Need help wit
Need help with this C program please!
For this task, you will complete bit_ops.c by implementing the following three bit manipulation batch functions. You will want to use bitwise operations such as and (&), or (|), xor (^), not (~), left shifts (<<), and right shifts (>>).
#include <stdio.h>
#include <stdlib.h>
// Note, the bits are counted from right to left.
// Return the bit states of x within range of [start, end], in which both are inclusive.
// Assume 0 <= start & end <= 31
unsigned * get_bits(unsigned x,
unsigned start,
unsigned end) {
return NULL;
// YOUR CODE HERE
// Returning NULL is a placeholder
// get_bits dynamically allocates an array a and set a[i] = 1 when (i+start)-th bit
// of x is 1, otherwise siet a[i] = 0;
// At last, get_bits returns the address of the array.
}
// Set the bits of x within range of [start, end], in which both are inclusive
// Assume 0 <= start & end <= 31
void set_bits(unsigned * x,
unsigned start,
unsigned end,
unsigned *v) {
// YOUR CODE HERE
// No return value
// v points to an array of at least (end-start+1) unsigned integers.
// if v[i] == 0, then set (i+start)-th bit of x zero, otherwise, set (i+start)-th bit of x one.
}
// Flip the bits of x within range [start, end], in which both are inclusive.
// Assume 0 <= start & end <= 31
void flip_bits(unsigned * x,
unsigned start,
unsigned end) {
// YOUR CODE HERE
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

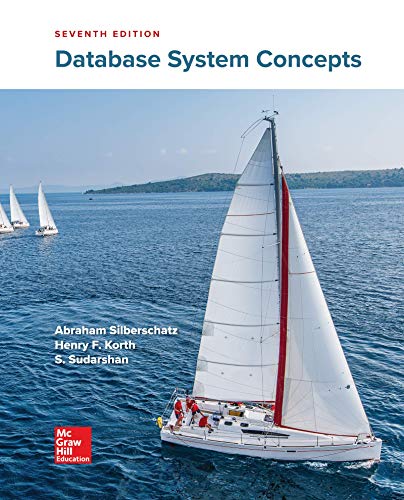
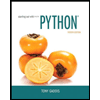
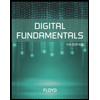
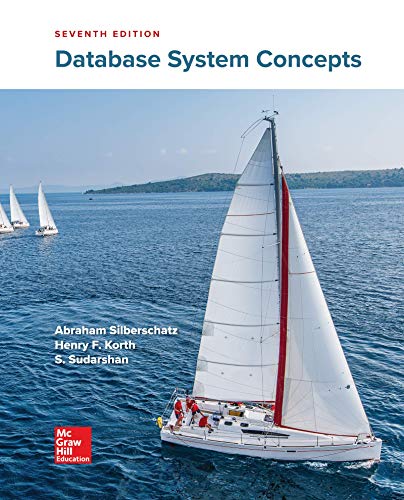
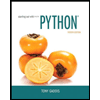
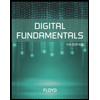
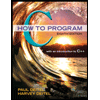
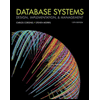
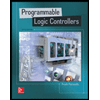