123456789012 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 444 41 42 0-0 0-0 00 from random import randint, choice import time import help instructions = """ARITHMETIC PLEASE You love number crunching don't you? Nice! so do we. Let's crunch some numbers then: First you select a level (i.e. number of operands in the expression) then we give you an arithmetic expression to solve based on your level -\nENJOY!!!\n""" user_data = ["\t\tSession Results: \n'] def get_level () -> int: """Get player level as input from stdin :raises ValueError :return n: user input value def generate_expr (n=2, *new_ops) -> tuple [str, float]: """Generates random arithmetic expression n number of operands :param n: Number of operands in expression :param new_ops: New operator to be ed to the standard operator set i.e. {'*', '/', '//', '', '+', '-') :return tuple: the expression as a string and it's solution as a float def check_user_answer (expr, ans) -> float | str: """Get user answer from stdin for given expression. Print result of comparison to actual value of expression if user answer is equal, different or an invalid number print results accordingly and return user answer :param expr: the expression to be printed :param ans: actual value expression evaluates to :return : user answer must be float or string in the case of Invalid input
Complete the function definitions and main sections in the given template script:
from random import randint, choice
import time
import help
instructions = """ARITHMETIC PLEASE
You love number crunching don't you? Nice! so do we.
Let's crunch some numbers then:
First you select a level (i.e. number of operands in the expression)
then we give you an arithmetic expression to solve based on your level
\nENJOY!!!\n"""
user_data = ['\t\tSession Results:\n']
def get_level() -> int:
"""Get player level as input from stdin
:raises ValueError
:return n: user input value
"""
def generate_expr(n=2, *new_ops) -> tuple[str, float]:
"""Generates random arithmetic expression n number of operands
:param n: Number of operands in expression
:param new_ops: New operator to be added to the standard operator set
i.e. {'*', '/', '//', '%', '+', '-'}
:return tuple: the expression as a string and it's solution as a float
"""
def check_user_answer(expr, ans) -> float | str:
"""Get user answer from stdin for given expression.
Print result of comparison to actual value of expression
if user answer is equal, different or an invalid number
print results accordingly and return user answer
:param expr: the expression to be printed
:param ans: actual value expression evaluates to
:return : user answer must be float or string in the case of Invalid input
"""
def end_session(session, expr, ans, user_ans, file='ARITHP.txt') -> bool:
"""Stores the session information and requests user input to
exit or continue the program.
Writes the stored data to a file (default name ARITHP.txt) if the user
wants to quit and returns True. Else returns False
:param session: integer referencing a count of sessions run
:param expr: expr solved in the session
:param ans: the value the expression evaluates to
:param user_ans: the user's answer for the expression
:param file: name of file to write data to
:return: True if the user wants to quit False otherwise
"""
if __name__ == '__main__':
...

![1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18.
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
mmm
UNTO68
39
40
41
42
43
0-0
00
0-0
from random import randint, choice
import time
import help
instructions = """ARITHMETIC PLEASE
You love number crunching don't you? Nice! so do we.
Let's crunch some numbers then:
First you select a level (i.e. number of operands in the expression)
then we give you an arithmetic expression to solve based on your level
-\nENJOY!!!\n"""
user_data
=
def get_level () -> int:
www
['\t\tSession Results: \n']
"""Get player level as input from stdin
:raises ValueError
:return n: user input value
def generate_expr (n=2, *new_ops) -> tuple [str, float]:
"""Generates random arithmetic expression n number of operands
:param n: Number of operands in expression
:param new_ops: New operator to be added to the standard operator set
i.e. {¹*', '/', '//', '8', '+', ''}
:return tuple: the expression as a string and it's solution as a float
def check_user_answer (expr, ans) -> float | str:
"""Get user answer from stdin for given expression.
11 11 11
Print result of comparison to actual value of expression
if user answer is equal, different or an invalid number
print results accordingly and return user answer
:param expr: the expression to be printed
:param ans: actual value expression evaluates to
:return: user answer must be float or string in the case of Invalid input](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa2fae0d2-f8de-4d86-b278-3d843b434350%2Fe30ed6fa-64b8-461a-aa35-cd1f99de2542%2Fslkf93_processed.jpeg&w=3840&q=75)

Algorithm:
1. Print instructions to explain the game.
2. Initialize the session counter and user data list.
3. Begin loop for each session.
4. Get user level as input from stdin.
5. Generate random arithmetic expressions of the given level.
6. Get the user's answer for the expression.
7. Compare the user's answer to the actual value of the expression.
8. Print results based on the comparison.
9. Store session information and request user input to exit or continue.
10. If the user wants to quit, write stored data to a file and exit the loop.
11. Else, go back to step 4.
12. End.
Step by step
Solved in 4 steps with 5 images

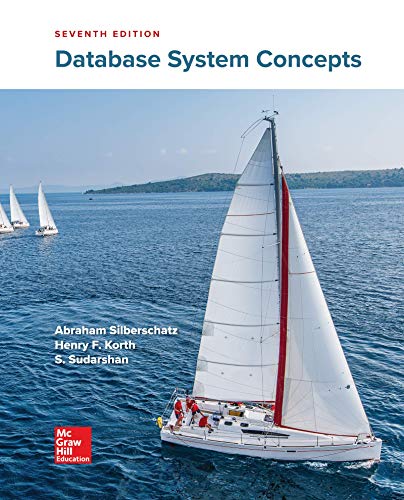
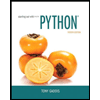
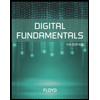
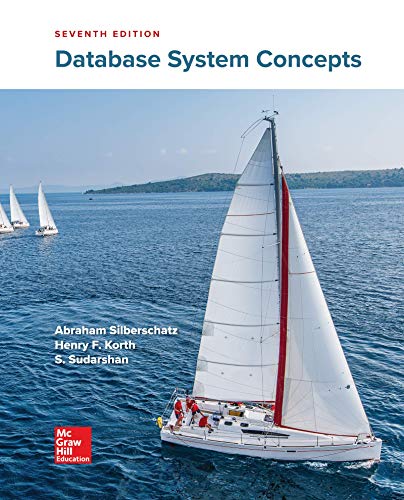
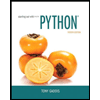
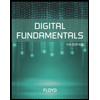
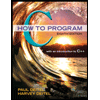
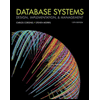
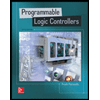