parameter. This will be achieved via inheritance – DateOfYear is the derived class of the Date base class. In DateOfYear, will need to write…
- The include guards for the class
- A default constructor that initializes all parameters to 1
- A custom constructor that takes in a month, day, and year to initialize the parameters
- Mutator and accessor for the year parameter
- Redefined versions of inputDate and outputDate that takes into account the new year parameter.
The driver program, dateTest.cpp, is already set for use with both Date and DateOfYear. All you need to do is complete the DateOfYear class and make sure it is compatible with the date class.
HINT: The inputDate and outputDate functions are used within the operator >> and << functions inside of the Date class. Although you are redefining them in dateOfYear, you’ll need to do something to the base class header to make sure everything works correctly.
See the Sample Runs for reference (user input bolded and underlined):
Same Days
Enter month: 9
Enter day: 18
Enter month: 9
Enter day: 18
Enter year: 1995
9/18
2/27
9/18/1995
3/1/1996
d1 is your birthday!
Different Days
Enter month: 9
Enter day: 13
Enter month: 10
Enter day: 27
Enter year: 2017
9/13
2/27
10/27/2017
3/1/1996
These are different days.
date.cpp
//Date Class Implementation
#include "date.h"
// Friend Function of Date
bool equal(const Date& date1, const Date& date2)
{
return (date1.month == date2.month && date1.day == date2.day);
}
Date::Date():month(1), day(1)
{
}
Date::Date(int m, int d):month(m), day(d)
{
}
void Date::setMonth(int m)
{
month = m;
}
void Date::setDay(int d)
{
day = d;
}
double Date::getMonth() const
{
return month;
}
double Date::getDay() const
{
return day;
}
void Date::inputDate(istream& ins)
{
cout << "Enter month: ";
ins >> month;
cout << "Enter day: ";
ins >> day;
}
void Date::outputDate(ostream& outs) const
{
outs << month << '/' << day << endl;
}
istream& operator >>(istream& ins, Date& d)
{
d.inputDate(ins);
}
ostream& operator <<(ostream& outs, const Date& d)
{
d.outputDate(outs);
}
date.h
// Holds a month and a day to represent a date.
#ifndef DATE_H
#define DATE_H
#include <iostream>
using namespace std;
class Date
{
public:
friend bool equal(const Date& date1, const Date& date2);
//Precondition: date1 and date2 have values.
//Returns true if date1 and date2 represent the same date;
//otherwise, returns false.
Date();
Date(int m, int d);
void setMonth(int m);
void setDay(int d);
double getMonth() const;
double getDay() const;
void inputDate(istream& ins);
void outputDate(ostream& outs) const;
friend istream& operator >>(istream& ins, Date& d);
// Input Operator >>
// calls the inputDate function within here to perform input.
friend ostream& operator <<(ostream& outs, const Date& d);
// Output Operator <<
// Calls the outputDate function within here to perform output.
private:
int month;
int day;
};
#endif
dateOFYear.cpp
#include <iostream>
using namespace std;
#include "dateOfYear.cpp"
// Implement the member functions!
dateOFYear.h
#include "date.h"
#include <iostream>
using namespace std;
// Include Guards:x
class DateOfYear
{
public:
// Create Constructors Here
// Mutators and Accessor Functions Here
// inputDate and outputDate here, taking into account the new
private:
// Declare new data members here
};

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

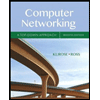
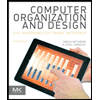
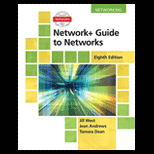
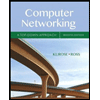
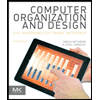
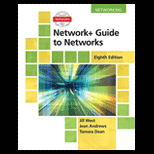
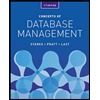
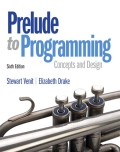
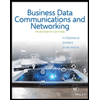