• Try different n values, and compare the execution times of two functions. Please compare the time complexity of two functions and explain which function is better when n is large and why this function is better, add your answer as comment at the beginning of lab04.cpp. The sample run: $ g++ lab04.cpp -o lab04. $ ./lab04 Please enter x: 3 Please enter n: 10 88573 The execution time is : 4.7292e-05 88573 The execution time is 5.294e-06 $ ./lab04 Please enter x: 3. Please enter n: 100 13969809805403233721 The execution time is 0.000104452 13969809805403233721 The execution time is : 6.633e-06 $ ./lab04 Please enter x: 3 Please enter n: 1000 $ ./lab04 Please enter x: 3 Please enter n: 10000 $ ./lab04 Please enter x: 3 Please enter n: 100000 Lab Exercise The following C++ program lab04.cpp prints the result and execution times of two functions that calculate the geometric series sum using different algorithms. You are supposed to implement two functions to calculate the geometric sum. #include #include #include #include using namespace std; long long int geom_sum1 (int x, int n); long long int geom_sum2 (int x, int n); int main() { int x = 1; } int n = 1; cout << "Please enter x: "; cin >> x; cout << "Please enter n: "; cin >> n; using clock = chrono::steady_clock; clock::time_point start = clock::now(); cout << geom_sum1(x, n) << endl; clock::time_point end = clock::now(); clock: duration time span = end start; double nseconds = double(time_span.count()) * clock::period: : num clock: : period::den; cout << "The execution time is : " << nseconds << endl; start = clock::now(); cout << geom_sum2(x, n) << endl; end = clock::now(); time_span= end - start; nseconds = double(time_span.count()) * clock::period: : num clock: : period::den; cout << "The execution time is : << nseconds; return 0; long long int geom_sum1 (int x, int n) { } long long int geom_sum2 (int x, int n) { }
• Try different n values, and compare the execution times of two functions. Please compare the time complexity of two functions and explain which function is better when n is large and why this function is better, add your answer as comment at the beginning of lab04.cpp. The sample run: $ g++ lab04.cpp -o lab04. $ ./lab04 Please enter x: 3 Please enter n: 10 88573 The execution time is : 4.7292e-05 88573 The execution time is 5.294e-06 $ ./lab04 Please enter x: 3. Please enter n: 100 13969809805403233721 The execution time is 0.000104452 13969809805403233721 The execution time is : 6.633e-06 $ ./lab04 Please enter x: 3 Please enter n: 1000 $ ./lab04 Please enter x: 3 Please enter n: 10000 $ ./lab04 Please enter x: 3 Please enter n: 100000 Lab Exercise The following C++ program lab04.cpp prints the result and execution times of two functions that calculate the geometric series sum using different algorithms. You are supposed to implement two functions to calculate the geometric sum. #include #include #include #include using namespace std; long long int geom_sum1 (int x, int n); long long int geom_sum2 (int x, int n); int main() { int x = 1; } int n = 1; cout << "Please enter x: "; cin >> x; cout << "Please enter n: "; cin >> n; using clock = chrono::steady_clock; clock::time_point start = clock::now(); cout << geom_sum1(x, n) << endl; clock::time_point end = clock::now(); clock: duration time span = end start; double nseconds = double(time_span.count()) * clock::period: : num clock: : period::den; cout << "The execution time is : " << nseconds << endl; start = clock::now(); cout << geom_sum2(x, n) << endl; end = clock::now(); time_span= end - start; nseconds = double(time_span.count()) * clock::period: : num clock: : period::den; cout << "The execution time is : << nseconds; return 0; long long int geom_sum1 (int x, int n) { } long long int geom_sum2 (int x, int n) { }
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
Provide the full C++ code needed for the expected output

Transcribed Image Text:• Try different n values, and compare the execution times of two functions. Please compare the time complexity of two functions and explain which function is better when n is large and why this function is better, add your answer as comment at the beginning of
lab04.cpp.
The sample run:
$ g++ lab04.cpp -o lab04.
$ ./lab04
Please enter x: 3
Please enter n: 10
88573
The execution time is : 4.7292e-05
88573
The execution time is 5.294e-06
$ ./lab04
Please enter x: 3.
Please enter n: 100
13969809805403233721
The execution time is 0.000104452
13969809805403233721
The execution time is : 6.633e-06
$ ./lab04
Please enter x: 3
Please enter n: 1000
$ ./lab04
Please enter x: 3
Please enter n: 10000
$ ./lab04
Please enter x: 3
Please enter n: 100000

Transcribed Image Text:Lab Exercise
The following C++ program lab04.cpp prints the result and execution times of two functions that calculate the geometric series sum using different algorithms. You are supposed to implement two functions to calculate the geometric sum.
#include <iostream>
#include <ctime>
#include <cstdlib>
#include <chrono>
using namespace std;
long long int geom_sum1 (int x, int n);
long long int geom_sum2 (int x, int n);
int main()
{
int x = 1;
}
int n = 1;
cout << "Please enter x: ";
cin >> x;
cout << "Please enter n: ";
cin >> n;
using clock =
chrono::steady_clock;
clock::time_point start = clock::now();
cout << geom_sum1(x, n) << endl;
clock::time_point end = clock::now();
clock: duration time span = end start;
double nseconds = double(time_span.count()) * clock::period: : num clock: : period::den;
cout << "The execution time is : " << nseconds << endl;
start = clock::now();
cout << geom_sum2(x, n) << endl;
end =
clock::now();
time_span= end - start;
nseconds = double(time_span.count()) * clock::period: : num clock: : period::den;
cout << "The execution time is : << nseconds;
return 0;
long long int geom_sum1 (int x, int n)
{
}
long long int geom_sum2 (int x, int n)
{
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
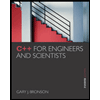
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
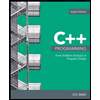
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
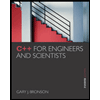
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
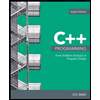
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning