Lab 14.1 Beginning to build an Araxliat recnraively In this sequence of problems we practice recursion by abandoning our reliance on iteration. We resolve to solve a sequence of problems without using while or for loops. Instead we will think recursively and look at the world through a different lens. Recursion is all about solving a large problem by using the solution to a similar smaller problem. The brilliant thing about recursion is that you can assume you already know how to solve the smaller problem. The goal is to demonstrate how the smaller solution relates to the larger problem at hand. For example, suppose you want to print all binary strings of length 3 and you already know how to print all binary strings of length 2. Here they are: 00 01 10 11
Lab 14.1 Beginning to build an Araxliat recnraively In this sequence of problems we practice recursion by abandoning our reliance on iteration. We resolve to solve a sequence of problems without using while or for loops. Instead we will think recursively and look at the world through a different lens. Recursion is all about solving a large problem by using the solution to a similar smaller problem. The brilliant thing about recursion is that you can assume you already know how to solve the smaller problem. The goal is to demonstrate how the smaller solution relates to the larger problem at hand. For example, suppose you want to print all binary strings of length 3 and you already know how to print all binary strings of length 2. Here they are: 00 01 10 11
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Lab 14.1 Beginning to build an Arrazlizt recursively
In this sequence of problems we practice recursion by abandoning our reliance on iteration. We resolve to solve a
sequence of problems without using while or for loops. Instead we will think recursively and look at the world
through a different lens.
Recursion is all about solving a large problem by using the solution to a similar smaller problem. The brilliant thing
about recursion is that you can assume you already know how to solve the smaller problem. The goal is to
demonstrate how the smaller solution relates to the larger problem at hand. For example, suppose you want to
print all binary strings of length 3 and you already know how to print all binary strings of length 2. Here they are:
00
01
10
11
How can we solve the larger problem with a list of strings of length 2? Add a "0" or "1", right? So here is the
solution to the larger problem:
00 + 0 = 000
01 + 0 = 010
10 + 0 = 100
11 +0 = 110
and
00 + 1 = 001
01 + 1 = 011
10 + 1 = 101
11 +1 = 111 Voila!
Not every binary string problem can be solved in terms of a smaller one. At some point we haye to declare the
smallest problem we are interested in and just solve it. In the case of binary strings, we might agree that the
smallest problem we will solve is the 1 digit problem and that the solution is simply 0 and 1. When we write
solutions to problems using recursion we will always solve the smallest problem first, so our programs will usually
have a similar structure:
if this is the smallest problem
Just solve it by hand.
else
Solve the large problem by using a solution to a smaller version of the same problem.
Now let's tackle a real problem. Suppose our goal is to produce an arzazList with Integer objects 1, 2, 3, ., n by
building a method called makelistlint n). We want to end up with a stand-alone method so we will package the
method as a static method. Remember we are refusing to use while and for loops - think recursively!
Here is the skeleton code:
import jakautil.;
public class Listletheds
{
public static aEKaxLiat Integer> makelistlint n)
{
AEKaztist<Integer> tesList = null;
if (n <= 0)
// The smallest list we can make
![}
else
{
// All other size lists are created here
}
return temslist:
The smallest problem we will solve is building and returning an arzaxkiat when n <= 0. In that case we want to
return an empty arravlist. Leave the else block empty for the moment and write the code that will solve this
smallest problem. Test it using the test harness listed below:
import javautiditKaxtiat:
public class ListlethedsRwnner.
{
public static void main (String [] args)
ListMethodsmakelist (0);
aEKazkist<Integer> seuskist
if (teelistize () == 0)
%3!
Sxatem.RutBKAntda ("The list is empty");
}
else
for (Integer : teslia)
Lab 14.2 Adding recursion to our Arravlist solution
We left the else block empty in the last problem. Let's fill it in now.
This is where we need to show how we can solve the large problem (building an ALLAvLÄst with 1, 2, 3, ., n) by
using a smaller version of the same problem. What is the next smallest problem? Yes, building an Agzavkist with
1, 2, 3, ., n - 1. How can we do that? Here's the brilliant part: We can solve that problem by calling makelist in
- 1) - the very same method we are trying to write! That's what makes the recursion happen. Whenever a
method calls itself, the method is recursive. By calling the same method again, each instance of a problem is
solved using the solution to a smaller instance. Logically, this process has.to stop somewhere. In the case of
our arxazliat problem, it stops when we call makelistio). This is the problem we solved by hand in Lab 13.1.1.
