Objective: Write a program in Python using wrapper API to get weather information. Step 1.1: Get PyOWM API key Follow these steps to get a weather info API key from Open Weather Map.org: Go to openweathermap.org/appid Under “Sign up and call API for free”, click “Sign up” Register. Click “Create an Account”. Remember your login and password. They may send a confirmation link to the email you used to register. Use it to confirm. Go to “API keys”. Copy key from gray box into a text file and save it for later. You will insert the key into the provided Python code that accesses the API. Step 1.2. Install PyOWM module on your computer Follow these steps to install PyOWM module on your computer: Start your terminal (Windows users start WSL terminal or Windows Console). Type pip3 install pyowm (the module should install). Step 1.3: Download, Add Code, and Test interactive App You should use the starter app weather.py. It includes a predefined function getweather() that calls OWM’s Web-API to get today’s temperatures (in Fahrenheit) for the specified city and return them in a dictionary with keys “temp_max”, “temp”, and “temp_min”. Do not edit getweather(). Insert your API key where indicated. Add code in main() that asks users for a city and country, calls getweather() with the city and country, prints the min, current, and max temperatures from the returned dictionary in both Fahrenheit and Celsius ( (°F - 32) / 1.8 = °C), then asks the user if they want to check another city (with input validation). The program’s input and output should look like this if the user enters Y or N (but if the user enters anything other than Y or N, it asks again): Check temperature in cities worldwide City: Paris Country: France The max temperature: 42.01 F 5.56 C The current temperature: 36.39 F 2.44 C The minimum temperature: 28.99 F -1.67 C Check the weather in another city? (Y or N): Y City: NY Country: USA
Objective: Write a program in Python using wrapper API to get weather information.
Step 1.1: Get PyOWM API key
Follow these steps to get a weather info API key from Open Weather Map.org:
- Go to openweathermap.org/appid
- Under “Sign up and call API for free”, click “Sign up”
- Register. Click “Create an Account”. Remember your login and password.
- They may send a confirmation link to the email you used to register. Use it to confirm.
- Go to “API keys”.
- Copy key from gray box into a text file and save it for later. You will insert the key into the provided Python code that accesses the API.
Step 1.2. Install PyOWM module on your computer
Follow these steps to install PyOWM module on your computer:
- Start your terminal (Windows users start WSL terminal or Windows Console).
- Type pip3 install pyowm (the module should install).
Step 1.3: Download, Add Code, and Test interactive App
You should use the starter app weather.py. It includes a predefined function getweather() that calls OWM’s Web-API to get today’s temperatures (in Fahrenheit) for the specified city and return them in a dictionary with keys “temp_max”, “temp”, and “temp_min”. Do not edit getweather(). Insert your API key where indicated. Add code in main() that asks users for a city and country, calls getweather() with the city and country, prints the min, current, and max temperatures from the returned dictionary in both Fahrenheit and Celsius ( (°F - 32) / 1.8 = °C), then asks the user if they want to check another city (with input validation). The program’s input and output should look like this if the user enters Y or N (but if the user enters anything other than Y or N, it asks again):
Check temperature in cities worldwide
City: Paris
Country: France
The max temperature: 42.01 F 5.56 C
The current temperature: 36.39 F 2.44 C
The minimum temperature: 28.99 F -1.67 C
Check the weather in another city? (Y or N): Y
City: NY
Country: USA


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

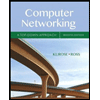
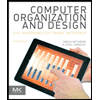
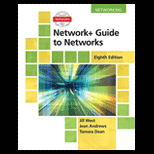
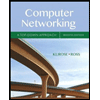
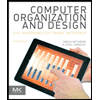
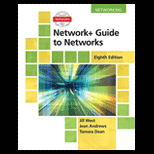
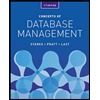
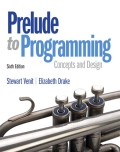
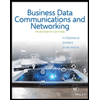