Kindly correct the palindrome validation "MIPS Assembly Reverse" dataprompt: .asciiz "Enter a string: "result: .asciiz "The entered string is a palindrome.\n"not_palindrome: .asciiz "The entered string is not a palindrome.\n" .text.globl main main: # Print prompt li $v0, 4 # Load immediate value 4 la $a0, prompt syscall # Read string from input li $v0, 8 # Load immediate value 8 la $a0, buffer li $a1, 256 syscall # Find string length la $t0, buffer li $t1, 0 loop_length: lb $t2, 0($t0) # Load byte from address in $t0 into $t2 beqz $t2, end_length # If byte is null terminator, exit loop addi $t0, $t0, 1 # Increment pointer to next character addi $t1, $t1, 1 # Increment length counter j loop_length # Repeat loopend_length: # Check for palindrome la $t2, buffer # Load address of buffer into $t2 la $t3, buffer # Load address of buffer into $t3 addi $t3, $t3, $t1 # Move $t3 to end of string subi $t3, $t3, 1 # Adjust for null terminatorcheck_palindrome: beq $t2, $t3, is_palindrome # If pointers meet, it's a palindrome bge $t2, $t3, not_palindrome_output # If pointers have crossed, it's not a palindrome lb $t4, 0($t2) # Load byte from $t2 into $t4 lb $t5, 0($t3) # Load byte from $t3 into $t5 beq $t4, $t5, continue_check # If characters match, continue checking j not_palindrome_output # Otherwise, it's not a palindromecontinue_check: addi $t2, $t2, 1 # Move $t2 to next character subi $t3, $t3, 1 # Move $t3 to previous character j check_palindrome # Repeat loop is_palindrome: # Print result li $v0, 4 la $a0, result syscall j end_program not_palindrome_output: # Print result li $v0, 4 la $a0, not_palindrome syscall end_program: # Exit program li $v0, 10 # Load immediate value 10 (exit program) syscall .databuffer: .space 256
Kindly correct the palindrome validation
"MIPS Assembly Reverse"
data
prompt: .asciiz "Enter a string: "
result: .asciiz "The entered string is a palindrome.\n"
not_palindrome: .asciiz "The entered string is not a palindrome.\n"
.text
.globl main
main:
# Print prompt
li $v0, 4 # Load immediate value 4
la $a0, prompt
syscall
# Read string from input
li $v0, 8 # Load immediate value 8
la $a0, buffer
li $a1, 256
syscall
# Find string length
la $t0, buffer
li $t1, 0
loop_length:
lb $t2, 0($t0) # Load byte from address in $t0 into $t2
beqz $t2, end_length # If byte is null terminator, exit loop
addi $t0, $t0, 1 # Increment pointer to next character
addi $t1, $t1, 1 # Increment length counter
j loop_length # Repeat loop
end_length:
# Check for palindrome
la $t2, buffer # Load address of buffer into $t2
la $t3, buffer # Load address of buffer into $t3
addi $t3, $t3, $t1 # Move $t3 to end of string
subi $t3, $t3, 1 # Adjust for null terminator
check_palindrome:
beq $t2, $t3, is_palindrome # If pointers meet, it's a palindrome
bge $t2, $t3, not_palindrome_output # If pointers have crossed, it's not a palindrome
lb $t4, 0($t2) # Load byte from $t2 into $t4
lb $t5, 0($t3) # Load byte from $t3 into $t5
beq $t4, $t5, continue_check # If characters match, continue checking
j not_palindrome_output # Otherwise, it's not a palindrome
continue_check:
addi $t2, $t2, 1 # Move $t2 to next character
subi $t3, $t3, 1 # Move $t3 to previous character
j check_palindrome # Repeat loop
is_palindrome:
# Print result
li $v0, 4
la $a0, result
syscall
j end_program
not_palindrome_output:
# Print result
li $v0, 4
la $a0, not_palindrome
syscall
end_program:
# Exit program
li $v0, 10 # Load immediate value 10 (exit program)
syscall
.data
buffer: .space 256

Step by step
Solved in 2 steps

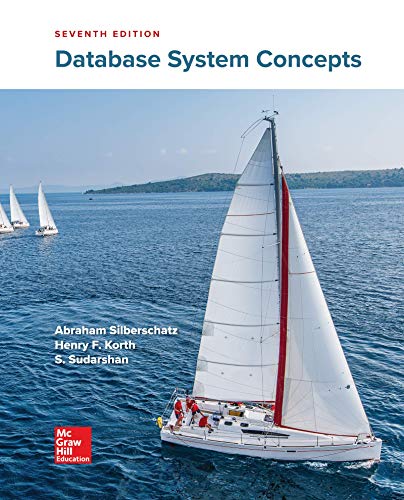
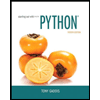
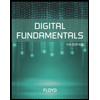
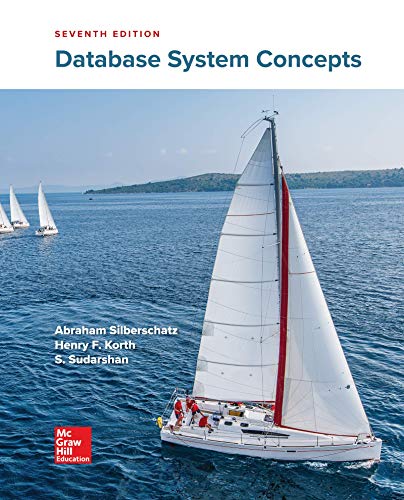
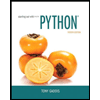
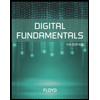
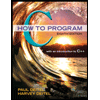
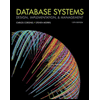
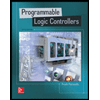