mplement “SoftwareEngineer” and “CheifEngineer” class derived from “Employee” class with necessary properties so that the expected output is generated. Do not change any given code. # Write your codes here. # Do not change the following lines of code. sw1 = SoftwareEngineer("Bob","Software Engineer",40,1200) print('1.------------------------------------') sw1.calculateWeeklyIncome() print('2.------------------------------------') print(sw1) print('3.------------------------------------') sw2 = SoftwareEngineer("Carol","Software Engineer",45,1200,5) print('4.------------------------------------') sw2.calculateWeeklyIncome() print('5.------------------------------------') print(sw2) print('6.------------------------------------') print(Employee.employeeInfo) print('7.====================================') ce1 = ChiefEngineer("John","Chief Engineer",50,1500,7) print('8.------------------------------------') ce1.calculateWeeklyIncome() print('9.------------------------------------') print(ce1) print('10.------------------------------------') ce2 = ChiefEngineer("Brad","Chief Engineer",55,2000) print('11.------------------------------------') ce2.calculateWeeklyIncome() print('12.------------------------------------') print(ce2) print('13.------------------------------------') print(Employee.employeeInfo) Output: Software Engineer,Bob created. 1.------------------------------------ Weekly income of Bob has been calculated 2.------------------------------------ Name: Bob Designation: Software Engineer Work hour per week: 40 hours Per hour income: 1200 Taka Over time:0 hours Weekly income:48000Taka 3.------------------------------------ Software Engineer,Carol created. 4.------------------------------------ Weekly income of Carol has been calculated 5.------------------------------------ Name: Carol Designation: Software Engineer Work hour per week: 45 hours Per hour income: 1200 Taka Over time:5 hours Weekly income:60000Taka 6.------------------------------------ {'Software Engineer': ['Bob', 'Carol']} 7.==================================== Chief Engineer,John created. 8.------------------------------------ Weekly income of John has been calculated 9.------------------------------------ Name: John Designation: Chief Engineer Work hour per week: 50 hours Per hour income: 1500 Over time:7 hours Weekly income:85500 Taka 10.------------------------------------ Chief Engineer,Brad created. 11.------------------------------------ Weekly income of Brad has been calculated 12.------------------------------------ Name: Brad Designation: Chief Engineer Work hour per week: 55 hours Per hour income: 2000 Over time:0 hours Weekly income:110000 Taka 13.------------------------------------ {'Software Engineer': ['Bob', 'Carol'], 'Chief Engineer': ['John', 'Brad']}
mplement “SoftwareEngineer” and “CheifEngineer” class derived from “Employee” class with necessary properties so that the expected output is generated. Do not change any given code.
# Write your codes here.
# Do not change the following lines of code.
sw1 = SoftwareEngineer("Bob","Software Engineer",40,1200)
print('1.------------------------------------')
sw1.calculateWeeklyIncome()
print('2.------------------------------------')
print(sw1)
print('3.------------------------------------')
sw2 = SoftwareEngineer("Carol","Software Engineer",45,1200,5)
print('4.------------------------------------')
sw2.calculateWeeklyIncome()
print('5.------------------------------------')
print(sw2)
print('6.------------------------------------')
print(Employee.employeeInfo)
print('7.====================================')
ce1 = ChiefEngineer("John","Chief Engineer",50,1500,7)
print('8.------------------------------------')
ce1.calculateWeeklyIncome()
print('9.------------------------------------')
print(ce1)
print('10.------------------------------------')
ce2 = ChiefEngineer("Brad","Chief Engineer",55,2000)
print('11.------------------------------------')
ce2.calculateWeeklyIncome()
print('12.------------------------------------')
print(ce2)
print('13.------------------------------------')
print(Employee.employeeInfo)
Output:
Software Engineer,Bob created.
1.------------------------------------
Weekly income of Bob has been calculated
2.------------------------------------
Name: Bob
Designation: Software Engineer
Work hour per week: 40 hours
Per hour income: 1200 Taka
Over time:0 hours
Weekly income:48000Taka
3.------------------------------------
Software Engineer,Carol created.
4.------------------------------------
Weekly income of Carol has been calculated
5.------------------------------------
Name: Carol
Designation: Software Engineer
Work hour per week: 45 hours
Per hour income: 1200 Taka
Over time:5 hours
Weekly income:60000Taka
6.------------------------------------
{'Software Engineer': ['Bob', 'Carol']}
7.====================================
Chief Engineer,John created.
8.------------------------------------
Weekly income of John has been calculated
9.------------------------------------
Name: John
Designation: Chief Engineer
Work hour per week: 50 hours
Per hour income: 1500
Over time:7 hours
Weekly income:85500 Taka
10.------------------------------------
Chief Engineer,Brad created.
11.------------------------------------
Weekly income of Brad has been calculated
12.------------------------------------
Name: Brad
Designation: Chief Engineer
Work hour per week: 55 hours
Per hour income: 2000
Over time:0 hours
Weekly income:110000 Taka
13.------------------------------------
{'Software Engineer': ['Bob', 'Carol'], 'Chief Engineer': ['John', 'Brad']}

Step by step
Solved in 4 steps with 2 images

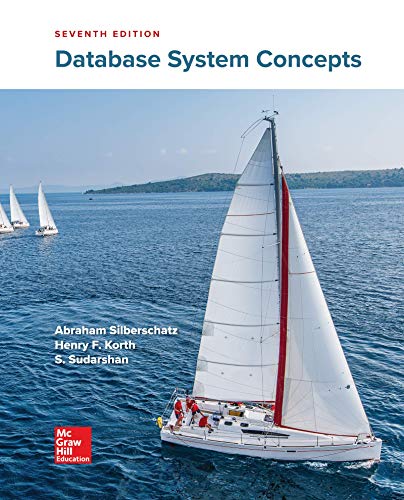
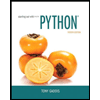
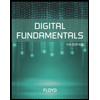
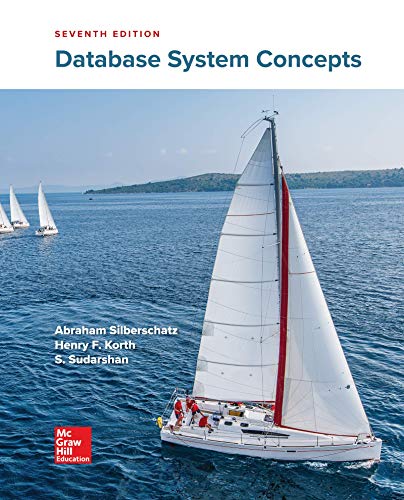
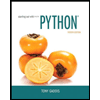
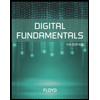
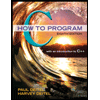
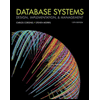
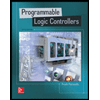