Lo Shu Magic Square) The Lo Shu Magic Square is a grid with 3 rows and 3 columns. The Lo Shu Magic Square has the following properties: 1. The grid contains the numbers 1 through 9 exactly. 2. The sum of each row, each column, and each diagonal all add up to the same number. in a program you can simulate a magic square using a two-dimensional array. Write a function that accepts a two-dimensional array as an argument, and determines whether the array is a Lo Shu Magic Square. Test the function in a program. 15 9 2 3 7 + 15 8 1 6 +15 15 15 15 15 • Required function header: const int COLS - 3; void showArray(const int array[[COLS], int rows); //display 2-D array content bool checkLoShuSquare(const int array[][COLS], int rows); // check if the 2-D is a Lo Shu Square Hint
Lo Shu Magic Square) The Lo Shu Magic Square is a grid with 3 rows and 3 columns. The Lo Shu Magic Square has the following properties: 1. The grid contains the numbers 1 through 9 exactly. 2. The sum of each row, each column, and each diagonal all add up to the same number. in a program you can simulate a magic square using a two-dimensional array. Write a function that accepts a two-dimensional array as an argument, and determines whether the array is a Lo Shu Magic Square. Test the function in a program. 15 9 2 3 7 + 15 8 1 6 +15 15 15 15 15 • Required function header: const int COLS - 3; void showArray(const int array[[COLS], int rows); //display 2-D array content bool checkLoShuSquare(const int array[][COLS], int rows); // check if the 2-D is a Lo Shu Square Hint
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

**Diagram Explanation:**
- The 3x3 grid contains the numbers:
4 9 2
3 5 7
8 1 6
- Arrows show that the sum of each row, column, and diagonal equals 15.
**Required Function Header:**
```c
const int COLS = 3;
void showArray(const int array[][COLS], int rows); // Display 2-D array content
bool checkLoShuSquare(const int array[][COLS], int rows); // Check if the 2-D is a Lo Shu Square
```
**Hint:**
- Find the sum of the first row. If the sum of any other row or column is not equal to the sum of the first row, return false from the `checkLoShuSquare()` function.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e7c4d7d-ede9-4b81-8e82-325ff8e9546c%2F9dd526d9-d7a1-4c5f-b8e4-69d4f2f24a52%2Fid1jya_processed.png&w=3840&q=75)
Transcribed Image Text:**Lo Shu Magic Square**
The Lo Shu Magic Square is a grid with 3 rows and 3 columns. The Lo Shu Magic Square has the following properties:
1. The grid contains the numbers 1 through 9 exactly.
2. The sum of each row, each column, and each diagonal all add up to the same number.
In a program, you can simulate a magic square using a two-dimensional array. Write a function that accepts a two-dimensional array as an argument and determines whether the array is a Lo Shu Magic Square. Test the function in a program.

**Diagram Explanation:**
- The 3x3 grid contains the numbers:
4 9 2
3 5 7
8 1 6
- Arrows show that the sum of each row, column, and diagonal equals 15.
**Required Function Header:**
```c
const int COLS = 3;
void showArray(const int array[][COLS], int rows); // Display 2-D array content
bool checkLoShuSquare(const int array[][COLS], int rows); // Check if the 2-D is a Lo Shu Square
```
**Hint:**
- Find the sum of the first row. If the sum of any other row or column is not equal to the sum of the first row, return false from the `checkLoShuSquare()` function.
![```cpp
bool validateArray(const int array[][COLS], int rows)
```
### Function Overview
Write a function to validate that a 2D array contains the numbers 1 through 9 exactly once, in each case.
---
### Sample Squares (2D Array) for Testing Purposes:
```cpp
int LoShu[3][COLS] = {{4, 9, 2}, {3, 5, 7}, {8, 1, 6}};
int square3[3][COLS] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int square2[3][COLS] = {{1, 4, 7}, {2, 5, 8}, {3, 6, 9}};
int squareTest[3][COLS] = {0}; // test the extra credit assignment
```
---
### Sample Output:
```
**Test the First Square**
4 9 2
3 5 7
8 1 6
It is a Lo Shu Magic Square!
