The while loop reads values from input until an integer is read. Complete the exception handler in the while loop to catch an InputMismatchException exception and: • Output "Removed unacceptable input for number of cents". End with a newline. • Discard the invalid input. Ex: If the input is 20 60, then the output is: 20 cents = 4 nickels Processed one valid input value Ex: If the input is Aya Tia 20 60, then the output is: Removed unacceptable input for number of cents Removed unacceptable input for number of cents 20 cents = 4 nickels Processed one valid input value Note: Each value read from input is on one line. 1 import java.util.Scanner; 2 import java.util.Input MismatchException; 3 4 public class Cents { 4567 5 public static void main(String[] args) { Scanner scnr = new Scanner (System.in); int numCents; boolean valueFound = false; 7 8 9 3456 WNHO6 10 11 12 13 14 15 16 17 while (!valueFound) { try { numCents = scnr.nextInt (); valueFound = true; System.out.println(numCents + "cents = " + (numCents / 5) + " nickels"); System.out.println("Processed one valid input value");
new java code can only be added after line 17.
![The while loop reads values from input until an integer is read. Complete the exception handler in the while loop to catch an InputMismatchException exception and:
- Output "Removed unacceptable input for number of cents". End with a newline.
- Discard the invalid input.
Ex: If the input is 20 60, then the output is:
```
20 cents = 4 nickels
Processed one valid input value
```
Ex: If the input is Aya Tia 20 60, then the output is:
```
Removed unacceptable input for number of cents
Removed unacceptable input for number of cents
20 cents = 4 nickels
Processed one valid input value
```
Note: Each value read from input is on one line.
```java
import java.util.Scanner;
import java.util.InputMismatchException;
public class Cents {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int numCents;
boolean valueFound = false;
while (!valueFound) {
try {
numCents = scnr.nextInt();
valueFound = true;
System.out.println(numCents + " cents = " + (numCents / 5) + " nickels");
System.out.println("Processed one valid input value");
}
}
}
}
```](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2Fcc1c7da6-23d3-4f8b-b5ac-8c9700860e31%2F6wneqs_processed.png&w=3840&q=75)

1. Start
2. Initialize Scanner object scnr to read input from the user.
3. Declare an integer variable numCents and a boolean variable valueFound, both initially set to false.
4. Enter a while loop with condition !valueFound to repeatedly prompt for input until a valid input is provided.
a. Inside the loop:
i. Try to read an integer from the user using scnr.nextInt().
ii. If no exception occurs, set valueFound to true.
- Print numCents + " cents = " + (numCents / 5) + " nickels"
- Print "Processed one valid input value"
iii. If an InputMismatchException occurs:
- Print "Removed unacceptable input for number of cents"
- Discard the invalid input using scnr.nextLine()
5. End
Step by step
Solved in 4 steps with 2 images

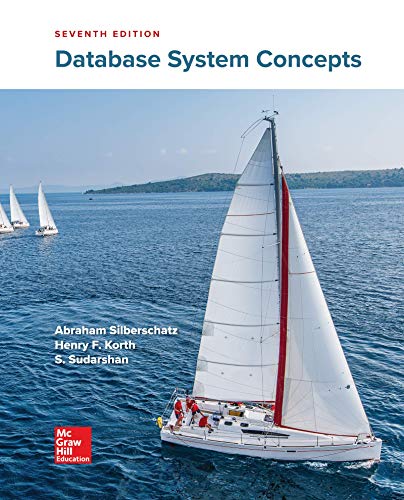
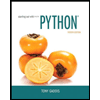
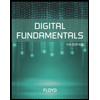
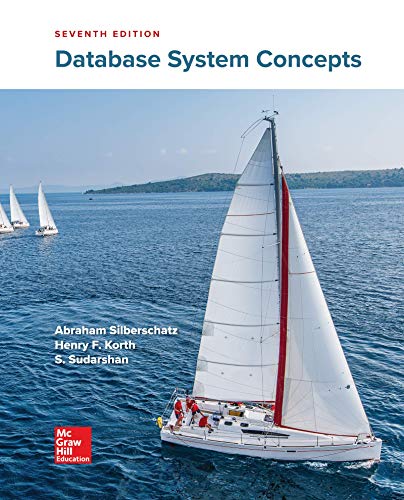
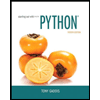
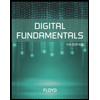
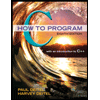
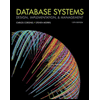
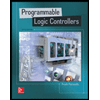