make a different version of this code with the same functions as i can not use my teacher's code
instructions are in photo and links in photos has code which I have pasted to make it easy to copy.
Below is my professor code for Sudoku #2, please make a different version of this code with the same functions as i can not use my teacher's code
Sudoku #2 code
import java.util.*;
import java.io.*;
public class MySudokuBoard {
public final int SIZE = 9;
protected char[][] myBoard;
public MySudokuBoard(String theFile) {
myBoard = new char[SIZE][SIZE];
try {
Scanner file = new Scanner(new File(theFile));
for(int row = 0; row < SIZE; row++) {
String theLine = file.nextLine();
for(int col = 0; col < theLine.length(); col++) {
myBoard[row][col] = theLine.charAt(col);
}
}
} catch(Exception e) {
System.out.println("Something went wrong :(");
e.printStackTrace();
}
}
public boolean isSolved() {
if(!isValid())
return false;
Map<Character,Integer> map = new HashMap<>();
for(char[] row : myBoard) {
for(char cell : row) {
if(map.containsKey(cell))
map.put(cell, map.get(cell) + 1);
else
map.put(cell, 1);
}
}
// info on Collections: https://docs.oracle.com/javase/8/docs/api/?java/util/Collections.html
return map.keySet().size() == 9 && Collections.frequency(map.values(),9) == 9;
}
public boolean isValid() {
// checks for bad data
for(char[] row : myBoard)
for(char cell : row)
if(cell != '.' && (cell < '1' || cell > '9'))
return false;
// checks for row/col violations
for(int r = 0; r < myBoard.length; r++) {
Set<Character> trackingRow = new HashSet<>();
Set<Character> trackingCol = new HashSet<>();
for(int c = 0; c < myBoard[r].length; c++) {
// check for row violation
if(trackingRow.contains(myBoard[r][c]))
return false;
else if(myBoard[r][c] != '.')
trackingRow.add(myBoard[r][c]);
// check for col violation
if(trackingCol.contains(myBoard[c][r]))
return false;
else if(myBoard[c][r] != '.')
trackingCol.add(myBoard[c][r]);
}
}
// check for mini-squares
for(int square = 1; square <= 9; square++) {
char[][] mini = miniSquare(square);
Set<Character> trackingMini = new HashSet<>();
for(int r = 0; r < 3; r++)
for(int c = 0; c < 3; c++)
// check for mini violation
if(trackingMini.contains(mini[r][c]))
return false;
else if(mini[r][c] != '.')
trackingMini.add(mini[r][c]);
}
// if there weren't any violations above...
return true;
}
private char[][] miniSquare(int spot) {
char[][] mini = new char[3][3];
for(int r = 0; r < 3; r++) {
for(int c = 0; c < 3; c++) {
// whoa - wild! This took me a solid hour to figure out.
// This translates between the "spot" in the 9x9 Sudoku board
// and a new mini square of 3x3
mini[r][c] = myBoard[(spot - 1) / 3 * 3 + r][(spot - 1) % 3 * 3 + c];
}
}
return mini;
}
public String toString() {
String result = "My Board:\n\n";
for(int row = 0; row < SIZE; row++) {
for(int col = 0; col < SIZE; col++) {
result += (myBoard[row][col]);
}
result += ("\n");
}
return result;
}
}
Part 3: Test your Sudoku Solver functionality
To test that everything works, run my SudokuSolverEngine.java using these provided board states: very-fast-solve.sdk and fast-solve.sdk I am giving you these test boards because it turns out that recursive backtracking is both memory in-efficient and slow. This means that if you try to solve() some of the boards from Sudoku #2, the solve() method might run for a very long time. (the code is below)
SudokuSolverEngine.java code
public class SudokuSolverEngine {
public static void main(String[] args) {
// Here I have called my class `MySudokuBoard` if you named your class
// differently, modify the line below to use your own class name
MySudokuBoard board = new MySudokuBoard("boards/very-fast-solve.sdk");
System.out.println("Initial board");
System.out.println(board);
System.out.println();
System.out.print("Solving board...");
long start = System.currentTimeMillis();
board.solve();
long stop = System.currentTimeMillis();
System.out.printf("SOLVED in %.3f seconds.\n", ((stop-start)/1000.0));
System.out.println();
System.out.println(board);
}
}
fast-solve.sdk
827154396
965.27148
3416.9752
.........
.........
61897.435
786235.14
1547968.3
23984....
very-fast-solve.sdk
.34678912
.72195348
198342567
..9.61423
.26853791
.13924.56
.61537284
.8.419635
345.86179
You need to add two things to the SolverEngine.
- Before trying to solve the board, check if the board is in an invalid state. If it is, print a message to the screen that says that the board cannot be solved.
- You can test with the any of the rules violating boards from Sudoku #2
- Before trying to solve the board, check if the board is already solved. If it is, print a message to the screen that says that the board is already solved.
- You can test with the valid-complete.sdk board from Sudoku #2
What to Submit
- Your modified board class
- The SudokuSolverEngine with your modifications
Let me know if you need more information!



Please check the step 2 & 3 for solution
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

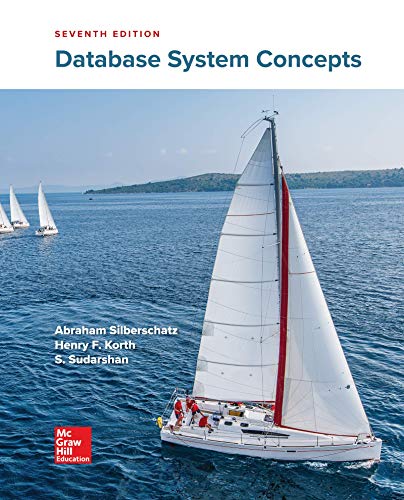
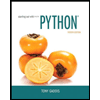
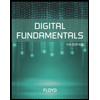
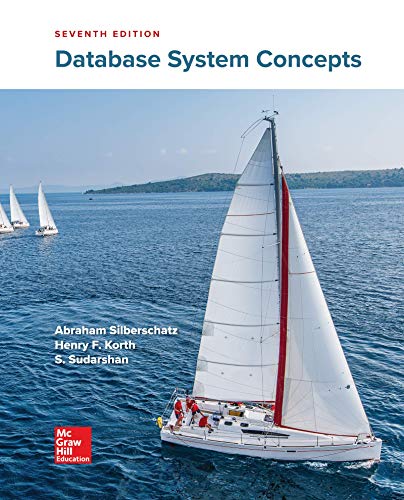
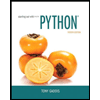
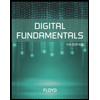
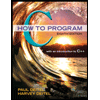
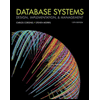
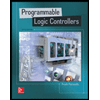