Only new java code can be added after the code give
Only new java code can be added after the code given:
The while loop makes multiple attempts to read a nonnegative integer from input into userAge. Use multiple exception handlers to:
- Catch an InputMismatchException, output "Unexpected input: The UserAge
program quits", and assign retry with false. - Catch an Exception and output the message of the Exception.
End each output with a newline.
Ex: If the input is 44, then the output is:
Valid input: User's age is 44
Ex: If the input is L, then the output is:
Unexpected input: The UserAge program quits
Ex: If the input is -65 44, then the output is:
User's age must be nonnegative Valid input: User's age is 44
import java.util.Scanner;
import java.util.InputMismatchException;
public class UserAge {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int userAge;
boolean retry = true;
while (retry) {
try {
userAge = scnr.nextInt();
if (userAge < 0) {
throw new Exception("User's age must be nonnegative");
}
retry = false;
System.out.print("Valid input: ");
System.out.println("User's age is " + userAge);
}
/******************************************************************************************************* New Java Code Here ; Plese test input

Initialize Variables:
- Create a
Scanner
object (scnr
) to read input from the console. - Declare an integer variable (
userAge
) to store the user's age. - Declare a boolean variable (
retry
) to control whether the program should retry or not.
- Create a
Main Loop (
while (retry)
):- This loop continues until
retry
is set tofalse
.
- This loop continues until
Input Reading Loop (
while (lineScanner.hasNext())
):- Read a line of input using
scnr.nextLine()
. - Create a new
Scanner
(lineScanner
) to scan the input line. - Enter a loop that continues as long as there are tokens in the input line.
- Read a line of input using
Token Processing:
- Attempt to read an integer from the token using
lineScanner.nextInt()
. - Check if the user's age is less than 0. If so, throw an exception with the message "User's age must be nonnegative."
- Attempt to read an integer from the token using
Output Valid Input:
- If the input is valid (no exceptions thrown), print "Valid input: User's age is " followed by the user's age.
Exception Handling:
- Catch
InputMismatchException
and print "Unexpected input: The UserAge program quits." - Catch the general
Exception
and print the exception message along with a newline.
- Catch
Update Retry Flag:
- Set
retry
tofalse
to exit the main loop if the input processing is successful or if anInputMismatchException
occurs.
- Set
Step by step
Solved in 3 steps with 1 images

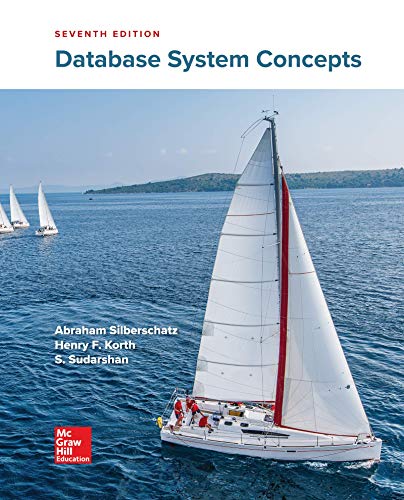
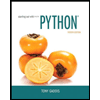
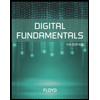
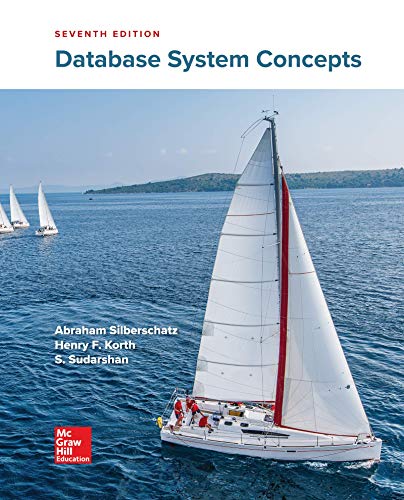
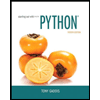
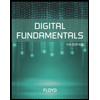
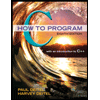
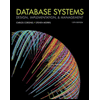
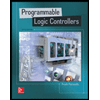