-
Whenever we call nakelist in - 1) we receive an Arraylist with 1, 2, 3, ..., n - 1. How can we use that to
produce an Ezaviat with 1, 2, 3, ., n?
Add that code to the else block and complete the nakelist method. Test your code with the test harness by
changing the argument that is passed to gakelist, Can you make a list with 100 items?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F47bba70b-d35c-4545-add1-e8862c4403b1%2Fc5586f37-ca3d-48a7-a237-9b637fb2b87a%2Fatfg03w_processed.jpeg&w=3840&q=75)
Transcribed Image Text:}
else
{
// All other size lists are created here
}
return temslist:
The smallest problem we will solve is building and returning an arzaxkiat when n <= 0. In that case we want to
return an empty arravlist. Leave the else block empty for the moment and write the code that will solve this
smallest problem. Test it using the test harness listed below:
import javautiditKaxtiat:
public class ListlethedsRwnner.
{
public static void main (String [] args)
ListMethodsmakelist (0);
aEKazkist<Integer> seuskist
if (teelistize () == 0)
%3!
Sxatem.RutBKAntda ("The list is empty");
}
else
for (Integer : teslia)
Lab 14.2 Adding recursion to our Arravlist solution
We left the else block empty in the last problem. Let's fill it in now.
This is where we need to show how we can solve the large problem (building an ALLAvLÄst with 1, 2, 3, ., n) by
using a smaller version of the same problem. What is the next smallest problem? Yes, building an Agzavkist with
1, 2, 3, ., n - 1. How can we do that? Here's the brilliant part: We can solve that problem by calling makelist in
- 1) - the very same method we are trying to write! That's what makes the recursion happen. Whenever a
method calls itself, the method is recursive. By calling the same method again, each instance of a problem is
solved using the solution to a smaller instance. Logically, this process has.to stop somewhere. In the case of
our arxazliat problem, it stops when we call makelistio). This is the problem we solved by hand in Lab 13.1.1.
-
Whenever we call nakelist in - 1) we receive an Arraylist with 1, 2, 3, ..., n - 1. How can we use that to
produce an Ezaviat with 1, 2, 3, ., n?
Add that code to the else block and complete the nakelist method. Test your code with the test harness by
changing the argument that is passed to gakelist, Can you make a list with 100 items?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 9 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
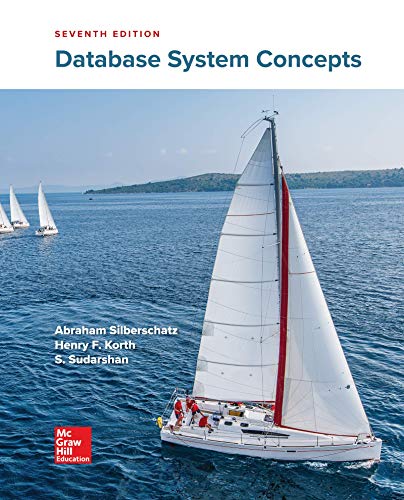
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
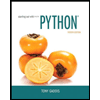
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
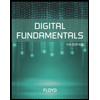
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
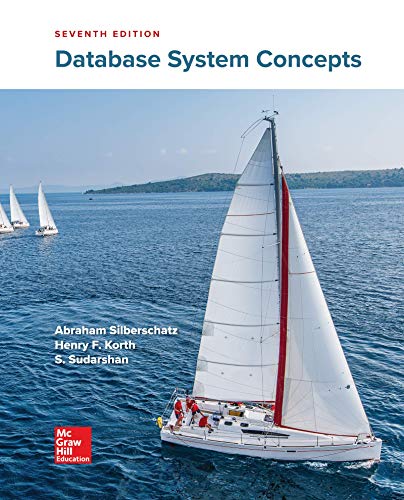
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
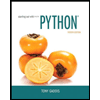
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
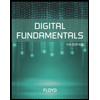
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
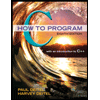
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
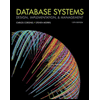
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
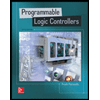
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education