odeling the spread of a virus like COVID-19 using recursion. Let N = total population (assumed constant, disregarding deaths, births, immigration, and emigration). S n = number who are susceptible to the disease at time n (n is in weeks). I n = number who are infected (and contagious) at time n.
odeling the spread of a virus like COVID-19 using recursion. Let N = total population (assumed constant, disregarding deaths, births, immigration, and emigration). S n = number who are susceptible to the disease at time n (n is in weeks). I n = number who are infected (and contagious) at time n.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Modeling the spread of a virus like COVID-19 using recursion.
Let
N = total population (assumed constant, disregarding deaths, births, immigration, and emigration).
S
n
= number who are susceptible to the disease at time n (n is in weeks).
I
n
= number who are infected (and contagious) at time n.
R
n
= number who are recovered (and not contagiuous) at time n.
The total population is divided between these three groups:
N = S
n
+ I
n
+ R
n
There are several hidden assumptions here that may or may not apply to COVID-19, such as a recovered
person is assumed to not be able to get the disease a second time, at least within the time window being
examined.
On week 0 (the start), you assume a certain small number of people have the infection (just to get things
going). Everyone else is initially susceptible, and no one is recovered.
There are two constants of interest:
Let period = time period that it takes for an infected person to recover (recover meaning they become
not infectious to someone else).
Let ratio = infection ratio (an infected person can infect this many others in period weeks),
assuming the
entire population was susceptible)
.
We can deduce three equations that track how S, I, and R change from time period n to time period n+1:
First it may be helpful to define three intermediate variables.
The infection ratio is for the entire infection period, so the weekly infection ratio would be a fraction of
that, ratio / period. Also, since the infection ratio is the ratio if all the population was susceptible, the
effective ratio is really this ratio scaled down for the percent of the population that is susceptible, S
n
/ N:
(A) effectiveWeeklyRatio = ratio/period * S
n
/N
The number of new infections will be the number currently infected times the effectiveWeeklyRatio:
(B) newInfections = I
n
* effectiveWeeklyRatio
On average 1/period of the infected people will recover in a single time period:
(C) newRecovered = I
n
/ period
Using these intermediate variables you calculate new values for R, S, and I:
(1) R
n+1
= R
n
+ newRecovered
(2) S
n+1
= S
n
– newInfections
(3) I
n+1
= I
n
+ newInfections − newRecovered
Instead of using this equation, perhaps better:
(3) I
n+1
= N − R
n+1
− S
n+1
(keeps the total number N constant even after rounding errors)
You will also maintain a “total” variable to add together those that ever got the virus at any point.
total
+= newInfections
These equations lend themselves naturally to recursion. The recursive function is passed values of the
variables for the previous week and calculates new values for the variables for the current week.
For the this project, you will input a value for ratio.
You can assume N = 1000000 (one million) and period=1.0 (one week)
Use initial values of I=100, R=0, S=999900, week=0, and total=100 just to get things going.
Call a function named NextWeek recursively for each new week. Show in three columns the week, the
number of new infections, and the running total of new infections. Right justify each column in a field of
appropriate width. Stop when the number of new infections equals zero.
Note that most values are integers, except that period, ratio, and weeklyEffectiveRatio are floating-point
(doubles). You may need to force a division to be floating point, and you will need to round some
answers off to the nearest integer. To round a double to int, use #include <math.h>
(#include <cmath> for c++) and use the round() function. The function will return the total (all the
way back up the recursive tree so main can get it).
int NextWeek(I, R, S, N, total, ratio, period, week)
{
declare variables of appropiate type:
weeklyEffectiveRatio, newRecovered, newInfections
increment week
calc weeklyEffectiveRatio
calc newRecovered (remember to round to int)
calc newInfections (remember to round to int)
add newInfections to total
calc new values for I, R, S
print week, newInfections, total in asked for format
if (base case)
return total
return NextWeek(..)
}
COVID NOTES:
The estimates for ratio range from about 2.5 to about 5.
The estimates for the period that one is infectious vary widely, it is probably more than the 1.0 week
given here. Assuming you keep ratio the same, the period doesn’t affect the total that eventually get the
disease much, it just shortens or lengthens the total time for the course of the disease,.
Example output/input (assuming a value for r of 2.5),
showing the first few lines:
Enter r:
2.5
WEEK NEW CASES TOTAL CASES
---- ----------- -----------
1 250 350
2 625 975
3 1561 2536
4 3893 6429
5 9670 16099
(only first five weeks shown here, but program goes until zero new cases)
Note that there are two lines of header that are printed out once as literal strings (in main):
"
WEEK NEW CASES TOTAL CASES
"
"---- ----------- -----------"
Then for each week,
Right justified width 4 for the week
Right justified width 12 for new cases
Right justified width 12 for total cases
Submission
Submit one file in D2L under Assignments:
Submit your source code in one file (named with an extension .c for C, .cpp for C++).
I will run the program, so you don't need to supply the output
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
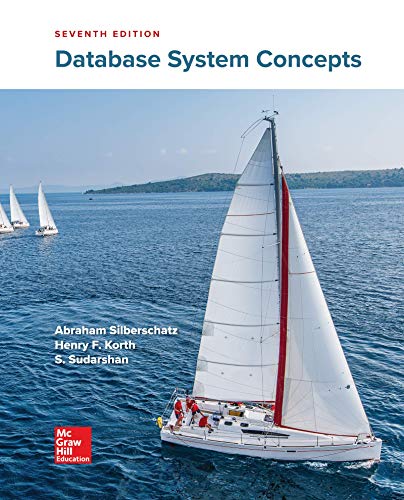
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
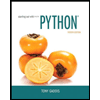
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
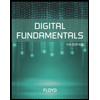
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
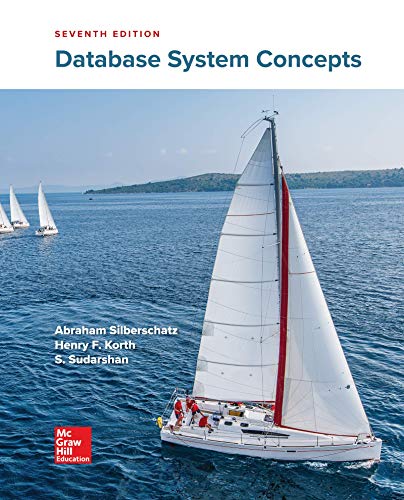
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
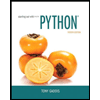
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
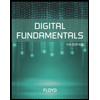
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
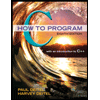
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
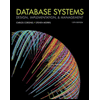
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
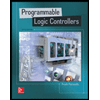
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education