java.util.Date birthDate and the following public instance methods: toString: Returns the animal’s vegetarian, eatings, numOfLegs AND birthDate information as a string The Cat class should also have override toString: Returns the cat’s vegetarian, eatings, numOfLegs, birthDate and color information as a string can you please explain to me why Animal.java class's "set" method doesn't assign an argument of type java.util.Date to birthDate as well as why Animal.java class doesn't have a "get" method for birthDate. Animal.java
The Animal class should also have
java.util.Date birthDate and the following public instance methods:
toString: Returns the animal’s vegetarian, eatings, numOfLegs AND birthDate information as a string
The Cat class should also have
override toString: Returns the cat’s vegetarian, eatings, numOfLegs, birthDate and color information as a string
can you please explain to me why Animal.java class's "set" method doesn't assign an argument of type java.util.Date to birthDate as well as why Animal.java class doesn't have a "get" method for birthDate.
Animal.java
class Animal
{
protected boolean vegetarian;
protected String eatings;
protected int numOfLegs;
public Animal()
{
vegetarian = false;
eatings = "meat";
numOfLegs = 4;
}
public Animal(boolean vegetarian,String eatings, int numOfLegs)
{
this.vegetarian = vegetarian;
this.eatings = eatings;
this.numOfLegs = numOfLegs;
}
public void SetAnimal(boolean vegetarian,String eatings, int numOfLegs)
{
this.vegetarian = vegetarian;
this.eatings = eatings;
this.numOfLegs = numOfLegs;
}
public boolean getVegetarian()
{
return vegetarian;
}
public String getEatings()
{
return eatings;
}
public int getNumOfLegs()
{
return numOfLegs;
}
public String toString()
{
return "Animal’s vegetarian : "+ vegetarian +", eatings : "+ eatings+" number of legs : "+numOfLegs;
}
}
Cat.java
class Cat extends Animal
{
private String color;
public Cat()
{
super();
}
public Cat(boolean vegetarian,String eatings, int numOfLegs, String color)
{
super(vegetarian,eatings,numOfLegs);
this.color = color;
}
public void SetColor(String color)
{
this.color = color;
}
public String GetColor()
{
return color;
}
public String toString()
{
return super.toString() +" color : "+color;
}
}
class testAnimal
{
public static void main (String[] args)
{
Cat e1 = new Cat();
Cat e2 = new Cat(false,"meat,milk",4, "white");
e1.SetAnimal(true,"grass",4);
e1.SetColor("black");
System.out.println(e1);
System.out.println(e2);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

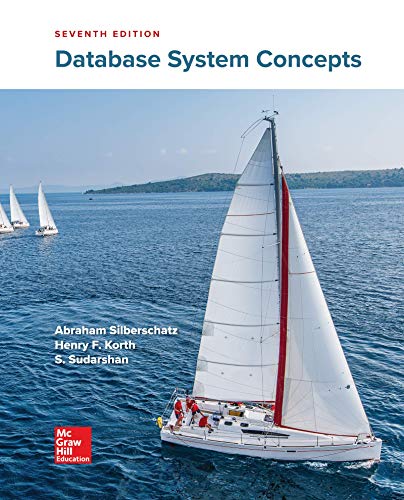
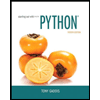
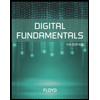
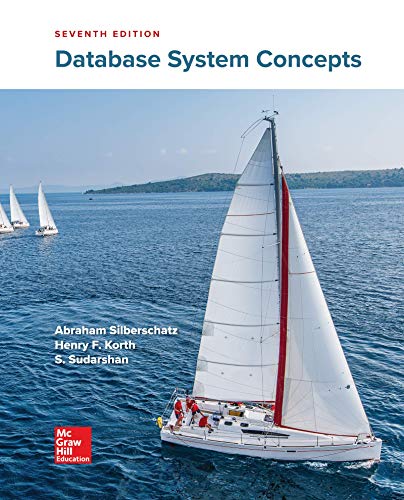
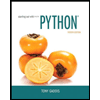
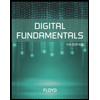
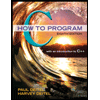
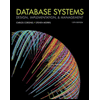
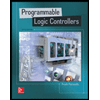