Multiple integers, representing the number of babies, are read from input and inserted into a linked list of TurtleNodes. Find the sum of all the integers in the linked list of TurtleNodes. Ex: If the input is 3 38 18 23, then the output is: 82 import java.util.Scanner; public class TurtleLinkedList { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); TurtleNode headTurtle = null; TurtleNode currTurtle = null; TurtleNode lastTurtle = null; int count; int inputValue; int i; int sum; count = scnr.nextInt(); headTurtle = new TurtleNode(count); lastTurtle = headTurtle; for (i = 0; i < count; ++i) { inputValue = scnr.nextInt(); currTurtle = new TurtleNode(inputValue); lastTurtle.insertAfter(currTurtle); lastTurtle = currTurtle; } /* Your code goes here */ System.out.println(sum); } } class TurtleNode { private int babiesVal; private TurtleNode nextNodeRef; public TurtleNode(int babiesInit) { this.babiesVal = babiesInit; this.nextNodeRef = null; } public void insertAfter(TurtleNode nodeLoc) { TurtleNode tmpNext = null; tmpNext = this.nextNodeRef; this.nextNodeRef = nodeLoc; nodeLoc.nextNodeRef = tmpNext; } public TurtleNode getNext() { return this.nextNodeRef; } public int getNodeData() { return this.babiesVal; } }
Multiple integers, representing the number of babies, are read from input and inserted into a linked list of TurtleNodes. Find the sum of all the integers in the linked list of TurtleNodes.
Ex: If the input is 3 38 18 23, then the output is:
82
import java.util.Scanner;
public class TurtleLinkedList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
TurtleNode headTurtle = null;
TurtleNode currTurtle = null;
TurtleNode lastTurtle = null;
int count;
int inputValue;
int i;
int sum;
count = scnr.nextInt();
headTurtle = new TurtleNode(count);
lastTurtle = headTurtle;
for (i = 0; i < count; ++i) {
inputValue = scnr.nextInt();
currTurtle = new TurtleNode(inputValue);
lastTurtle.insertAfter(currTurtle);
lastTurtle = currTurtle;
}
/* Your code goes here */
System.out.println(sum);
}
}
class TurtleNode {
private int babiesVal;
private TurtleNode nextNodeRef;
public TurtleNode(int babiesInit) {
this.babiesVal = babiesInit;
this.nextNodeRef = null;
}
public void insertAfter(TurtleNode nodeLoc) {
TurtleNode tmpNext = null;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
public TurtleNode getNext() {
return this.nextNodeRef;
}
public int getNodeData() {
return this.babiesVal;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

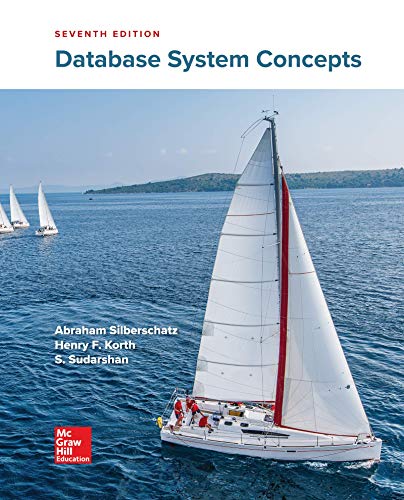
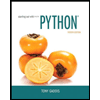
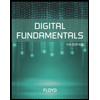
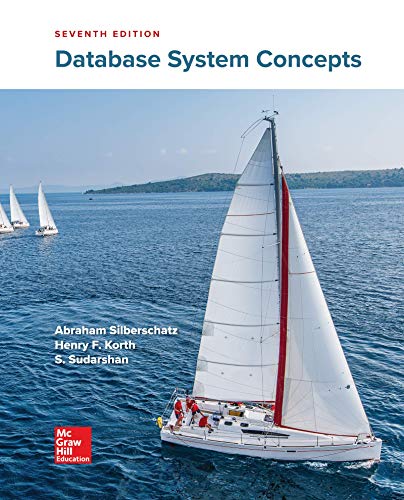
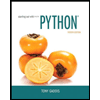
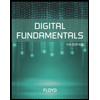
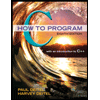
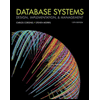
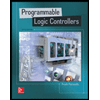