Merge2AscListsRecur that combines the nodes in X-list and Y-list into Z-list such that, after calling the function, Z-list is a sorted list (in non-decreasing order) containing all the nodes initially contained in X-list and Y-list – X-list and Y-list should both be empty after the call.
Merge2AscListsRecur that combines the nodes in X-list and Y-list into Z-list such that, after calling the function, Z-list is a sorted list (in non-decreasing order) containing all the nodes initially contained in X-list and Y-list – X-list and Y-list should both be empty after the call.
The function should: |
► | Have only three parameters (each a pointer-to-Node) and no return value (be a void function). |
► | Not use any global variables or static local variables. |
► | Not use any looping constructs (for, while, do-while, ...). |
► | Be directly (not indirectly) recursive. |
► | Not create any new nodes, destroy any existing nodes or copy/replace the data item of any node. |
► | Not make temporary copies of the data involved using any other storage structures (arrays, stacks, queues, etc.). |
► | Use (if ever needed) no more than a small number of pointers to hold certain link addresses. |
○ | Not make temporary copies of the entire list's link addresses wholesale. |
● | Z-list should be sorted at all times as it "grows" from an empty list to one that contains all the nodes originally contained in X-list and Y-list. |
► | What this means is that you should not, for instance, attempt to first append all the nodes in X-list to Z-list, then append all the nodes in Y-list to Z-list, and finally use a sorting |
#ifndef LLCP_INT_H
#define LLCP_INT_H
#include <iostream>
struct Node
{
int data;
Node *link;
};
int FindListLength(Node* headPtr);
bool IsSortedUp(Node* headPtr);
void InsertAsHead(Node*& headPtr, int value);
void InsertAsTail(Node*& headPtr, int value);
void InsertSortedUp(Node*& headPtr, int value);
bool DelFirstTargetNode(Node*& headPtr, int target);
bool DelNodeBefore1stMatch(Node*& headPtr, int target);
void ShowAll(std::ostream& outs, Node* headPtr);
void FindMinMax(Node* headPtr, int& minValue, int& maxValue);
double FindAverage(Node* headPtr);
void ListClear(Node*& headPtr, int noMsg = 0);
// prototype of Merge2AscListsRecur
#endif



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

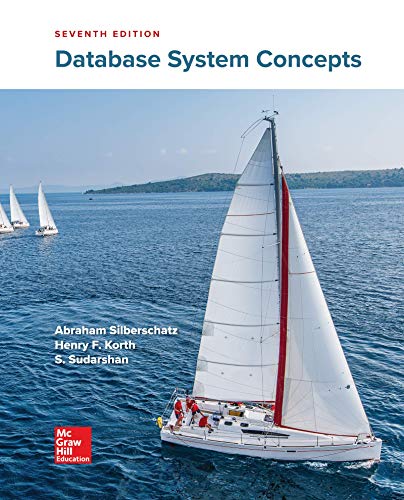
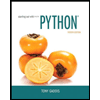
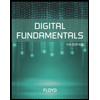
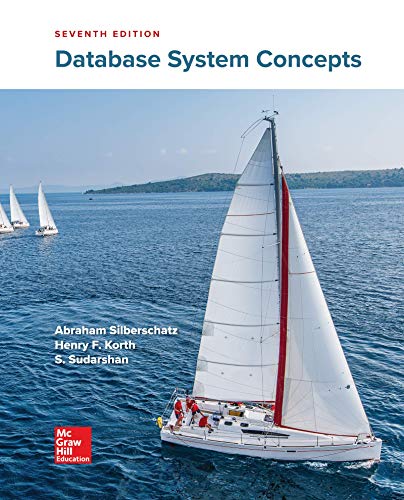
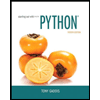
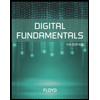
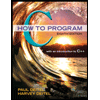
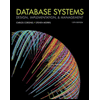
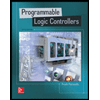