Jump to level 1 Write a while loop that reads integers from input and calculates integer finalNum as follows: • If the input is divisible by 4, add the input divided by 4 to finalNum. • Otherwise, subtract the input multiplied by 4 from finalNum. The loop iterates until a negative integer is read. Ex: If the input is 9 12 4 -5, then the output is: Final number is -37 Note: x % 4 == 0 returns true if x is divisible by 4.
Jump to level 1 Write a while loop that reads integers from input and calculates integer finalNum as follows: • If the input is divisible by 4, add the input divided by 4 to finalNum. • Otherwise, subtract the input multiplied by 4 from finalNum. The loop iterates until a negative integer is read. Ex: If the input is 9 12 4 -5, then the output is: Final number is -37 Note: x % 4 == 0 returns true if x is divisible by 4.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++

Transcribed Image Text:## Challenge Activity: 4.2.2 While Loops
**Jump to level 1**
Write a while loop that reads integers from input and calculates `finalNum` as follows:
- If the input is divisible by 4, add the input divided by 4 to `finalNum`.
- Otherwise, subtract the input multiplied by 4 from `finalNum`.
The loop iterates until a negative integer is read.
**Example**: If the input is `9 12 4 -5`, then the output is:
```
Final number is -37
```
**Note**: `x % 4 == 0` returns true if `x` is divisible by 4.
```cpp
1 #include <iostream>
2 using namespace std;
3
4 int main() {
5 int numInput;
6 int finalNum;
7
8 finalNum = -5;
9 cin >> numInput;
10
11 /* Your code goes here */
12
13 cout << "Final number is " << finalNum << endl;
14
15 return 0;
16 }
```
### Explanation:
In this coding challenge, you are tasked with creating a while loop to process a series of integer inputs. The goal is to adjust a `finalNum` variable based on whether each input is divisible by 4. The loop will terminate when a negative number is input.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
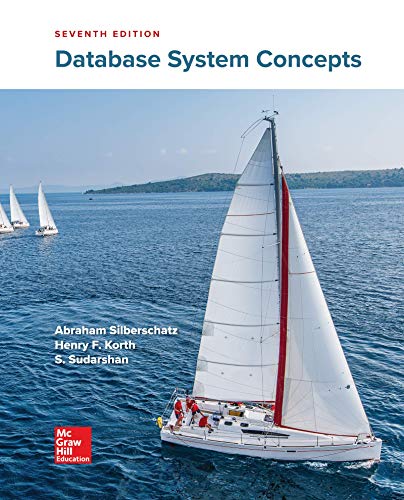
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
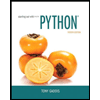
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
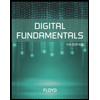
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
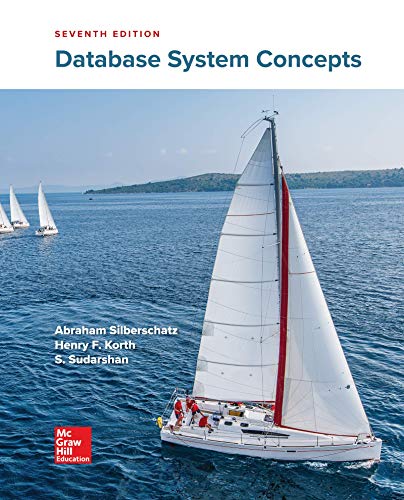
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
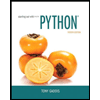
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
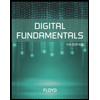
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
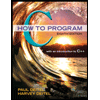
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
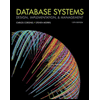
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
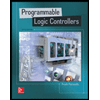
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education