latch buffer.py (continued) import RPi.GPIO as GPIO import time # Import the PRi.GPIO library # Import the Time library GPIO.setmode (GPIO.BCM) GPIO.setwarnings (False) # BCM: Broadcom SOC channel # Do not print GPIO warning messages # Define Decimal (or binary) output pins # Define GPIO pin # 5 for 1's column # Define GPIO pin # 6 for 2's column # Define GPIO pin # 13 for 4's column # Define GPIO pin # 19 for 8's column D1 = 5 D2 = 6. D3 = 13 D4 = 19 GPIO.setup(D1, GPIO.OUT) GPIO.setup (D2, GPIO.OUT) GPIO. setup (D3, GPIO.OUT) GPIO. setup (D4, GPIO.OUT) # Setup D1 for GPIO.OUT # Setup D2 for GPIO.OUT # Setup D3 for GPIO.OUT # Setup D4 for GPIO.OUT # Define Enalbing pins en latch = 16 en buffer = 12 values to BUS - LED will be on # Enable the latches to get the decimal (or binary) value # Enalble the buffers, resulting in forwarding the buffer GPIO.setup(en latch, GPIO.OUT) GPIO.setup (en buffer, GPIO.OUT) # Setup en buffer for GPIO.OUT # Setup en latch for GPIO.OUT
latch buffer.py (continued) import RPi.GPIO as GPIO import time # Import the PRi.GPIO library # Import the Time library GPIO.setmode (GPIO.BCM) GPIO.setwarnings (False) # BCM: Broadcom SOC channel # Do not print GPIO warning messages # Define Decimal (or binary) output pins # Define GPIO pin # 5 for 1's column # Define GPIO pin # 6 for 2's column # Define GPIO pin # 13 for 4's column # Define GPIO pin # 19 for 8's column D1 = 5 D2 = 6. D3 = 13 D4 = 19 GPIO.setup(D1, GPIO.OUT) GPIO.setup (D2, GPIO.OUT) GPIO. setup (D3, GPIO.OUT) GPIO. setup (D4, GPIO.OUT) # Setup D1 for GPIO.OUT # Setup D2 for GPIO.OUT # Setup D3 for GPIO.OUT # Setup D4 for GPIO.OUT # Define Enalbing pins en latch = 16 en buffer = 12 values to BUS - LED will be on # Enable the latches to get the decimal (or binary) value # Enalble the buffers, resulting in forwarding the buffer GPIO.setup(en latch, GPIO.OUT) GPIO.setup (en buffer, GPIO.OUT) # Setup en buffer for GPIO.OUT # Setup en latch for GPIO.OUT
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
PLEASE INCORPORATE THIS IN THE CODE
Infinite Loop
Assert to enter a decimal number (0 – 15)
Convert DEC to BIN
Set the Latches enabled and the Buffers disabled
Set the binary value to the Latches
Wait 2 – 3 seconds
Set the Latches disabled and the Buffers enabled
![## Python Code for Latch Buffer Example
### latch_buffer.py (continued)
```python
A = input("Enter a decimal number to store [0-15]: ")
IN = input("Enable the latches? (0: disable, 1: enable): ")
OUT = input("Enable the buffers? (0: disable, 1: enable): ")
# Convert DEC to BIN
Abin = []
Abin = [0, 0, 0, 0]
n = 0
while A > 0:
Remainder = int(A % 2)
Abin[n] = Remainder
A = int(A / 2)
n = n + 1
print "Binary value for Decimal: "
for x in reversed(Abin):
print(x)
# Set the enable signal for Latch
GPIO.output(en_latch, IN)
# Set the enable signal for Buffer
GPIO.output(en_buffer, OUT)
# Set the binary value to the Latch
GPIO.output(D4, Abin[3])
GPIO.output(D3, Abin[2])
GPIO.output(D2, Abin[1])
GPIO.output(D1, Abin[0])
```
This script is designed to convert a decimal number (0-15) into its binary representation and then send this binary data to a set of outputs that can be enabled or disabled based on user input. The code utilizes the GPIO library for output operations, typically used in hardware scenarios like a Raspberry Pi to interface with I/O pins.
1. **User Input**: It prompts the user for a decimal number, as well as enabling latches and buffers.
2. **Binary Conversion**: A loop converts the decimal number into binary, storing each bit in the `Abin` list.
3. **Output the Binary Value**: The binary equivalent of the input decimal is printed in reverse order.
