Hands-On Practice 14.9.(SHOWN ABOVE). Add a text box for the user’s city. Ensure that this text box is not empty when the form is submitted. If the city text box is empty, pop up an appropriate alert message and do not submit the form. If the city text box is not empty and the other data is valid, submit the form.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Practice</title>
<meta charset="utf-8">
<style>
input { display: block;
margin-bottom: 1em;
}
label { float: left;
width: 5em;
padding-right: 1em;
text-align: right;
}
input[type="submit"] { margin-left: 7em; }
</style>
<script>
function validateForm() {
if (document.forms[0].userName.value == "" ) {
alert("Name field cannot be empty.");
return false;
} // end if
if (document.forms[0].userAge.value < 18) {
alert("Age is less than 18. You are not an adult.");
return false;
} // end if
alert("Name and Age are valid.");
return true;
} // end function validateForm
</script>
</head>
<body>
<h1>JavaScript Form Handling</h1>
<form method="post"
action="https://webdevbasics.net/scripts/demo.php"
onsubmit="return validateForm();">
<label for="userName">Name:</label>
<input type="text" name="userName" id="userName">
<label for="userAge">Age:</label>
<input type="text" name="userAge" id="userAge">
<input type="submit" value="Send information">
</form>
</body>
</html>
Continue with Hands-On Practice 14.9.(SHOWN ABOVE). Add a text box for the user’s city. Ensure that this text box is not empty when the form is submitted. If the city text box is empty, pop up an appropriate alert message and do not submit the form. If the city text box is not empty and the other data is valid, submit the form.

Step by step
Solved in 3 steps with 4 images

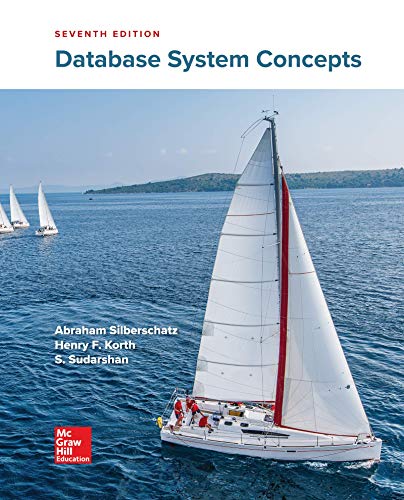
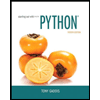
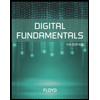
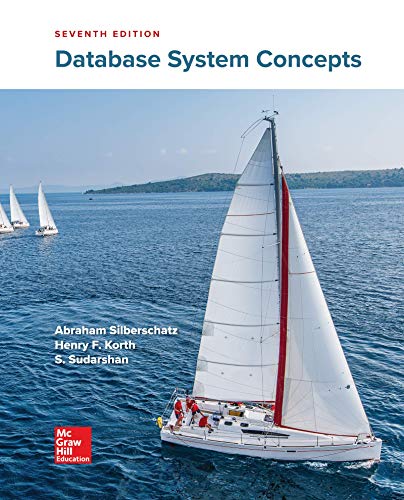
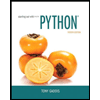
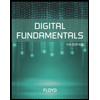
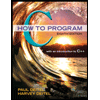
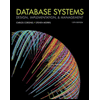
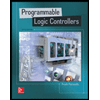