Suppose num is an int variable, declared and initialized. The value of num is between 2 and 12. Write a C# switch statement to output • "Game status is WON" if num is 7 or 11 • "Game status is LOST" if num is 2, 3 or 12 "Game status is CONTINUE" if num is 4, 5, 6, 8, 9 or 10
Suppose num is an int variable, declared and initialized. The value of num is between 2 and 12. Write a C# switch statement to output • "Game status is WON" if num is 7 or 11 • "Game status is LOST" if num is 2, 3 or 12 "Game status is CONTINUE" if num is 4, 5, 6, 8, 9 or 10
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C#

Transcribed Image Text:### Example of C# Switch Statement Based on Variable Value
Suppose a variable named `num` is declared and initialized as an integer in a C# program. The value of `num` ranges between 2 and 12. Depending on the value of `num`, we need to output different game statuses using a C# `switch` statement.
Here are the conditions for the game statuses:
- Output "Game status is WON" if `num` is 7 or 11.
- Output "Game status is LOST" if `num` is 2, 3, or 12.
- Output "Game status is CONTINUE" if `num` is 4, 5, 6, 8, 9, or 10.
### Example C# Code
Below is the example of the switch statement implementing the above logic:
```csharp
int num = // some integer value between 2 and 12;
switch (num)
{
case 7:
case 11:
Console.WriteLine("Game status is WON");
break;
case 2:
case 3:
case 12:
Console.WriteLine("Game status is LOST");
break;
case 4:
case 5:
case 6:
case 8:
case 9:
case 10:
Console.WriteLine("Game status is CONTINUE");
break;
default:
Console.WriteLine("Invalid number");
break;
}
```
### Explanation
The `switch` statement evaluates the variable `num` and matches it against various `case` labels:
- If `num` is 7 or 11, the output will be "Game status is WON".
- If `num` is 2, 3, or 12, the output will be "Game status is LOST".
- If `num` is 4, 5, 6, 8, 9, or 10, the output will be "Game status is CONTINUE".
- If the value of `num` does not match any of the specified cases, the `default` case will be executed, which outputs "Invalid number". Although the value of `num` is presumed to be between 2 and 12, including a `default` case is good practice to handle unexpected values.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
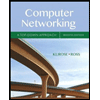
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
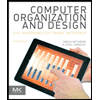
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
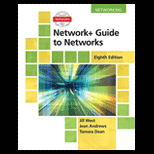
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
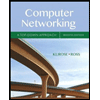
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
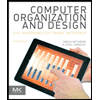
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
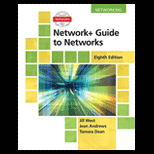
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
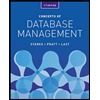
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
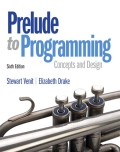
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
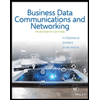
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY