ou created a scatterplot of miles per gallon against weight; check to make sure it was included in your attachment. Does the graph show any trend? If yes, is the trend what you expected? Why or why not? See Step 2 in the Python script. What is the coefficient of correlation between miles per gallon and weight? What is the sign of the correlation coefficient? Does the coefficient of correlation indicate a strong correlation, weak correlation, or no correlation between the two variables? How do you know? See Step 3 in the Python script. Write the simple linear regression equation for miles per gallon as the response variable and weight as the predictor variable. How might the car rental company use this model? See Step 4 in the Python script. What is the slope coefficient? Is this coefficient significant at a 5% level of significance (alpha=0.05)? (Hint: Check the P-value, P>|t| , for weight in the Python output.) See Step 4 in the Python script.
ou created a scatterplot of miles per gallon against weight; check to make sure it was included in your attachment. Does the graph show any trend? If yes, is the trend what you expected? Why or why not? See Step 2 in the Python script. What is the coefficient of correlation between miles per gallon and weight? What is the sign of the correlation coefficient? Does the coefficient of correlation indicate a strong correlation, weak correlation, or no correlation between the two variables? How do you know? See Step 3 in the Python script. Write the simple linear regression equation for miles per gallon as the response variable and weight as the predictor variable. How might the car rental company use this model? See Step 4 in the Python script. What is the slope coefficient? Is this coefficient significant at a 5% level of significance (alpha=0.05)? (Hint: Check the P-value, P>|t| , for weight in the Python output.) See Step 4 in the Python script.
MATLAB: An Introduction with Applications
6th Edition
ISBN:9781119256830
Author:Amos Gilat
Publisher:Amos Gilat
Chapter1: Starting With Matlab
Section: Chapter Questions
Problem 1P
Related questions
Question
Answer based off of the information in the pictures.
- You created a scatterplot of miles per gallon against weight; check to make sure it was included in your attachment. Does the graph show any trend? If yes, is the trend what you expected? Why or why not? See Step 2 in the Python script.
- What is the coefficient of
correlation between miles per gallon and weight? What is the sign of thecorrelation coefficient ? Does the coefficient of correlation indicate a strong correlation, weak correlation, or no correlation between the two variables? How do you know? See Step 3 in the Python script. - Write the simple linear regression equation for miles per gallon as the response variable and weight as the predictor variable. How might the car rental company use this model? See Step 4 in the Python script.
- What is the slope coefficient? Is this coefficient significant at a 5% level of significance (alpha=0.05)? (Hint: Check the P-value, P>|t| , for weight in the Python output.) See Step 4 in the Python script.
![Step 1: Generating cars dataset
This block of Python code will generate the sample data for you. You will not be generating the dataset using numpy module this week. Instead, the dataset
will be imported from a CSV file. To make the data unique to you, a random sample of size 30, without replacement, will be drawn from the data in the CSV
file. The data set will be saved into a Python dataframe which you will use in later calculations.
Click the block of code below and hit the Run button above.
import pandas as pd
from IPython.display import display, HTML
#read data from mtcars.csv data set.
cars_df_orig = pd.read_csv("https://s3-us-west-2.amazonaws.com/data-analytics.zybooks.com/ntcars.csv")
#randomly pick 30 observations without replacement from mtcars dataset to make the data unique to you.
cars_df = cars_df_orig.sample(n=30, replace=False)
# print only the first five observations in the data set.
print("\nCars data frame (showing only the first five observations)")
display (HTML (cars_df.head().to_html()))
Cars data frame (showing only the first five observations)
Unnamed: 0 mpg cyl diep hp drat wt qeec ve am
3
0
110 3.08 3.215 19.44 1
113 3.77 1.513 16.90
1
1
Hornet 4 Drive 21.4 6 258.0
27 Lotus Europa 30.4 4 95.1
27.3 4 79.0
15.0
25
68 4.08
1.935 18.90
1
Fiat X1-9
Maserati Bora
30
8 301.0 335 3.54 3.570 14.60 0
6 Duster 380 14.3 8 380.0 245 3.21 3.570 15.84 0
#set a title for the plot, x-axis, and y-axis.
plt.title('MPG against Weight")
plt.xlabel('Weight (1000s lbs)')
plt.ylabel('MPG")
import matplotlib.pyplot as plt
#create scatterplot of variables mpg against wt.
plt.plot(cars_df["wt"], cars_df["mpg"], 'o', color="red")
#show the plot.
plt.show()
1
MPG against Weight
1
0
Step 2: Scatterplot of miles per gallon against weight
The block of code below will create a scatterplot of miles per gallon (coded as mpg in the data set) and weight of the car (coded as wt).
Click the block of code below and hit the Run button above.
NOTE: If the plot is not created, click the code section and hit the Run button again.
