lease due in c++ A sample run is as follows (be sure to format your output): BOOK INFORMATION In Search of Lost Time Marcel Proust 1913 $17.99 Ulysses James Joyce 1904 $21.95 Don Quixote Miguel de Cervantes 1605 $21.49 One Hundred Years of Solitude Gabriel Garcia Marquez 1982 $28.09 The Great Gatsby F. Scott Fitzgerald 1925 $10.99 This is files from the picture: //Book.h - The Book class/structure #ifndef _BOOK_H_ #define _BOOK_H_ //GIVEN: some useful constants const int MAX_STRING = 64; const int MAX_STREAM = 128; const int NUM_BOOKS = 5;//Let's read only five books from the file //TODO: Create a class or structure called Book // If you create a class, you can make all variables public #endif _BOOK_H_ This is Book.cpp : [1:27 a.m., 2023-03-13] Amjd: //Book.cpp - function definitions for the Book class #include "Book.h" //Create a function to extract a string from the stream. //It will be called four times from main() to extract the //NAME, AUTHOR, YEAR OF PUBLICATION, and PRICE. //Use below as a guide if you wish //Extracting NAME: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | //dataBeginPtr dataEndPtr //Extracting AUTHOR: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | // dataBeginPtr dataEndPtr //Extracting YEAR OF PUBLICATION: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | // dataBeginPtr dataEndPtr //Extracting PRICE: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | // dataBeginPtr dataEndPtr this is BookMain.cpp from the picture: //BookMain.cpp - main function for Book #define _CRT_SECURE_NO_WARNINGS #include //TODO: Add any other required header files #include "Book.h" int main(int argc, char* argv[]) { int retVal = 0; if (argc != 2) { printf("Usage: BookMain.exe Book.txt"); return -1; } FILE* fp = fopen(argv[1], "r"); //TODO: Declare an array of NUM_BOOKS objects of type Book for (int i = 0; i < NUM_BOOKS; ++i) { char bookData[MAX_STREAM] = { 0 }; fscanf_s(fp, "%[^\n]s", bookData, MAX_STREAM);//Read an entire line from the file and store in bookData while (fgetc(fp) != '\n');//clear out the carriage return //NAME;AUTHOR;YEAR;PRICE; //TODO: Call the extraction function four times too extract the NAME, AUTHOR, YEAR, and PRICE using // the following pointers. Store the information into the array of Book objects one at a time: char* leftPtr = bookData;//&bookData[0] char* rightPtr = nullptr;//C++ equivalent of NULL } fclose(fp); //TODO: print out all the information for all the books return 0; }
lease due in c++ A sample run is as follows (be sure to format your output): BOOK INFORMATION In Search of Lost Time Marcel Proust 1913 $17.99 Ulysses James Joyce 1904 $21.95 Don Quixote Miguel de Cervantes 1605 $21.49 One Hundred Years of Solitude Gabriel Garcia Marquez 1982 $28.09 The Great Gatsby F. Scott Fitzgerald 1925 $10.99 This is files from the picture: //Book.h - The Book class/structure #ifndef _BOOK_H_ #define _BOOK_H_ //GIVEN: some useful constants const int MAX_STRING = 64; const int MAX_STREAM = 128; const int NUM_BOOKS = 5;//Let's read only five books from the file //TODO: Create a class or structure called Book // If you create a class, you can make all variables public #endif _BOOK_H_ This is Book.cpp : [1:27 a.m., 2023-03-13] Amjd: //Book.cpp - function definitions for the Book class #include "Book.h" //Create a function to extract a string from the stream. //It will be called four times from main() to extract the //NAME, AUTHOR, YEAR OF PUBLICATION, and PRICE. //Use below as a guide if you wish //Extracting NAME: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | //dataBeginPtr dataEndPtr //Extracting AUTHOR: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | // dataBeginPtr dataEndPtr //Extracting YEAR OF PUBLICATION: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | // dataBeginPtr dataEndPtr //Extracting PRICE: // NAME;AUTHOR;YEAR;PRICE; // ^ ^ // | | // dataBeginPtr dataEndPtr this is BookMain.cpp from the picture: //BookMain.cpp - main function for Book #define _CRT_SECURE_NO_WARNINGS #include //TODO: Add any other required header files #include "Book.h" int main(int argc, char* argv[]) { int retVal = 0; if (argc != 2) { printf("Usage: BookMain.exe Book.txt"); return -1; } FILE* fp = fopen(argv[1], "r"); //TODO: Declare an array of NUM_BOOKS objects of type Book for (int i = 0; i < NUM_BOOKS; ++i) { char bookData[MAX_STREAM] = { 0 }; fscanf_s(fp, "%[^\n]s", bookData, MAX_STREAM);//Read an entire line from the file and store in bookData while (fgetc(fp) != '\n');//clear out the carriage return //NAME;AUTHOR;YEAR;PRICE; //TODO: Call the extraction function four times too extract the NAME, AUTHOR, YEAR, and PRICE using // the following pointers. Store the information into the array of Book objects one at a time: char* leftPtr = bookData;//&bookData[0] char* rightPtr = nullptr;//C++ equivalent of NULL } fclose(fp); //TODO: print out all the information for all the books return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please due in c++
A sample run is as follows (be sure to format your output):
BOOK INFORMATION
In Search of Lost Time Marcel Proust 1913 $17.99
Ulysses James Joyce 1904 $21.95
Don Quixote Miguel de Cervantes 1605 $21.49
One Hundred Years of Solitude Gabriel Garcia Marquez 1982 $28.09
The Great Gatsby F. Scott Fitzgerald 1925 $10.99
This is files from the picture:
//Book.h - The Book class/structure
#ifndef _BOOK_H_
#define _BOOK_H_
//GIVEN: some useful constants
const int MAX_STRING = 64;
const int MAX_STREAM = 128;
const int NUM_BOOKS = 5;//Let's read only five books from the file
//TODO: Create a class or structure called Book
// If you create a class, you can make all variables public
#endif _BOOK_H_
This is Book.cpp :
[1:27 a.m., 2023-03-13] Amjd: //Book.cpp - function definitions for the Book class
#include "Book.h"
//Create a function to extract a string from the stream.
//It will be called four times from main() to extract the
//NAME, AUTHOR, YEAR OF PUBLICATION, and PRICE.
//Use below as a guide if you wish
//Extracting NAME:
// NAME;AUTHOR;YEAR;PRICE;
// ^ ^
// | |
//dataBeginPtr dataEndPtr
//Extracting AUTHOR:
// NAME;AUTHOR;YEAR;PRICE;
// ^ ^
// | |
// dataBeginPtr dataEndPtr
//Extracting YEAR OF PUBLICATION:
// NAME;AUTHOR;YEAR;PRICE;
// ^ ^
// | |
// dataBeginPtr dataEndPtr
//Extracting PRICE:
// NAME;AUTHOR;YEAR;PRICE;
// ^ ^
// | |
// dataBeginPtr dataEndPtr
this is BookMain.cpp from the picture:
//BookMain.cpp - main function for Book
#define _CRT_SECURE_NO_WARNINGS
#include
//TODO: Add any other required header files
#include "Book.h"
int main(int argc, char* argv[]) {
int retVal = 0;
if (argc != 2) {
printf("Usage: BookMain.exe Book.txt");
return -1;
}
FILE* fp = fopen(argv[1], "r");
//TODO: Declare an array of NUM_BOOKS objects of type Book
for (int i = 0; i < NUM_BOOKS; ++i) {
char bookData[MAX_STREAM] = { 0 };
fscanf_s(fp, "%[^\n]s", bookData, MAX_STREAM);//Read an entire line from the file and store in bookData
while (fgetc(fp) != '\n');//clear out the carriage return
//NAME;AUTHOR;YEAR;PRICE;
//TODO: Call the extraction function four times too extract the NAME, AUTHOR, YEAR, and PRICE using
// the following pointers. Store the information into the array of Book objects one at a time:
char* leftPtr = bookData;//&bookData[0]
char* rightPtr = nullptr;//C++ equivalent of NULL
}
fclose(fp);
//TODO: print out all the information for all the books
return 0;
}

Transcribed Image Text:(Global Scope)
>
release Property Pages
Configuration Active(Debug)
Configuration Properties
General
Advanced,
Debugging
Ve Directories
D C/C++
▷ Linker
Manifest Tool
DXML Document Generator
Browse Information
Build Events
Custom Build Step
Code Analysis
.
Platform: Active(x64)
Debugger to launch
Local Windows Debugger
Command
Command Arguments
Working Directory
Attach
Debugger Type
Environment
●
Merge Environment
SQL Debugging
Amp Default Accelerator
nuctper;//C++ equivalent of NULL
Command
The debug command to execute.
S(TargetPath)
Book.txt
$(Project Dir)
No
Auto
Yes
No
WARP software accelerator
OK
In Search of Lost Time
Ulysses
Don Quixote
One Hundred Years of Solitude
The Great Gatsby
Cancel
This is the
sample
?
Configuration Manager.
X
Apply
A sample run is as follows (be sure to format your output):
BOOK INFORMATION
-+
ar
ķ
Solution Explorer
264438
Search Solution Explorer (Ctr)
Solution release' (1 of 1 project
Complete the Code
Three files have been provided for you: Book.h, Book.cpp and BookMain.cpp.
Book.h has some useful constants. You have to create a class or structure of
type Book.
release)
References
> External Dependencies
Header Files
Bookh
Resource Files
Source Files
Book.cpp
BookMain.cpp
>
Book.cpp has some useful suggestions on how to extract a string from a stream
of data. In extracting a string, you are not allowed to use any of the C++ libraries
in doing so. You are to use pointers only.
. BookMain.cpp has been started for you. Please complete the TODO sections.
Solution Explorer Git Changes
Properties
release Project Properties
Misc
(Name)
Project Depende
Project File CSenecaYear 20
Root Namespace release
Unrelated to this lab is some sample code on how to handle invalid keyboard entries for
an integer and double float can be seen at GetValue.c.
Sample Run
release
Marcel Proust
James Joyce
Miguel de Cervantes
Gabriel Garcia Marquez
1913 $17.99
1904 $21.95
1605 $21.49
1982 $28.09
F. Scott Fitzgerald 1925 $10.99
![This lab will test your ability to parse data into a structure.
The Book Information
A text file contains information about a set of books. The token for separating
information for each book is the colon ;. There is a separate line for each book entry.
Each book in this text file has a name, an author, a year of publication and a price. Your
mission is to extract this information from the file and store it somewhere in your
program. Once you have completed the extraction, you are to print out information for
each of the books.
Data Format
Information for each book is stored as follows:
NAME; AUTHOR; YEAR; PRICE;
Note that the book's name and author might consist of many strings. The text file
containing all book information can be found at Book.txt.
The data stored there is:
In Search of Lost Time; Marcel Proust;1913;17.99;
Ulysses; James Joyce; 1904;21.95;
Don Quixote;Miguel de Cervantes;1605;21.49;
One Hundred Years of Solitude;Gabriel Garcia Marquez; 1982;28.09;
The Great Gatsby;F. Scott Fitzgerald; 1925;10.99;
Moby Dick;Herman Melville; 1851;6.23
Command Line Arguments
Book.txt must be passed through to your program by command line. Let's say your
executable is called BookMain.exe, then BookMain.exe will be invoked from the
command line as follows:
BookMain.exe Book.txt
Command line arguments are passed through to your program through the main
function as follows:
int main(int argc, char* argv[])
argc is the argument count (which in this case is 2), and argv[] is an array of strings
representing the arguments (in this case argv[0]="BookMain.exe" and
argv[1]="Book.txt").
In Visual Studio, you can set the command line arguments through the project's
properties as follows:
Click on the project name.
Right click on Properties.
Click on Configuration Properties, then click on Debugging.
Look for Command Arguments and enter in the command argument (which in
this case is Book.txt)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F51617a83-5d5f-4556-8af0-d966d758226f%2F29aaefb6-19c7-4a94-9527-9750d84335cc%2Fa1wjxxm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:This lab will test your ability to parse data into a structure.
The Book Information
A text file contains information about a set of books. The token for separating
information for each book is the colon ;. There is a separate line for each book entry.
Each book in this text file has a name, an author, a year of publication and a price. Your
mission is to extract this information from the file and store it somewhere in your
program. Once you have completed the extraction, you are to print out information for
each of the books.
Data Format
Information for each book is stored as follows:
NAME; AUTHOR; YEAR; PRICE;
Note that the book's name and author might consist of many strings. The text file
containing all book information can be found at Book.txt.
The data stored there is:
In Search of Lost Time; Marcel Proust;1913;17.99;
Ulysses; James Joyce; 1904;21.95;
Don Quixote;Miguel de Cervantes;1605;21.49;
One Hundred Years of Solitude;Gabriel Garcia Marquez; 1982;28.09;
The Great Gatsby;F. Scott Fitzgerald; 1925;10.99;
Moby Dick;Herman Melville; 1851;6.23
Command Line Arguments
Book.txt must be passed through to your program by command line. Let's say your
executable is called BookMain.exe, then BookMain.exe will be invoked from the
command line as follows:
BookMain.exe Book.txt
Command line arguments are passed through to your program through the main
function as follows:
int main(int argc, char* argv[])
argc is the argument count (which in this case is 2), and argv[] is an array of strings
representing the arguments (in this case argv[0]="BookMain.exe" and
argv[1]="Book.txt").
In Visual Studio, you can set the command line arguments through the project's
properties as follows:
Click on the project name.
Right click on Properties.
Click on Configuration Properties, then click on Debugging.
Look for Command Arguments and enter in the command argument (which in
this case is Book.txt)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
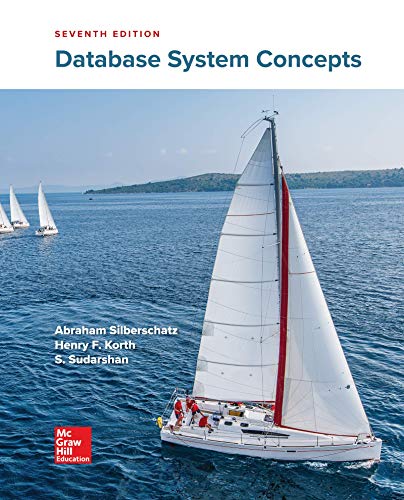
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
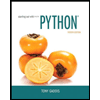
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
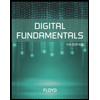
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
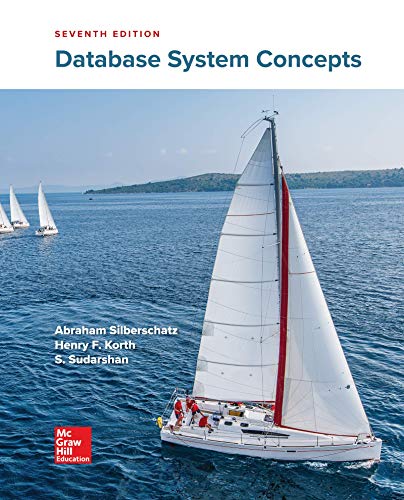
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
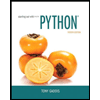
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
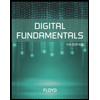
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
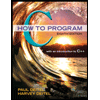
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
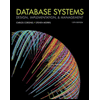
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
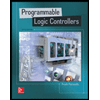
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education