MY CODE print("--------------------------------") print(" UIC CS Track") print("--------------------------------") print() # Ask if the user is a CS major cs_major_answer = input("QUESTION 1\nAre you a CS major? (Yes or No): ").strip().upper() # Initialize earned credit hours to 0 earned_credit_hours = 0 engr100_message = None # Check if the user is a CS major if cs_major_answer == "YES": # Ask if the user has taken ENGR100 print("\nQUESTION 2") engr100_answer = input("Have you taken ENGR100? (Yes or No): ").strip().upper() # Check if the user has taken ENGR100 if engr100_answer == "YES": # Increment earned credit hours by 0 for ENGR100 earned_credit_hours += 0 else: # Display the message if ENGR100 is not taken engr100_message = "Do not forget to take ENGR100!" # Ask about "Level 1" CS classes print("\nQUESTION 3") cs111_answer = input("Have you taken CS111? (Yes or No): ").strip().upper() if cs111_answer == "YES": earned_credit_hours += 3 # Ask about "Level 2" CS classes print("\nQUESTION 4") cs141_answer = input("Have you taken CS141? (Yes or No): ").strip().upper() if cs141_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 5") cs151_answer = input("Have you taken CS151? (Yes or No): ").strip().upper() if cs151_answer == "YES": earned_credit_hours += 3 # Ask about "Level 3" CS classes print("\nQUESTION 6") cs211_answer = input("Have you taken CS211? (Yes or No): ").strip().upper() if cs211_answer == "YES": earned_credit_hours += 3 # Regardless of the answer to CS211, ask question 7 print("\nQUESTION 7") cs251_answer = input("Have you taken CS251? (Yes or No): ").strip().upper() # Ask about CS277, CS377, and CS401 only if CS251 is taken if cs251_answer == "YES": earned_credit_hours += 4 print("Have you taken CS277? (Yes or No): ", end='') cs277_answer = input().strip().upper() if cs277_answer == "YES": earned_credit_hours += 3 if cs277_answer != "NO": # Check if CS277 was not taken print("Have you taken CS377? (Yes or No): ", end='') cs377_answer = input().strip().upper() if cs377_answer == "YES": earned_credit_hours += 3 print("Have you taken CS401? (Yes or No): ", end='') cs401_answer = input().strip().upper() if cs401_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 8") cs261_answer = input("Have you taken CS261? (Yes or No): ").strip().upper() if cs261_answer == "YES": earned_credit_hours += 4 # Display the summary print("\n--------------------------------") print(" Summary") print("--------------------------------") print() if cs_major_answer == "YES": print("You are a CS major!") if engr100_message: print(engr100_message) print() print("Required CS credits earned:", earned_credit_hours, end=".") else: print("Sadly, you are not a CS major.") print() print("\nThank you for using App!") print("Closing app...") ------------------------------------------- Do this : In a file called main.py, implement a program that asks if the user has taken any of the "Level 4" CS classes in addition to the questions from the previous milestone, and then prints a short summary based on the user's replies. The program should have up to 17 input prompts: the string values of the user's answers. Firstly, copy and paste your code from the previous milestone. Your program should continue what you did in the previous milestone by asking up to six additional questions. As you can see in the diagram below, "Level 4" contains CS301, CS341, CS342, CS361, CS362 and CS499. Secondly, here's functionality you need to implement: If the user has not taken all "Level 3" CS courses, the program skips to the summary. Else, your program should ask if the user has taken CS301, CS341 etc. If the user has taken all required CS classes, the program should ask if the user has taken CS499. Similarly to the previous milestone, your program should track how many required credit hours the user has earned so far. In addition, your program should print how many required credit hours is left to take for the user so far. Note that if the user completed all required CS classes, the program should congratulate the user with upcoming graduation.
MY CODE
-------------------------------------------
Do this :
In a file called main.py, implement a
Firstly, copy and paste your code from the previous milestone. Your program should continue what you did in the previous milestone by asking up to six additional questions. As you can see in the diagram below, "Level 4" contains CS301, CS341, CS342, CS361, CS362 and CS499.
Secondly, here's functionality you need to implement:
- If the user has not taken all "Level 3" CS courses, the program skips to the summary. Else, your program should ask if the user has taken CS301, CS341 etc.
- If the user has taken all required CS classes, the program should ask if the user has taken CS499.
Similarly to the previous milestone, your program should track how many required credit hours the user has earned so far. In addition, your program should print how many required credit hours is left to take for the user so far. Note that if the user completed all required CS classes, the program should congratulate the user with upcoming graduation. See the diagram below to see how many credit hours each "Level 4" CS class is worth.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

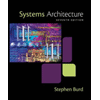
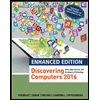
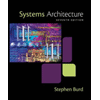
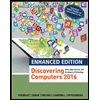
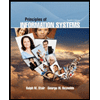
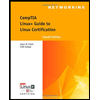