nGenome.java NucleicAcid.java Write a class called DNA to create two strands of DNA in the helix, implemented as an array of objects of type NucleicAcid. Each strand will have the same size. DNA class should be created with following properties LtoRHelix is an array of objects of type NucleicAcid. RtoLHelx is an array of objects of type NucleicAcid. DNA class should be created with following methods: Default Constructor to initialize the prope
Java.
Use the following classes to help you.
-
HumanGenome.java
-
NucleicAcid.java
Write a class called DNA to create two strands of DNA in the helix, implemented as an array of objects of type NucleicAcid. Each strand will have the same size.
DNA class should be created with following properties
-
LtoRHelix is an array of objects of type NucleicAcid.
-
RtoLHelx is an array of objects of type NucleicAcid.
DNA class should be created with following methods:
Default Constructor to initialize the properties to null.
public DNA ()
Overloaded constructor
public DNA (String strand)
that receives a String containing a sequence of Nucleic Acids represented with letters ‘A’, ‘G’, ‘T’ and ‘C’ (For e.g. “ATGCCTAGGATCAG”) and
-
Populates LtoRHelix with corresponding objects using array of NucleicAcids.
-
Populates RtoLHelix with complementary NucleicAcid objects with following criteria:
-
Adenine, A, is always paired with thymine, T.
-
Cytosine, C, is always paired with guanine, G.
-
For e.g.
-
if index value 0 in LtoRHelix contains A then populate RtoHHelix index value 0 should contain T
-
if index value 1 in LtoRHelix contains C then populate RtoHHelix index value 1 should contain G
public void LtoRHelixpopulate(String strand)
For input string ATGCCTAGGATCAG, parse it and for each nucleic acid (like A, T, G or C) create an object of type NucleicAcid in an array of Objects LtoRHelix. This method can be called in the overloaded constructor public DNA (String strand) - String charAT() to parse the characters in the String name strand.
public void print()
In this method, iterate through each index value and print the properties of each nucleic acid in the following array of objects:
-
LtoRHelix and
-
RtoLHelix
public void highestMolarMass()
In this method print the index values and highest MolarMass of Nucleic Acid after traversing the following array of Objects:
-
LtoRHelix and
-
RtoLHelix
public void totalDensity()
In this method print the total Density of all Nucleic Acid within the following array of Objects:
-
LtoRHelix and
-
RtoLHelix
Please don't forget to write a driver class called DNADriver and test all the methods with following Strings (important).
-
AGCCTAGGATCAG
-
AGCCTAGGATCTAGGATCAG
-
AGCCTATAGGATCAG
-
AAAGCCTAGGATAGGATCAG
-
AAAGCCTCTGAGGATAGGATCAG
-------------------------------------------------
(HumanGenome.java)
public class HumanGenome
{
private String genomeName;
private int numberOfGenes;
private int numberOfChromosomes;
private int numberOfCells;
public HumanGenome(String genomeName, int numberOfGenes, int numberOfChromosomes, int numberOfCells)
{
this.genomeName = genomeName;
this.numberOfGenes = numberOfGenes;
this.numberOfChromosomes = numberOfChromosomes;
this.numberOfCells = numberOfCells;
}
public String getGenomeName()
{
return genomeName;
}
public int getNumberOfGenes()
{
return numberOfGenes;
}
public int getNumberOfChromosomes()
{
return numberOfChromosomes;
}
public int getNumberOfCells()
{
return numberOfCells;
}
public void setGenomeName(String genomeName)
{
this.genomeName = genomeName;
}
public void setNumberOfGenes(int numberOfGenes)
{
this.numberOfGenes = numberOfGenes;
}
public void setNumberOfChromosomes(int numberOfChromosomes)
{
this.numberOfChromosomes = numberOfChromosomes;
}
public void setNumberOfCells(int numberOfCells)
{
this.numberOfCells = numberOfCells;
}
public void print()
{
System.out.printf("\nThe Genome Name: %s", getGenomeName());
System.out.printf("\nThe number of genes: %d", getNumberOfGenes());
System.out.printf("\nThe number of chromosomes: %d", getNumberOfChromosomes());
System.out.printf("\nThe number of cells (in trillions): %d", getNumberOfCells());
}
}
-------------------------------------------------
(NucleicAcid.java)
public class NucleicAcid
{
private String chemicalName;
private String chemicalFormula;
private float molarMass;
private float density;
public NucleicAcid(String chemicalName, String chemicalFormula, float molarMass, float density)
{
this.chemicalName = chemicalName;
this.chemicalFormula = chemicalFormula;
this.molarMass = molarMass;
this.density = density;
}
public String getChemicalName()
{
return chemicalName;
}
public String getChemicalFormula()
{
return chemicalFormula;
}
public float getMolarMass()
{
return molarMass;
}
public float getDensity()
{
return density;
}
public void setChemicalName(String chemicalName)
{
this.chemicalName = chemicalName;
}
public void setChemicalFormula(String chemicalFormula)
{
this.chemicalFormula = chemicalFormula;
}
public void setMolarMass(float molarMass)
{
this.molarMass = molarMass;
}
public void setDensity(float density)
{
this.density = density;
}
public void print()
{
System.out.printf("\n%s", chemicalName);
System.out.printf("\n\tChemical Formula - %s", chemicalFormula);
System.out.printf("\n\tMolar Mass - %f g/mol", molarMass);
System.out.printf("\n\tDensity - %f g/cm3 (calculated)", density);
}
}
Cytosine = 11.10 g/mol; 1.55 g/cm3
Adenine = 135.13 g/mol; 1.6 g/cm3
Guanine = 151.13 g/mol; 2.200 g/cm3
Thymine = 126.115 g/mol; 1.223 g/cm3
I need help incorporating the DNADriver class as well as the DNA class. Please don't use answers you've found elsewhere. Thanks.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

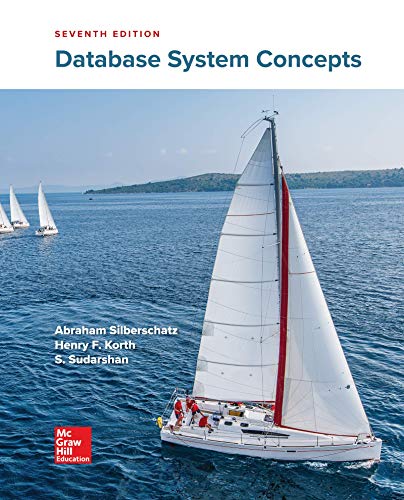
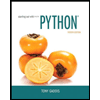
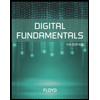
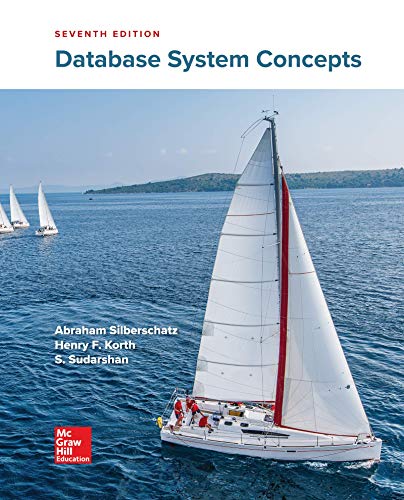
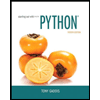
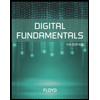
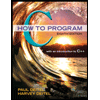
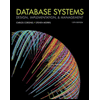
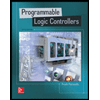