Learning Goals In this lab, you will Use the for OBJ in CONTAINER loop to go over characters in the string Use if statements to compare the strings and characters Use if statements to detect when to output a singular noun Create and increment variables to count (use the accumulator pattern) Use string concatenation to create a new string to assemble the requested output Instructions Write a program that asks the user for a line of text and counts how many spaces, periods, or commas the text contains using the specified output format. Examples If the input is: Hello, world. the output is: There are 1 space, 1 period, and 1 comma. If the input is: Listen, Mr. Jones, calm down. the output is: There are 4 spaces, 2 periods, and 2 commas. Hints Review Figure 6.5.2 to see how to iterate over characters. Go over the string, character by character, and increment the counters for the requested characters. You will need a separate counter for every symbol that you are counting. Do not forget - initially, we do not have any characters counted, so the counter should be 0. You will need a separate check for each symbol to know whether to print its counter with a singular or a plural noun
Learning Goals In this lab, you will Use the for OBJ in CONTAINER loop to go over characters in the string Use if statements to compare the strings and characters Use if statements to detect when to output a singular noun Create and increment variables to count (use the accumulator pattern) Use string concatenation to create a new string to assemble the requested output Instructions Write a program that asks the user for a line of text and counts how many spaces, periods, or commas the text contains using the specified output format. Examples If the input is: Hello, world. the output is: There are 1 space, 1 period, and 1 comma. If the input is: Listen, Mr. Jones, calm down. the output is: There are 4 spaces, 2 periods, and 2 commas. Hints Review Figure 6.5.2 to see how to iterate over characters. Go over the string, character by character, and increment the counters for the requested characters. You will need a separate counter for every symbol that you are counting. Do not forget - initially, we do not have any characters counted, so the counter should be 0. You will need a separate check for each symbol to know whether to print its counter with a singular or a plural noun
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Learning Goals
In this lab, you will
- Use the for OBJ in CONTAINER loop to go over characters in the string
- Use if statements to compare the strings and characters
- Use if statements to detect when to output a singular noun
- Create and increment variables to count (use the accumulator pattern)
- Use string concatenation to create a new string to assemble the requested output
Instructions
Write a program that asks the user for a line of text and counts how many spaces, periods, or commas the text contains using the specified output format.
Examples
If the input is:
Hello, world.
the output is:
There are 1 space, 1 period, and 1 comma.
If the input is:
Listen, Mr. Jones, calm down.
the output is:
There are 4 spaces, 2 periods, and 2 commas.
Hints
- Review Figure 6.5.2 to see how to iterate over characters.
- Go over the string, character by character, and increment the counters for the requested characters.
- You will need a separate counter for every symbol that you are counting.
- Do not forget - initially, we do not have any characters counted, so the counter should be 0.
- You will need a separate check for each symbol to know whether to print its counter with a singular or a plural noun

Transcribed Image Text:**Title: LAB: Count Spaces, Periods, or Commas (Exercise 6.18.1)**
**File: main.py**
This coding exercise is designed to teach how to analyze text input in Python by counting specific characters: spaces, commas, and periods. Below is a transcription of the code framework provided in the lab activity:
```python
1 user_text = input()
2
3 num_spaces = ...
4 num_commas = ...
5 num_periods = ...
6
7 # TODO: for loop to go over each character in user_text
8
9 output = f"There are "
10 if num_spaces == 1:
11 output += f"1 space, "
12 else:
13 output += f"{num_spaces} spaces, "
14
15 # TODO: finish the concatenation of the output string
```
### Code Explanation:
1. **User Input:**
- `user_text = input()` captures text from the user.
2. **Variable Initialization:**
- `num_spaces`, `num_commas`, and `num_periods` are placeholders for counting spaces, commas, and periods, respectively.
3. **Loop Requirement:**
- A comment indicates the need for a `for loop` to iterate through each character in `user_text` to count spaces, commas, and periods.
4. **Output Construction:**
- Begins with a base string assigned to `output`.
- Conditional logic is used to check the number of spaces:
- If there is exactly one space, the string is updated accordingly.
- Otherwise, it indicates the total number of spaces.
5. **Further Steps:**
- A `TODO` comment suggests completion of the output string to include counts for commas and periods.
This exercise provides practice in handling string input and performing character-specific counts. The comments guide students through areas requiring their input for a complete solution.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
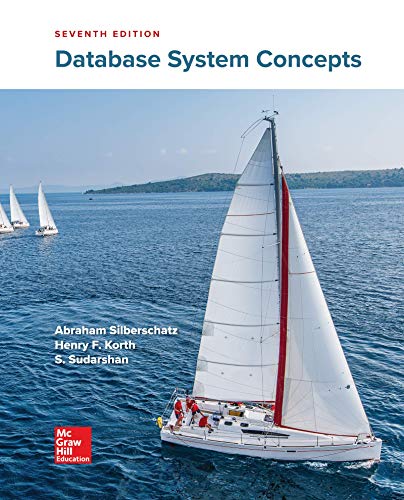
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
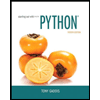
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
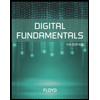
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
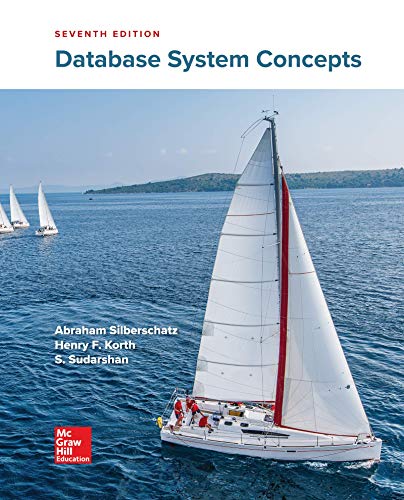
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
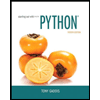
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
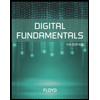
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
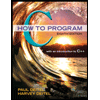
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
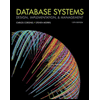
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
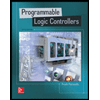
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education