Option 2: Blackjack Write a program that scores a blackjack hand. In blackjack, a player receives from two to five cards. (The player decides how many, but that has no effect t on this exercise.) The cards 2 through 10 are scored as 2 through 10 points each. The face cards, jack, queen, and king are scored as 10 points. The goal is to come as close to a score of 21 as possible without going over 21. Hence, any score over 21 is called "busted." The ace can count as either 1 or 11, whichever is better for the user. For example, an ace and a 10 can be scored as either 11 or 21. Since 21 is a better score, this hand is scored as 21. An ace and two 8' s can be scored as either 17 or 27. Since 27 is a "busted" score, this hand is scored as 17. The user is asked how many cards she or he has, and the user responds with one of the integers 2, 3, 4, or 5. The user is then asked for the card values. Card values are 2 through 10, jack, queen, king, and ace. A good way to handle input is to use the type char so that the card input 2, for example, is read as the character '2', rather than as the number 2. Input the values 2 through 9 as the characters '2' through '9'. Input the values 10, jack, queen, king, and ace as the characters 't', 'j', 'q', 'k', and 'a'. An ace can also be entered as a '1'. (Of course, the user does not type in the single quotes.) Be sure to allow upper as well as lowercase letters as input. After reading in the values, the program should convert them from character values to numeric card scores, taking special care for aces. The output is either a number between 2 and 21 (inclusive) or the word Busted. Use functions where appropriate. Use a switch statement to determine the correct value for a card. Your program should include a loop that lets the user repeat this calculation until the user says she or he is done. Example Output: Enter the number of cards: 3 Enter cards: a78 The value of your hand is 16. Would you like to score another hand (Y or N)?: y Enter the number of cards: 4 Enter cards: 354K You are BUSTED! Would you like to score another hand (Y or N) ?: n Required Test Cases: 2, 87 2, ak 5, 12345 3, AAK 2, aa 4, 4567
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C++
Submission Requirements:
Use the “Steps for creating a
.txt or .rtf file and upload this with the other required files.
Use meaningful variable names and reasonable data types, const as appropriate.
Use spacing, indenting to increase program readability.
Include a comment section at the top of you file, with your name, the assignment number
and a brief summary (in your own words) of what the program does.
Include comments to describe the major sections of the program
Prompt for each input value you need


Step by step
Solved in 4 steps with 4 images

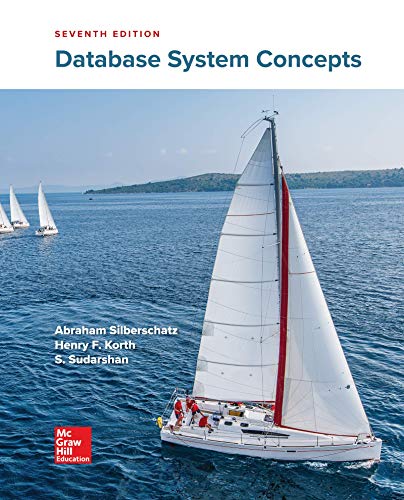
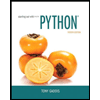
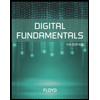
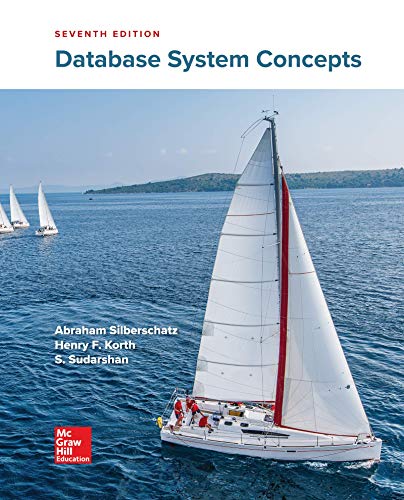
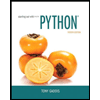
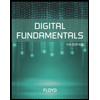
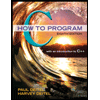
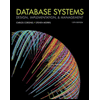
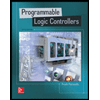