4. **GPIO Signals**: Depending on the user input, the specified GPIO pins are set with the binary data and enable signals for latches and buffers.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa9ea4650-40bb-48db-9266-4f1ed996eb4b%2F58153e04-8e11-468b-b0f4-12734ab9e01c%2Fuqxt67l_processed.png&w=3840&q=75)
Transcribed Image Text:## Python Code for Latch Buffer Example
### latch_buffer.py (continued)
```python
A = input("Enter a decimal number to store [0-15]: ")
IN = input("Enable the latches? (0: disable, 1: enable): ")
OUT = input("Enable the buffers? (0: disable, 1: enable): ")
# Convert DEC to BIN
Abin = []
Abin = [0, 0, 0, 0]
n = 0
while A > 0:
Remainder = int(A % 2)
Abin[n] = Remainder
A = int(A / 2)
n = n + 1
print "Binary value for Decimal: "
for x in reversed(Abin):
print(x)
# Set the enable signal for Latch
GPIO.output(en_latch, IN)
# Set the enable signal for Buffer
GPIO.output(en_buffer, OUT)
# Set the binary value to the Latch
GPIO.output(D4, Abin[3])
GPIO.output(D3, Abin[2])
GPIO.output(D2, Abin[1])
GPIO.output(D1, Abin[0])
```
This script is designed to convert a decimal number (0-15) into its binary representation and then send this binary data to a set of outputs that can be enabled or disabled based on user input. The code utilizes the GPIO library for output operations, typically used in hardware scenarios like a Raspberry Pi to interface with I/O pins.
1. **User Input**: It prompts the user for a decimal number, as well as enabling latches and buffers.
2. **Binary Conversion**: A loop converts the decimal number into binary, storing each bit in the `Abin` list.
3. **Output the Binary Value**: The binary equivalent of the input decimal is printed in reverse order.
4. **GPIO Signals**: Depending on the user input, the specified GPIO pins are set with the binary data and enable signals for latches and buffers.

Transcribed Image Text:# Python code (1)
## latch_buffer.py (continued)
```python
import RPi.GPIO as GPIO # Import the RPi.GPIO library
import time # Import the Time library
GPIO.setmode(GPIO.BCM) # BCM: Broadcom SOC channel
GPIO.setwarnings(False) # Do not print GPIO warning messages
# Define Decimal (or binary) output pins
D1 = 5 # Define GPIO pin # 5 for 1's column
D2 = 6 # Define GPIO pin # 6 for 2's column
D3 = 13 # Define GPIO pin # 13 for 4's column
D4 = 19 # Define GPIO pin # 19 for 8's column
GPIO.setup(D1, GPIO.OUT) # Setup D1 for GPIO.OUT
GPIO.setup(D2, GPIO.OUT) # Setup D2 for GPIO.OUT
GPIO.setup(D3, GPIO.OUT) # Setup D3 for GPIO.OUT
GPIO.setup(D4, GPIO.OUT) # Setup D4 for GPIO.OUT
# Define Enabling pins
en_latch = 16 # Enable the latches to get the decimal (or binary) value
en_buffer = 12 # Enable the buffers, resulting in forwarding the buffer values to BUS - LED will be on
GPIO.setup(en_latch, GPIO.OUT) # Setup en_latch for GPIO.OUT
GPIO.setup(en_buffer, GPIO.OUT) # Setup en_buffer for GPIO.OUT
```
*Explanation:*
- This script is part of a Python code for controlling GPIO (General Purpose Input/Output) pins on a Raspberry Pi.
- **RPi.GPIO** is a Python library used to control the GPIO pins.
- The pins D1, D2, D3, and D4 are defined to control digital outputs on corresponding GPIO pins.
- **en_latch** and **en_buffer** are enabling pins. When activated, they enable certain operations or data flow in the GPIO circuit, such as driving LEDs.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
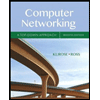
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
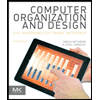
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
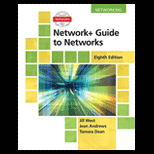
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
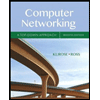
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
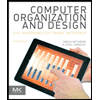
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
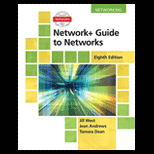
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
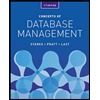
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
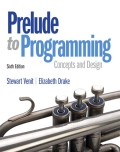
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
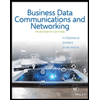
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY