Need help with this python question with to parts. The first part doesn't correspond with the second part and vise versa. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable. 1. AB Verify Your goal is to write a recursive function called in a file ab_verify.py which determines whether the string passed to the function has more a's than (or equal numbers of) b's counted from the start to any given point. The function ab_verify should take two arguments, the first one is the string, the second one will have zero passed in during testing but you can modify it during the recursion. For instance: aab should return True abba should return False aabb should return True since it's equal aabaabaab should return True You may assume that the string is entirely a's and b's. I used the driver code: s = input('Enter a string to test: ') while s != 'quit': print(ab_verify(s, 0)) s = input('Enter a string to test: ') Sample Output linux5[281]% python3 ab_verify.py Enter a string to test: aab True Enter a string to test: abba False Enter a string to test: ababab True Enter a string to test: aabbbbbaaaaabbbabaabababa False Enter a string to test: abb False Enter a string to test: aabaabaab True Enter a string to test: quit 2. (question in images) Spider Webbing Sample Output linux5[109]% python3 spider_web.py Input num_nodes, seed: 5, 101 {'Node 1': ['Node 2', 'Node 3', 'Node 5', 'Node 4'], 'Node 2': ['Node 1', 'Node 4', 'Node 3'], 'Node 3': ['Node 1', 'Node 2', 'Node 4', 'Node 5'], 'Node 4': ['Node 1', 'Node 2', 'Node 3', 'Node 5'], 'Node 5': ['Node 1', 'Node 3', 'Node 4']} ['Node 1', 'Node 2', 'Node 4', 'Node 3', 'Node 5'] linux5[110]% python3 spider_web.py Input num_nodes, seed: 8, 2323 {'Node 1': ['Node 8', 'Node 3', 'Node 5', 'Node 6', 'Node 7', 'Node 4'], 'Node 2': ['Node 6', 'Node 8'], 'Node 3': ['Node 1', 'Node 7', 'Node 6', 'Node 4'], 'Node 4': ['Node 1', 'Node 3', 'Node 8', 'Node 5'], 'Node 5': ['Node 1', 'Node 4', 'Node 8', 'Node 6'], 'Node 6': ['Node 1', 'Node 2', 'Node 3', 'Node 5', 'Node 8', 'Node 7'], 'Node 7': ['Node 1', 'Node 3', 'Node 6'], 'Node 8': ['Node 1', 'Node 2', 'Node 4', 'Node 5', 'Node 6']} ['Node 1', 'Node 8'] linux5[111]% python3 spider_web.py Input num_nodes, seed: 5, 6554 {'Node 1': [], 'Node 2': ['Node 4', 'Node 5'], 'Node 3': [], 'Node 4': ['Node 2'], 'Node 5': ['Node 2']} [] ([] means no path) Coding Standards Constants above your function definitions, outside of the "if __name__ == '__main__':" block. A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance: print(first_creature_name, 'has died in the fight. ') does not involve magic values. However, if my_string == 'EXIT': exit is a magic value since it's being used to compare against variables within your code, so it should be: EXIT_STRING = 'EXIT' ... if my_string == EXIT_STRING: A number is a magic value when it is not 0, 1, and if it is not 2 being used to test parity (even/odd). A number is magic if it is a position in an array, like my_array[23], where we know that at the 23rd position, there is some special data. Instead it should be USERNAME_INDEX = 23 my_array[USERNAME_INDEX] Constants in mathematical formulas can either be made into official constants or kept in a formula. Previously checked coding standards involving: snake_case_variable_names CAPITAL_SNAKE_CASE_CONSTANT_NAMES Use of whitespace (2 before and after a function, 1 for readability.)
Need help with this python question with to parts. The first part doesn't correspond with the second part and vise versa. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable.
1. AB Verify
Your goal is to write a recursive function called in a file ab_verify.py which determines whether the string passed to the function has more a's than (or equal numbers of) b's counted from the start to any given point.
The function ab_verify should take two arguments, the first one is the string, the second one will have zero passed in during testing but you can modify it during the recursion.
For instance:
aab should return True
abba should return False
aabb should return True since it's equal
aabaabaab should return True
You may assume that the string is entirely a's and b's.
I used the driver code:
s = input('Enter a string to test: ')
while s != 'quit':
print(ab_verify(s, 0))
s = input('Enter a string to test: ')
Sample Output
linux5[281]% python3 ab_verify.py Enter a string to test: aab True Enter a string to test: abba False Enter a string to test: ababab True Enter a string to test: aabbbbbaaaaabbbabaabababa False Enter a string to test: abb False Enter a string to test: aabaabaab True Enter a string to test: quit |
2. (question in images)
Spider Webbing Sample Output
linux5[109]% python3 spider_web.py Input num_nodes, seed: 5, 101 {'Node 1': ['Node 2', 'Node 3', 'Node 5', 'Node 4'], 'Node 2': ['Node 1', 'Node 4', 'Node 3'], 'Node 3': ['Node 1', 'Node 2', 'Node 4', 'Node 5'], 'Node 4': ['Node 1', 'Node 2', 'Node 3', 'Node 5'], 'Node 5': ['Node 1', 'Node 3', 'Node 4']} ['Node 1', 'Node 2', 'Node 4', 'Node 3', 'Node 5'] linux5[110]% python3 spider_web.py Input num_nodes, seed: 8, 2323 {'Node 1': ['Node 8', 'Node 3', 'Node 5', 'Node 6', 'Node 7', 'Node 4'], 'Node 2': ['Node 6', 'Node 8'], 'Node 3': ['Node 1', 'Node 7', 'Node 6', 'Node 4'], 'Node 4': ['Node 1', 'Node 3', 'Node 8', 'Node 5'], 'Node 5': ['Node 1', 'Node 4', 'Node 8', 'Node 6'], 'Node 6': ['Node 1', 'Node 2', 'Node 3', 'Node 5', 'Node 8', 'Node 7'], 'Node 7': ['Node 1', 'Node 3', 'Node 6'], 'Node 8': ['Node 1', 'Node 2', 'Node 4', 'Node 5', 'Node 6']} ['Node 1', 'Node 8'] linux5[111]% python3 spider_web.py Input num_nodes, seed: 5, 6554 {'Node 1': [], 'Node 2': ['Node 4', 'Node 5'], 'Node 3': [], 'Node 4': ['Node 2'], 'Node 5': ['Node 2']} [] ([] means no path) |
Coding Standards
- Constants above your function definitions, outside of the "if __name__ == '__main__':" block.
- A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance:
- print(first_creature_name, 'has died in the fight. ') does not involve magic values.
- However, if my_string == 'EXIT': exit is a magic value since it's being used to compare against variables within your code, so it should be:
EXIT_STRING = 'EXIT'
- A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance:
...
if my_string == EXIT_STRING:
- A number is a magic value when it is not 0, 1, and if it is not 2 being used to test parity (even/odd).
- A number is magic if it is a position in an array, like my_array[23], where we know that at the 23rd position, there is some special data. Instead it should be
USERNAME_INDEX = 23
my_array[USERNAME_INDEX]
- Constants in mathematical formulas can either be made into official constants or kept in a formula.
- Previously checked coding standards involving:
- snake_case_variable_names
- CAPITAL_SNAKE_CASE_CONSTANT_NAMES
- Use of whitespace (2 before and after a function, 1 for readability.)
![# Spider Webbing
Create a **recursive** function in a file called `spider_web.py`.
This is going to be a guided problem more than most of the others on this homework. This will teach you some basic pathfinding in a network (graph) for the project. You can use this problem to build your project, so I would recommend working on this problem until you understand it.
Imagine there is a spider web:
![Graph diagram showing nodes and connections, with a highlighted path from node A to node Z, traversing through green nodes.]
Our goal is to find a path (not the best path, but just any path) from A to Z. You see that the green path is not the shortest, but it does let us navigate from start to finish.
**How can we do this?**
First, we need to know A and Z, our starting and ending points. We’ll pass these into our function.
I’m going to use a dictionary to represent this graph. Each node (vertex, circle) will have a name. In this case “A” and “Z” were the names of the nodes, but in the generated maps I’m going to use “Node 1”, “Node 2”, “Node 3”, etc.
Here is an example `web_map`:
```python
web_map = {
'Node 1': ['Node 3', 'Node 2'],
'Node 2': ['Node 1', 'Node 4'],
'Node 3': ['Node 1'],
'Node 4': ['Node 2']
}
```
Node 1 is connected to 2 and 3 for instance, and then also note that Node 3 is connected back to Node 1. Similarly, Node 2 is connected back to Node 1.
Then there’s a connection between Node 2 and Node 4 that also goes both ways. All connections in our web will be bi-directional.
So, in order to find the path from the start to the finish, we should check if there’s a path recursively through any of the nodes connected to wherever we start.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa2a123a1-804d-4397-90a5-64b0c036273c%2F2c34ae81-41c2-4132-a22c-2c54bc50aa44%2F19bk10d_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

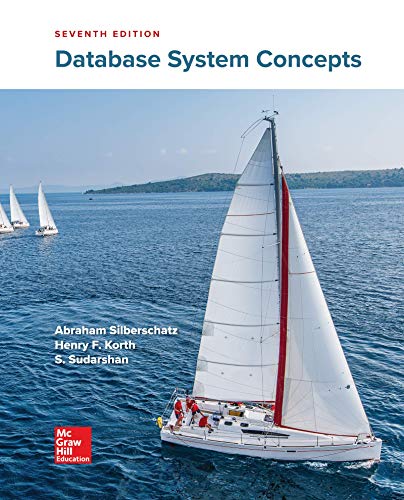
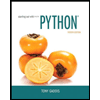
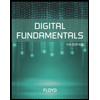
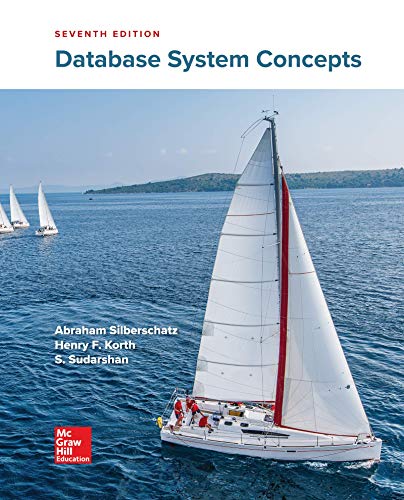
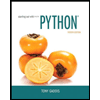
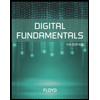
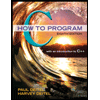
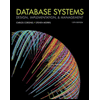
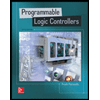