Objectives After this lab assignment, students should be able to: Design modular programs based on specifications Define and call functions with conditional logic Write programs that use functions ● Background A bank uses the following flowchart to determine whether a customer is eligible for a loan. It contains four steps, separated by color. 0 0 0 0 double askAmount() 0 0 double askincome() How much would you like to borrow? cin >> loan if loan < 0 Please enter positive # What is your yearly Income? cin >> income if income < 0 Please enter positive # askLoan() Return type: bool Parameter(s): None askIncome() Return type: double (loan) Parameter(s): None decideLoan() Yes 0 Return type: bool 0 Would you like to take out a loan? START HERE Thanks for visiting the bank! Return type: double (income) Parameter(s): None askAmount() 0 0 Yes/Y/yes/y or No/N/no/n No ● Instructions: You will use the flowchart to design and write a program that uses a function for each step. Write a program named bankloan.cpp that defines and calls the following functions, EXACTLY as specified below: if income <= 10,000 REJECT if income > 10,000 and income < 100,000 if income >= 100,000 ACCEPT bool askLoan() bool decideLoad(double income, double loan) Invalid choice Please enter yes or no if income * 5 >= loan ACCEPT if income * 5 < loan REJECT Parameter(s): double (income), double (loan) When validating the user input for askLoan(), Validate all user input using loops: accept the following user input for each choice: "Yes", "Y", "yes", and "y" returns true "No", "N", "no", and "n" returns false When validating the user input for askIncome()and askAmount(), make sure the input is greater than or equal to 0.



Algorithm:
1. Create a bool function called askLoan which will ask the user if they would like to take out a loan and return a boolean value.
2. Create a double function called askAmount which will ask the user how much they would like to borrow and return the value.
3. Create a double function called askIncome which will ask the user what their yearly income is and return the value.
4. Create a bool function called decideLoan which takes two double parameters, income and loan, and returns a boolean value.
5. In main, create a boolean variable called wantsLoan and assign it to the return value of askLoan.
6. If wantsLoan is false, print out an appropriate message and return 0.
7. Else, create a double variable called loan and assign it to the return value of askAmount.
8. Create a double variable called income and assign it to the return value of askIncome.
9. Create a boolean variable called isApproved and assign it to the return value of decideLoan.
10. If isApproved is true, print out an appropriate message.
11. Else, print out an appropriate message.
12. Return 0.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

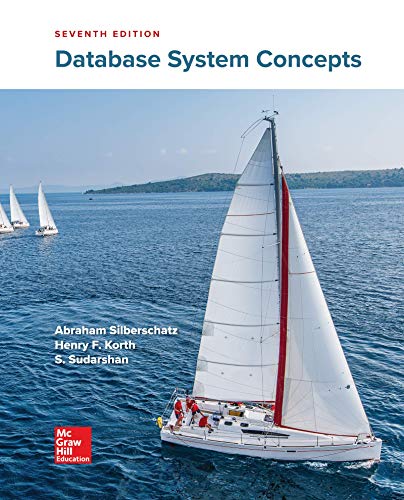
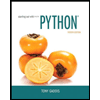
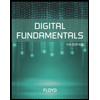
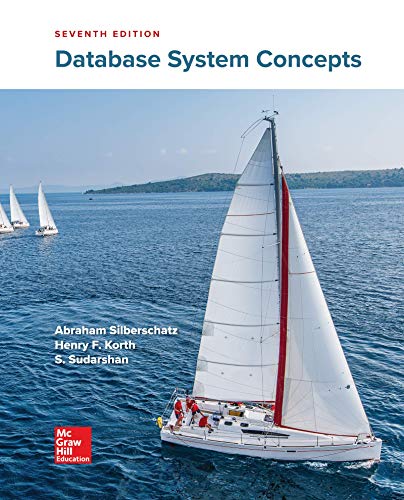
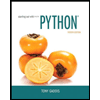
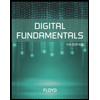
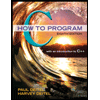
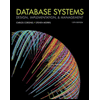
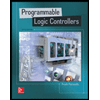