30 35 40
Weight (1000 lbs)
gear carb
3
5
4
5
3
1
2
1
8
4](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc697cfa7-35be-400d-88d6-26bf90a0b862%2F0cfe5856-e486-46ce-aebc-a1325d9cc723%2Figtxy3jf_processed.png&w=3840&q=75)
Transcribed Image Text:Step 1: Generating cars dataset
This block of Python code will generate the sample data for you. You will not be generating the dataset using numpy module this week. Instead, the dataset
will be imported from a CSV file. To make the data unique to you, a random sample of size 30, without replacement, will be drawn from the data in the CSV
file. The data set will be saved into a Python dataframe which you will use in later calculations.
Click the block of code below and hit the Run button above.
import pandas as pd
from IPython.display import display, HTML
#read data from mtcars.csv data set.
cars_df_orig = pd.read_csv("https://s3-us-west-2.amazonaws.com/data-analytics.zybooks.com/ntcars.csv")
#randomly pick 30 observations without replacement from mtcars dataset to make the data unique to you.
cars_df = cars_df_orig.sample(n=30, replace=False)
# print only the first five observations in the data set.
print("\nCars data frame (showing only the first five observations)")
display (HTML (cars_df.head().to_html()))
Cars data frame (showing only the first five observations)
Unnamed: 0 mpg cyl diep hp drat wt qeec ve am
3
0
110 3.08 3.215 19.44 1
113 3.77 1.513 16.90
1
1
Hornet 4 Drive 21.4 6 258.0
27 Lotus Europa 30.4 4 95.1
27.3 4 79.0
15.0
25
68 4.08
1.935 18.90
1
Fiat X1-9
Maserati Bora
30
8 301.0 335 3.54 3.570 14.60 0
6 Duster 380 14.3 8 380.0 245 3.21 3.570 15.84 0
#set a title for the plot, x-axis, and y-axis.
plt.title('MPG against Weight")
plt.xlabel('Weight (1000s lbs)')
plt.ylabel('MPG")
import matplotlib.pyplot as plt
#create scatterplot of variables mpg against wt.
plt.plot(cars_df["wt"], cars_df["mpg"], 'o', color="red")
#show the plot.
plt.show()
1
MPG against Weight
1
0
Step 2: Scatterplot of miles per gallon against weight
The block of code below will create a scatterplot of miles per gallon (coded as mpg in the data set) and weight of the car (coded as wt).
Click the block of code below and hit the Run button above.
NOTE: If the plot is not created, click the code section and hit the Run button again.
30 35 40
Weight (1000 lbs)
gear carb
3
5
4
5
3
1
2
1
8
4
![Step 3: Correlation coefficient for miles per gallon and weight
Now you will calculate the correlation coefficient between the miles per gallon and weight variables. The corr method of a dataframe returns the correlation
matrix with correlation coefficients between all variables in the dataframe. In this case, you will specify to only return the matrix for the variables "miles per
gallon" and "weight".
Click the block of code below and hit the Run button above.
#create correlation matrix for mpg and wt.
#the correlation coefficient between mpg and wt is contained in the cell for mpg row and wt column (or wt row and mpg column)
mpg_wt_corr = cars_df[['mpg', 'wt']].corr()
print(mpg_wt_corr)
mpg
mpg 1.000000 -0.871718
wt -0.871718 1.000000
Step 4: Simple linear regression model to predict miles per gallon using weight
The block of code below produces a simple linear regression model using "miles per gallon" as the response variable and "weight" (of the car) as a predictor
variable. The ols method in statsmodels.formula.api submodule returns all statistics for this simple linear regression model.
Click the block of code below and hit the Run button above.
from statsmodels.formula.api import ols
#create the simple Linear regression model with mpg as the response variable and weight as the predictor variable
model = ols("mpg ~ wt', data=cars_df).fit()
wt
#print the model summary
print(model.summary())
Dep. Variable:
Model:
Method:
Date:
Time:
No. Observations:
Df Residuals:
Df Model:
Covariance Type:
======
Intercept
wt
Omnibus:
Prob (Onnibus):
Skew:
Kurtosis:
coef
37.3171
-5.3136
OLS Regression Results
mpg R-squared:
OLS
Least Squares
Thu, 08 Dec 2022
03:43:58
nonrobust
std err
30
28
1
1.893
0.564
Adj. R-squared:
F-statistic:
Prob (F-statistic):
Log-Likelihood:
AIC:
BIC:
