My code is the list of words in the file mywordst.txt is not being added into the array, and instead of going to the list. can someone help me correct it public class MyArrayList2 { public static void main(String[] args) { ArrayList aList = new ArrayList<>(); Scanner fileReader; // here you can give the file name directly and make sure that the file is available in your project directory level fileReader = new Scanner(("myWords.txt")); Scanner keyboardReader = new Scanner(System.in); String inFilePath, line, word; int size = 0; while (fileReader.hasNext()) { line = fileReader.nextLine(); if (line == null) { break; } aList.add(line); } // while not end of file System.out.print("\n\nPlease enter the word you want to search for: "); word = keyboardReader.nextLine(); if (aList.indexOf(word) >= 0) { System.out.println(word + " was found.\n\n"); } else { System.out.println(word + " was not found.\n\n"); } System.out.print("Please enter the word you want to remove: "); word = keyboardReader.nextLine(); int removalCount = 0; while (aList.remove(word)) { removalCount++; } if (removalCount == 0) { System.out.println(word + " was not found, so not removed.\n\n"); } else if (removalCount == 1) { System.out.println("The only instance of " + word + " was removed.\n\n"); } else { System.out.println("All " + removalCount + " instances of " + word + " were removed.\n\n"); } System.out.print("Please enter the word you want to append: "); word = keyboardReader.nextLine(); aList.add(word); System.out.println(word + " was appended.\n\n"); System.out.print("Please enter the word you want to upper case: "); word = keyboardReader.nextLine(); int position = aList.indexOf(word); if (position >= 0) { aList.set(position, word.toUpperCase()); System.out.println(word + " was converted to upper-case.\n\n"); } else { System.out.println(word + " was not found, so not upper-cased.\n\n"); } System.out.println("Here is the final version:\n" + aList); } // method main }
My code is the list of words in the file mywordst.txt is not being added into the array, and instead of going to the list. can someone help me correct it
public class MyArrayList2 {
public static void main(String[] args) {
ArrayList<String> aList = new ArrayList<>();
Scanner fileReader;
// here you can give the file name directly and make sure that the file is available in your project directory level
fileReader = new Scanner(("myWords.txt"));
Scanner keyboardReader = new Scanner(System.in);
String inFilePath, line, word;
int size = 0;
while (fileReader.hasNext()) {
line = fileReader.nextLine();
if (line == null) {
break;
}
aList.add(line);
} // while not end of file
System.out.print("\n\nPlease enter the word you want to search for: ");
word = keyboardReader.nextLine();
if (aList.indexOf(word) >= 0) {
System.out.println(word + " was found.\n\n");
} else {
System.out.println(word + " was not found.\n\n");
}
System.out.print("Please enter the word you want to remove: ");
word = keyboardReader.nextLine();
int removalCount = 0;
while (aList.remove(word)) {
removalCount++;
}
if (removalCount == 0) {
System.out.println(word + " was not found, so not removed.\n\n");
} else if (removalCount == 1) {
System.out.println("The only instance of " + word + " was removed.\n\n");
} else {
System.out.println("All " + removalCount + " instances of " + word + " were removed.\n\n");
}
System.out.print("Please enter the word you want to append: ");
word = keyboardReader.nextLine();
aList.add(word);
System.out.println(word + " was appended.\n\n");
System.out.print("Please enter the word you want to upper case: ");
word = keyboardReader.nextLine();
int position = aList.indexOf(word);
if (position >= 0) {
aList.set(position, word.toUpperCase());
System.out.println(word + " was converted to upper-case.\n\n");
} else {
System.out.println(word + " was not found, so not upper-cased.\n\n");
}
System.out.println("Here is the final version:\n" + aList);
} // method main
}

import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
import java.util.*;
public class MyArrayList2
{
public static void main(String[] args)
{
ArrayList<String> aList = new ArrayList<>();
try
{
File myObj = new File("myWords.txt");
Scanner sc = new Scanner(myObj);
String inFilePath, line, word;
int size = 0;
while (sc.hasNextLine())
{
line = sc.nextLine();
aList.add(line);
} // while not end of file
sc.close();
Scanner sc1 = new Scanner(System.in);
System.out.println("Here is the list: " + aList);
System.out.print("\n\nPlease enter the word you want to search for: ");
word = sc1.next();
if (aList.indexOf(word) >= 0)
{
System.out.println(word + " was found.\n\n");
}
else
{
System.out.println(word + " was not found.\n\n");
}
System.out.print("Please enter the word you want to remove: ");
word = sc1.next();
int removalCount = 0;
while (aList.remove(word))
{
removalCount++;
}
if (removalCount == 0)
{
System.out.println(word + " was not found, so not removed.\n\n");
}
else if (removalCount == 1)
{
System.out.println("The only instance of " + word + " was removed.\n\n");
}
else
{
System.out.println("All " + removalCount + " instances of " + word + " were removed.\n\n");
}
System.out.print("Please enter the word you want to append: ");
word = sc1.next();
aList.add(word);
System.out.println(word + " was appended.\n\n");
System.out.print("Please enter the word you want to upper case: ");
word = sc1.next();
int position = aList.indexOf(word);
if (position >= 0)
{
aList.set(position, word.toUpperCase());
System.out.println(word + " was converted to upper-case.\n\n");
}
else
{
System.out.println(word + " was not found, so not upper-cased.\n\n");
}
System.out.println("Here is the final version:\n" + aList);
}
catch (FileNotFoundException e)
{
System.out.println("An error occurred.");
e.printStackTrace();
}
} // method main
}
Step by step
Solved in 3 steps with 2 images

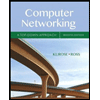
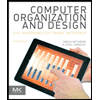
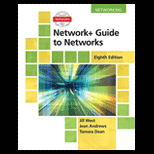
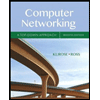
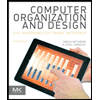
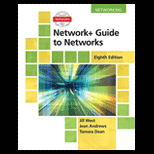
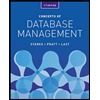
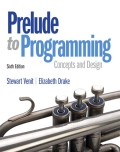
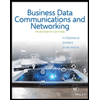