Lab Goal : This lab was designed to teach you how to use a while loop. Lab Description (JAVA PROGRAMMING): Remove all occurrences of the removal string from the original string. Each time you take out the removal string you must also remove the letter that preceded the removal string. After you remove a string and its leading character, you must check to see if any more occurrences of the removal string exist. Sample Data : xR-MxR-MHelloxR-M R-M sxsssxssxsxssexssxsesss xs fuxqwexqwertyxqwexqwe Sample Output : xR-MxR-MHelloxR-M - String to remove R-M Hello sxsssxssxsxssexssxsesss - String to remove xs sesss fuxqwexqwertyxqwexqwertyrtyxqwertyrtyn - String to remove qwerty fun dogdogcatddodogdogdoggog - String to remove dog catgog algorithm help use indexOf to look for more removals while you have more removals { take out the current removal using substring use indexOf to look for more removals } I need help with assignment: "String Cleaner" StringRemover.js: //(c) A+ Computer Science //www.apluscompsci.com //Name - class StringRemover { private String remove; private String sentence; public StringRemover( String sen, String rem ) { } public void removeStrings() { } public String toString() { return ""; } } StringRemoverRunner.js: //(c) A+ Computer Science //www.apluscompsci.com //Name - public class StringRemoverRunner { public static void main( String args[] ) { //add test cases } }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Lab Goal : This lab was designed to teach you how to use a while loop.
Lab Description (JAVA PROGRAMMING): Remove all occurrences of the removal string from the original string. Each time you take out the removal string you must also remove the letter that preceded the removal string. After you remove a string and its leading character, you must check to see if any more occurrences of the removal string exist.
Sample Data :
xR-MxR-MHelloxR-M R-M
sxsssxssxsxssexssxsesss xs
fuxqwexqwertyxqwexqwe
Sample Output : xR-MxR-MHelloxR-M - String to remove R-M Hello sxsssxssxsxssexssxsesss - String to remove xs sesss fuxqwexqwertyxqwexqwertyrtyxqwertyrtyn - String to remove qwerty fun dogdogcatddodogdogdoggog - String to remove dog catgog
use indexOf to look for more removals
while you have more removals
{
take out the current removal using substring
use indexOf to look for more removals
}
I need help with assignment: "String Cleaner"
StringRemover.js:
//(c) A+ Computer Science
//www.apluscompsci.com
//Name -
class StringRemover
{
private String remove;
private String sentence;
public StringRemover( String sen, String rem )
{
}
public void removeStrings()
{
}
public String toString()
{
return "";
}
}
StringRemoverRunner.js:
//(c) A+ Computer Science
//www.apluscompsci.com
//Name -
public class StringRemoverRunner
{
public static void main( String args[] )
{
//add test cases
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

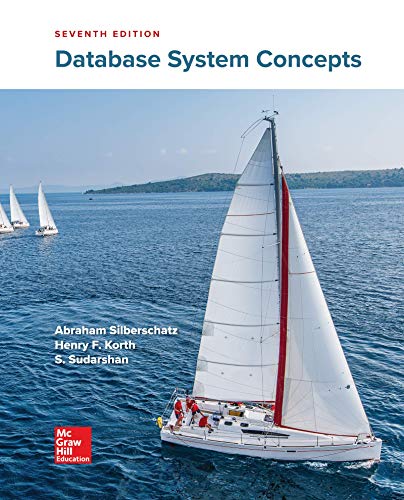
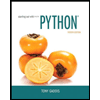
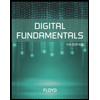
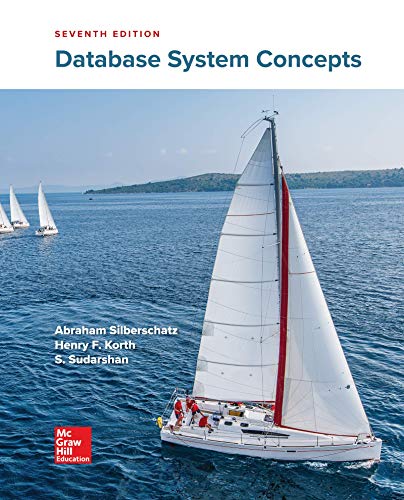
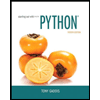
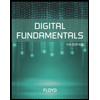
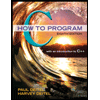
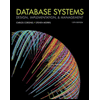
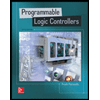