no magic number public class GolfClubMember { // Constants for driving range basket costs public static double SMALL_BASKET_COST = 7.0; public static double MIDIUM_BASKET_COST = 11.0; public static double LARGE_BASKET_COST = 15.0; // Constants for game costs public static double TWILIGHT_GAME_COST = 15.0; public static double REGULAR_GAME_COST = 17.0; // Step 2: Declare two instance variables // name: String // balance: double // Step 3: Write the following constructor // according to the description /** * The constructor has two parameters to initialize * the instance variables. * * @param nameParam the name of this golf club member * @param balanceParam the balance of this golf club member */ // Step 4: Override method toString() according to the description /** * Gets a string representaton for this golf club member in the * following format: * GolfClubMember[Name:Mike,Balance:390.0] * * @return a string containing the name and balance * of this golf club member */ @Override // Step 5: Write method drivingRange() according to the description /** * When you go to a golf driving range, you need to pay to get * a basket of golf balls. * * The method has one parameter for the size of the basket and * it reduces the balance of this golf club member based on * the basket size. * There are three different sizes, "small", "medium", and * "large", but anything other than "small" or "medium" will * be considered "large". * The cost is SMALL_BASKET_COST, MIDIUM_BASKET_COST * and LARGE_BASKET_COST, respectively. * * @param size the size of the basket. */ // Step 6: Write method playingGame() according to the description /** * When you play a golf game, you pay for the game to play. * * The method has one parameter for the type of game to play * and it reduces the balance of this golf club member based * on the game type. * The type is either "regular" or "twilight", but anything * not "regular" will be considered as "twilight". * The cost is REGULAR_GAME_COST and TWILIGHT_GAME_COST, * respectively. * * */ }
no magic number
public class GolfClubMember
{
// Constants for driving range basket costs
public static double SMALL_BASKET_COST = 7.0;
public static double MIDIUM_BASKET_COST = 11.0;
public static double LARGE_BASKET_COST = 15.0;
// Constants for game costs
public static double TWILIGHT_GAME_COST = 15.0;
public static double REGULAR_GAME_COST = 17.0;
// Step 2: Declare two instance variables
// name: String
// balance: double
// Step 3: Write the following constructor
// according to the description
/**
* The constructor has two parameters to initialize
* the instance variables.
*
* @param nameParam the name of this golf club member
* @param balanceParam the balance of this golf club member
*/
// Step 4: Override method toString() according to the description
/**
* Gets a string representaton for this golf club member in the
* following format:
* GolfClubMember[Name:Mike,Balance:390.0]
*
* @return a string containing the name and balance
* of this golf club member
*/
@Override
// Step 5: Write method drivingRange() according to the description
/**
* When you go to a golf driving range, you need to pay to get
* a basket of golf balls.
*
* The method has one parameter for the size of the basket and
* it reduces the balance of this golf club member based on
* the basket size.
* There are three different sizes, "small", "medium", and
* "large", but anything other than "small" or "medium" will
* be considered "large".
* The cost is SMALL_BASKET_COST, MIDIUM_BASKET_COST
* and LARGE_BASKET_COST, respectively.
*
* @param size the size of the basket.
*/
// Step 6: Write method playingGame() according to the description
/**
* When you play a golf game, you pay for the game to play.
*
* The method has one parameter for the type of game to play
* and it reduces the balance of this golf club member based
* on the game type.
* The type is either "regular" or "twilight", but anything
* not "regular" will be considered as "twilight".
* The cost is REGULAR_GAME_COST and TWILIGHT_GAME_COST,
* respectively.
*
*
*/
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

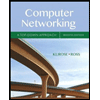
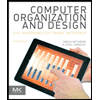
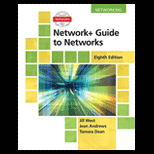
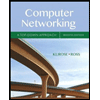
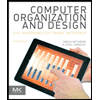
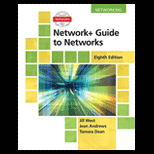
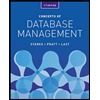
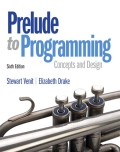
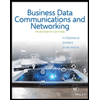