Make the code output sentence starts with a capitalization, and ends with a period. public class Word implements IWord{ private String word; public Word(String word){ this.word = word; } @Override public String getWord(){ return word; } } public class CompoundWord implements IWord { private IWord word1; private IWord word2; public CompoundWord(IWord w1, IWord w2){ word1 = w1; word2 = w2; } @Override public String getWord(){ return word1.getWord() + word2.getWord(); } } import java.util.ArrayList; public class Sentence { private ArrayList words; public Sentence(){ words = new ArrayList(); } public Sentence add(IWord word){ words.add(word); return this; } public String getSentence(){ String result = ""; for(IWord word:words) result += word.getWord()+" "; return result; } } public class Main { public static void main(String[] args) { new Main().go(); } private void go() { IWord w1 = new Word("an"); IWord w2 = new CompoundWord(new CompoundWord(new Word("upper"), new Word("class")), new Word("man")); IWord w3 = new Word("did"); IWord w4 = new Word("the"); IWord w5 = new CompoundWord(new Word("home"), new Word("work")); IWord w6 = new Word("correctly"); Sentence s = new Sentence(); s.add(w1).add(w2).add(w3).add(w4).add(w5).add(w6); System.out.println(s.getSentence()); w1 = new Word("my"); w2 = new CompoundWord(new CompoundWord(new Word("father-"), new Word("in-")), new Word("law")); w3 = new Word("ate"); w4 = new Word("eggs"); w5 = new Word("for"); w6 = new CompoundWord(new Word("break"), new Word("fast")); s = new Sentence(); s.add(w1).add(w2).add(w3).add(w4).add(w5).add(w6); System.out.println(s.getSentence()); } } public interface IWord { public String getWord(); } The output should be An upperclassman did the homework correctly.
Make the code output sentence starts with a capitalization, and ends with a period.
public class Word implements IWord{
private String word;
public Word(String word){
this.word = word;
}
@Override
public String getWord(){
return word;
}
}
public class CompoundWord implements IWord {
private IWord word1;
private IWord word2;
public CompoundWord(IWord w1, IWord w2){
word1 = w1;
word2 = w2;
}
@Override
public String getWord(){
return word1.getWord() + word2.getWord();
}
}
import java.util.ArrayList;
public class Sentence {
private ArrayList<IWord> words;
public Sentence(){
words = new ArrayList<IWord>();
}
public Sentence add(IWord word){
words.add(word);
return this;
}
public String getSentence(){
String result = "";
for(IWord word:words)
result += word.getWord()+" ";
return result;
}
}
public class Main
{
public static void main(String[] args)
{
new Main().go();
}
private void go()
{
IWord w1 = new Word("an");
IWord w2 = new CompoundWord(new CompoundWord(new Word("upper"), new Word("class")), new Word("man"));
IWord w3 = new Word("did");
IWord w4 = new Word("the");
IWord w5 = new CompoundWord(new Word("home"), new Word("work"));
IWord w6 = new Word("correctly");
Sentence s = new Sentence();
s.add(w1).add(w2).add(w3).add(w4).add(w5).add(w6);
System.out.println(s.getSentence());
w1 = new Word("my");
w2 = new CompoundWord(new CompoundWord(new Word("father-"), new Word("in-")), new Word("law"));
w3 = new Word("ate");
w4 = new Word("eggs");
w5 = new Word("for");
w6 = new CompoundWord(new Word("break"), new Word("fast"));
s = new Sentence();
s.add(w1).add(w2).add(w3).add(w4).add(w5).add(w6);
System.out.println(s.getSentence());
}
}
public interface IWord
{
public String getWord();
}
The output should be An upperclassman did the homework correctly.

Step by step
Solved in 2 steps

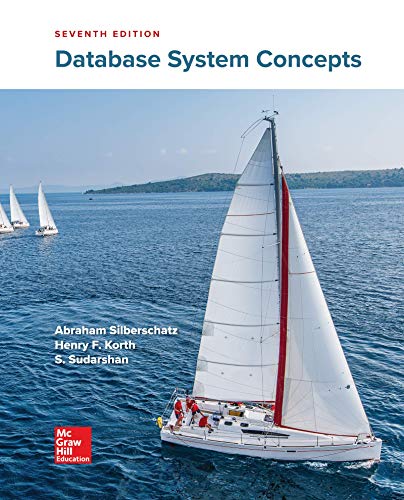
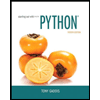
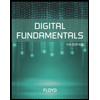
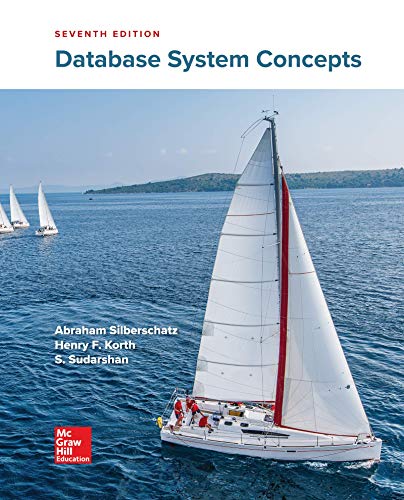
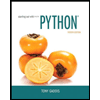
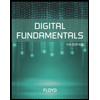
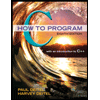
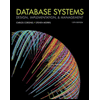
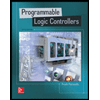