Jerry the Driver File: jerry.py In the Functions 2 lecture, you had a "Do this now" question like: Jerry's car's speedo shows miles (mph) instead of kilometres per hour (kph). He wants to be able to enter his speed in mph, the speed limit in kph and determine his speeding fine. You (we) wrote the pseudocode for the main function for this: function main speed_in_mph = get_valid_number("speed in mph") speed_limit_in_kph = get_valid_number("speed limit in kph") speed_in_kph = convert_miles_to_km(speed_in_mph) fine = determine_fine(speed_in_km, speed_limit_in_kph) print fine Note: The value of the fine as a number type is much better to return than a string, or a whole message. What if we wanted to determine a new bank balance after paying the fine? bank_balance -= fine This is an example of SRP. It is the determine_fine function's ONLY (single responsibility) job to determine/calculate the actual fine as an actual number. It is counter-productive for this function to print the fine or to format with a '$' or anything else. bank_balance -= fine doesn't work if fine is something like Your fine is $199. < insert "you had one job" meme here ;) > Write the complete Python program for this in jerry.py.
1. Jerry the Driver
File: jerry.py
In the Functions 2 lecture, you had a "Do this now" question like:
Jerry's car's speedo shows miles (mph) instead of kilometres per hour (kph). He wants to be able to enter his speed in mph, the speed limit in kph and determine his speeding fine.
You (we) wrote the pseudocode for the main function for this:
function main
speed_in_mph = get_valid_number("speed in mph")
speed_limit_in_kph = get_valid_number("speed limit in kph")
speed_in_kph = convert_miles_to_km(speed_in_mph)
fine = determine_fine(speed_in_km, speed_limit_in_kph)
print fine
Note: The value of the fine as a number type is much better to return than a string, or a whole message.
What if we wanted to determine a new bank balance after paying the fine?
bank_balance -= fine
This is an example of SRP. It is the determine_fine function's ONLY (single responsibility) job to determine/calculate the actual fine as an actual number.
It is counter-productive for this function to print the fine or to format with a '$' or anything else. bank_balance -= fine doesn't work if fine is something like Your fine is $199.
< insert "you had one job" meme here ;) >
Write the complete Python program for this in jerry.py.
Logic Exercise
Read the following out loud:
NORTH QUEENSLAND
IN THE
THE SPRING
Good job. Or was it? Double-check that you said it correctly.
If you didn't... notice that you had trouble with attention to detail.
This is a fairly normal issue, but one that programmers need to deal with more so than 'regular' people.
You need to continue to systematically build your attension to detail ;)
For this question, just write whether you read the statement correctly the first time or not.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

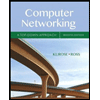
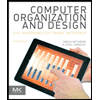
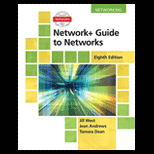
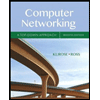
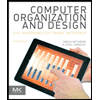
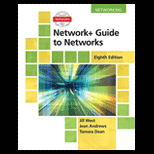
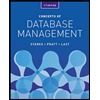
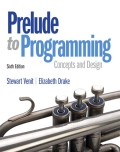
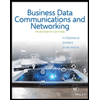