**Test the Second Square**
1 2 3
4 5 6
7 8 9
It is NOT a Lo Shu Magic Square!
**Test the Last Square**
1 4 7
2 5 8
3 6 9
It is NOT a Lo Shu Magic Square!
**Test the Invalid Square**
0 0 0
0 0 0
0 0 0
It is NOT a 3 x 3 array contained the numbers 1–9 exactly.
It is NOT a Lo Shu Magic Square!
```
---
### Requirements:
1. **Use nested for-loops** to find the sum of each row and column.
2. **DON'T** compare each sum == 15. **DON'T** assume the desired total is 15.
3. **Display the programmer info** at the beginning of the output.
4. **Include the function prototypes before the main()** function.
5. **All function definitions should be after the main()** function.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e7c4d7d-ede9-4b81-8e82-325ff8e9546c%2F9dd526d9-d7a1-4c5f-b8e4-69d4f2f24a52%2Fay8wlyf_processed.png&w=3840&q=75)
Transcribed Image Text:```cpp
bool validateArray(const int array[][COLS], int rows)
```
### Function Overview
Write a function to validate that a 2D array contains the numbers 1 through 9 exactly once, in each case.
---
### Sample Squares (2D Array) for Testing Purposes:
```cpp
int LoShu[3][COLS] = {{4, 9, 2}, {3, 5, 7}, {8, 1, 6}};
int square3[3][COLS] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int square2[3][COLS] = {{1, 4, 7}, {2, 5, 8}, {3, 6, 9}};
int squareTest[3][COLS] = {0}; // test the extra credit assignment
```
---
### Sample Output:
```
**Test the First Square**
4 9 2
3 5 7
8 1 6
It is a Lo Shu Magic Square!
**Test the Second Square**
1 2 3
4 5 6
7 8 9
It is NOT a Lo Shu Magic Square!
**Test the Last Square**
1 4 7
2 5 8
3 6 9
It is NOT a Lo Shu Magic Square!
**Test the Invalid Square**
0 0 0
0 0 0
0 0 0
It is NOT a 3 x 3 array contained the numbers 1–9 exactly.
It is NOT a Lo Shu Magic Square!
```
---
### Requirements:
1. **Use nested for-loops** to find the sum of each row and column.
2. **DON'T** compare each sum == 15. **DON'T** assume the desired total is 15.
3. **Display the programmer info** at the beginning of the output.
4. **Include the function prototypes before the main()** function.
5. **All function definitions should be after the main()** function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
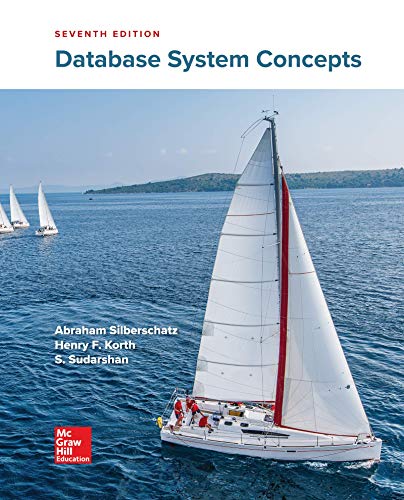
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
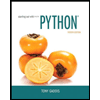
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
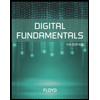
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
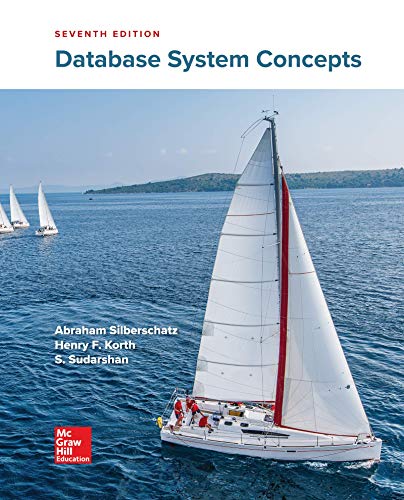
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
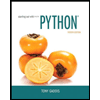
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
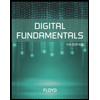
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
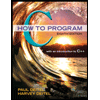
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
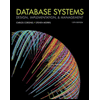
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
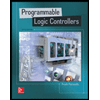
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education