t
19.717
-9.414
P>|t|
2.571
0.276
0.636
2.807 Cond. No.
===========
0.000
0.000
Durbin-Watson:
Jarque-Bera (JB):
Prob(JB):
[0.025
33.440
-6.470
0.760
0.751
88.61
3.59e-10
-75.181
154.4
157.2
=========
0.975]
41.194
-4.157
2.078
2.068
0.356
12.2
(========
Warnings:
[1] Standard Errors assume that the covariance matrix of the errors is correctly specified.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc697cfa7-35be-400d-88d6-26bf90a0b862%2F0cfe5856-e486-46ce-aebc-a1325d9cc723%2Fnx93hrn_processed.png&w=3840&q=75)
Transcribed Image Text:Step 3: Correlation coefficient for miles per gallon and weight
Now you will calculate the correlation coefficient between the miles per gallon and weight variables. The corr method of a dataframe returns the correlation
matrix with correlation coefficients between all variables in the dataframe. In this case, you will specify to only return the matrix for the variables "miles per
gallon" and "weight".
Click the block of code below and hit the Run button above.
#create correlation matrix for mpg and wt.
#the correlation coefficient between mpg and wt is contained in the cell for mpg row and wt column (or wt row and mpg column)
mpg_wt_corr = cars_df[['mpg', 'wt']].corr()
print(mpg_wt_corr)
mpg
mpg 1.000000 -0.871718
wt -0.871718 1.000000
Step 4: Simple linear regression model to predict miles per gallon using weight
The block of code below produces a simple linear regression model using "miles per gallon" as the response variable and "weight" (of the car) as a predictor
variable. The ols method in statsmodels.formula.api submodule returns all statistics for this simple linear regression model.
Click the block of code below and hit the Run button above.
from statsmodels.formula.api import ols
#create the simple Linear regression model with mpg as the response variable and weight as the predictor variable
model = ols("mpg ~ wt', data=cars_df).fit()
wt
#print the model summary
print(model.summary())
Dep. Variable:
Model:
Method:
Date:
Time:
No. Observations:
Df Residuals:
Df Model:
Covariance Type:
======
Intercept
wt
Omnibus:
Prob (Onnibus):
Skew:
Kurtosis:
coef
37.3171
-5.3136
OLS Regression Results
mpg R-squared:
OLS
Least Squares
Thu, 08 Dec 2022
03:43:58
nonrobust
std err
30
28
1
1.893
0.564
Adj. R-squared:
F-statistic:
Prob (F-statistic):
Log-Likelihood:
AIC:
BIC:
t
19.717
-9.414
P>|t|
2.571
0.276
0.636
2.807 Cond. No.
===========
0.000
0.000
Durbin-Watson:
Jarque-Bera (JB):
Prob(JB):
[0.025
33.440
-6.470
0.760
0.751
88.61
3.59e-10
-75.181
154.4
157.2
=========
0.975]
41.194
-4.157
2.078
2.068
0.356
12.2
(========
Warnings:
[1] Standard Errors assume that the covariance matrix of the errors is correctly specified.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
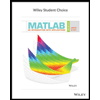
MATLAB: An Introduction with Applications
Statistics
ISBN:
9781119256830
Author:
Amos Gilat
Publisher:
John Wiley & Sons Inc
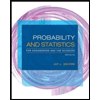
Probability and Statistics for Engineering and th…
Statistics
ISBN:
9781305251809
Author:
Jay L. Devore
Publisher:
Cengage Learning
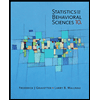
Statistics for The Behavioral Sciences (MindTap C…
Statistics
ISBN:
9781305504912
Author:
Frederick J Gravetter, Larry B. Wallnau
Publisher:
Cengage Learning
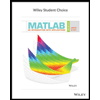
MATLAB: An Introduction with Applications
Statistics
ISBN:
9781119256830
Author:
Amos Gilat
Publisher:
John Wiley & Sons Inc
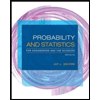
Probability and Statistics for Engineering and th…
Statistics
ISBN:
9781305251809
Author:
Jay L. Devore
Publisher:
Cengage Learning
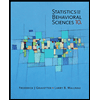
Statistics for The Behavioral Sciences (MindTap C…
Statistics
ISBN:
9781305504912
Author:
Frederick J Gravetter, Larry B. Wallnau
Publisher:
Cengage Learning
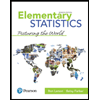
Elementary Statistics: Picturing the World (7th E…
Statistics
ISBN:
9780134683416
Author:
Ron Larson, Betsy Farber
Publisher:
PEARSON
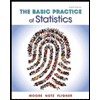
The Basic Practice of Statistics
Statistics
ISBN:
9781319042578
Author:
David S. Moore, William I. Notz, Michael A. Fligner
Publisher:
W. H. Freeman
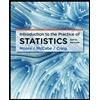
Introduction to the Practice of Statistics
Statistics
ISBN:
9781319013387
Author:
David S. Moore, George P. McCabe, Bruce A. Craig
Publisher:
W. H. Freeman