However, it is running on the thread, but in the wrong way. It needs to run continuously followed by how many threads I choose based on the start and end value to perform the calculation. For example, if I choose the start value to be 1 and the end value to be 10 as I choose to run 2 threads, the first thread runs 1 to 5 factorials and the second thread runs 6 to 10 factorial.
I need to add user input to allow the number of threads to be run and a start and an end value to print the lists of calculations.
However, it is running on the thread, but in the wrong way. It needs to run continuously followed by how many threads I choose based on the start and end value to perform the calculation.
For example, if I choose the start value to be 1 and the end value to be 10 as I choose to run 2 threads, the first thread runs 1 to 5 factorials and the second thread runs 6 to 10 factorial.
Or if I want to run 2 threads still, but from 1 to 100 factorial and it will halve into each thread.
For example, if I choose to run 2 threads and input the start number to be 1 and the end number to be 10, it will halve into each thread.
The following output:
(User input)
Enter the number of threads to generate:
2
Enter the first number:1
Enter the second number:10
(Output based on user input)
THREAD 1:
Factorial of 1 is: 1
Factorial of 2 is: 2
Factorial of 3 is: 6
Factorial of 4 is: 24
Factorial of 5 is: 120
THREAD 2:
Factorial of 6 is: 720
Factorial of 7 is: 5040
Factorial of 8 is: 40320
Factorial of 9 is: 362880
Factorial of 10 is: 3628800
Java Program:
As of now, it is only running on the thread but it is repeating the start and end values.
For example, if I choose to run 2 threads and I choose the start number to be 1 and the end number to be 10, the output will be:
Enter the number of threads to generate:
2
Enter the first number:1
Enter the second number:10
Factorial of 1 is: 1
Factorial of 2 is: 2
Factorial of 3 is: 6
Factorial of 4 is: 24
Factorial of 5 is: 120
Factorial of 6 is: 720
Factorial of 7 is: 5040
Factorial of 8 is: 40320
Factorial of 9 is: 362880
Factorial of 10 is: 3628800
Factorial of 1 is: 1
Factorial of 2 is: 2
Factorial of 3 is: 6
Factorial of 4 is: 24
Factorial of 5 is: 120
Factorial of 6 is: 720
Factorial of 7 is: 5040
Factorial of 8 is: 40320
Factorial of 9 is: 362880
Factorial of 10 is: 3628800

Step by step
Solved in 2 steps

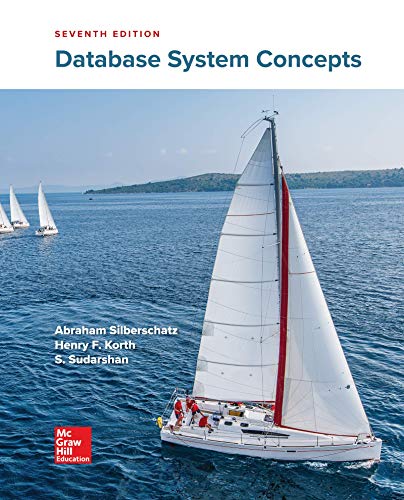
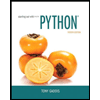
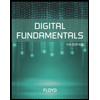
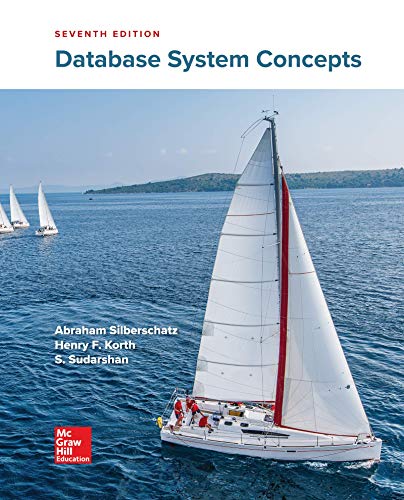
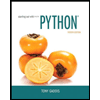
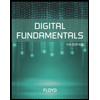
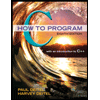
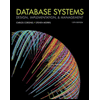
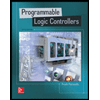