Java Programming: 6.) Acme Parts runs a small factory and employs workers who are paid one of three hourly rates depending on their shift: first shift, $17 per hour; second shift, $18.50 per hour; third shift, $22 per hour. Each factory worker might work any number of hours per week; and hours greater than 40 are paid at one and one-half times the usual rate. In addition, second- and third shift workers can elect to participate in the retirement plan, for which 3 percent of the worker’s gross pay is deducted from the paychecks. Write a program that prompts the user for hours worked and the shift number; if the shift number is 2 or 3, prompt the user to enter the worker’s choice to participate in the retirement plan. Display (1) the hours, (2) the shift, (3) the hourly pay rate, (4) the regular pay, (5) overtime pay, (6) the total of regular and overtime pay, (7) the retirement deduction, if any, and (8) the net pay. Save the file as AcmePay.java. Input/output would be appreciated!
Java Programming: 6.) Acme Parts runs a small factory and employs workers who are paid one of three hourly rates depending on their shift: first shift, $17 per hour; second shift, $18.50 per hour; third shift, $22 per hour. Each factory worker might work any number of hours per week; and hours greater than 40 are paid at one and one-half times the usual rate. In addition, second- and third shift workers can elect to participate in the retirement plan, for which 3 percent of the worker’s gross pay is deducted from the paychecks. Write a program that prompts the user for hours worked and the shift number; if the shift number is 2 or 3, prompt the user to enter the worker’s choice to participate in the retirement plan. Display (1) the hours, (2) the shift, (3) the hourly pay rate, (4) the regular pay, (5) overtime pay, (6) the total of regular and overtime pay, (7) the retirement deduction, if any, and (8) the net pay. Save the file as AcmePay.java. Input/output would be appreciated!
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Java Programming:
6.) Acme Parts runs a small factory and employs workers who are paid one of three hourly rates depending on their shift: first shift, $17 per hour; second shift, $18.50 per hour; third shift, $22 per hour. Each factory worker might work any number of hours per week; and hours greater than 40 are paid at one and one-half times the usual rate. In addition, second- and third shift workers can elect to participate in the retirement plan, for which 3 percent of the worker’s gross pay is deducted from the paychecks. Write a program that prompts the user for hours worked and the shift number; if the shift number is 2 or 3, prompt the user to enter the worker’s choice to participate in the retirement plan. Display (1) the hours, (2) the shift, (3) the hourly pay rate, (4) the regular pay, (5) overtime pay, (6) the total of regular and overtime pay, (7) the retirement deduction, if any, and (8) the net pay. Save the file as AcmePay.java.
Input/output would be appreciated!

Transcribed Image Text:Certainly! Here’s the transcribed text and an explanation suitable for an educational website.
---
**Transcription:**
5. **a.** Write an application that uses the LocalDate class to access the current date. Prompt a user for a month, day, and year, then display a message that specifies whether the entered date is (1) not this year, (2) in an earlier month this year, (3) in a later month this year, or (4) this month. Save the file as **PastPresentFuture.java**.
**b.** Use the Web to learn how to use the LocalDate Boolean methods isBefore(), isAfter(), and equals(). Use your knowledge to write a program that prompts a user for a month, day, and year, and then displays a message specifying whether the entered day is in the past, the current date, or in the future. Save the file as **PastPresentFuture2.java**.
6. Acme Parts runs a small factory and employs workers who are paid one of three hourly rates, depending on their shift: first shift, $17 per hour; second shift, $20 per hour; third shift, $22 per hour. Each factory worker might work any number of hours per week; however, hours greater than 40 are paid at one and one-half times the usual rate. In addition, second- and third-shift workers can elect to participate in the retirement plan, for which ...
4. Write a program for Horizon Phone, a provider of cellular phone service, to prompt a user for maximum monthly values for talk minutes and gigabytes of data needed, and then display a recommendation for the user to purchase either Plan A at $65 per month for any number of minutes of talk and texts, and 1 gigabyte of data; or Plan B at $75 per month for 1000 text messages, more talk, or Plan C for 3 gigabytes more at $80 per month ...
---
**Diagram/Graph Explanation:**
There are no graphs or diagrams visible in the image. The text consists of assignments related to programming and logical problem-solving tasks that involve Java applications and learning how to handle date/time functionalities and logical conditions. The focus is on creating Java applications to handle different scenarios such as working with dates and computing rates for factory workers.
If there is more information or specific questions, feel free to ask!
![### Transcription of AcmePay.java on Eclipse IDE
**File Structure:**
- **Project:** Chapter5
- **Source Folder (default package):**
- AcmePay.java
- ShadyRestRoom.java
- ShadyRestRoom2.java
- **JRE System Library:** [JavaSE-1.8]
- Person.java
**Code in AcmePay.java:**
```java
public class AcmePay {
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
```
### Explanation
The code structure shown is a basic setup for a Java class named `AcmePay`. It includes:
- **public class AcmePay:** This declares a public class named AcmePay. This is the starting point of creating a Java class.
- **public static void main(String[] args):** This is the main method where the program execution begins. It is currently a placeholder with a comment indicating that further implementation is needed.
- **// TODO Auto-generated method stub:** This comment is an auto-generated reminder for the developer to fill in the code for the main method.
### Graphs or Diagrams
There are no graphs or diagrams present in the image.
### Additional Information
- The file structure and content are displayed in the Eclipse IDE, a popular development environment for Java.
- The project seems to be part of a chapter exercise, likely within a Java programming course.
#### Note:
The bottom bar of the IDE outlines various system utilities and weather information like:
- **Temperature:** 69°F
- **Weather:** Mostly sunny
This setup provides a foundational starting point for learners to add functionality to the `AcmePay` class.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb9f0bdf4-572f-4133-8b3a-121eaba2f02a%2F953da246-5f0b-47aa-a0a9-68033504b490%2Ft3v2li_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Transcription of AcmePay.java on Eclipse IDE
**File Structure:**
- **Project:** Chapter5
- **Source Folder (default package):**
- AcmePay.java
- ShadyRestRoom.java
- ShadyRestRoom2.java
- **JRE System Library:** [JavaSE-1.8]
- Person.java
**Code in AcmePay.java:**
```java
public class AcmePay {
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
```
### Explanation
The code structure shown is a basic setup for a Java class named `AcmePay`. It includes:
- **public class AcmePay:** This declares a public class named AcmePay. This is the starting point of creating a Java class.
- **public static void main(String[] args):** This is the main method where the program execution begins. It is currently a placeholder with a comment indicating that further implementation is needed.
- **// TODO Auto-generated method stub:** This comment is an auto-generated reminder for the developer to fill in the code for the main method.
### Graphs or Diagrams
There are no graphs or diagrams present in the image.
### Additional Information
- The file structure and content are displayed in the Eclipse IDE, a popular development environment for Java.
- The project seems to be part of a chapter exercise, likely within a Java programming course.
#### Note:
The bottom bar of the IDE outlines various system utilities and weather information like:
- **Temperature:** 69°F
- **Weather:** Mostly sunny
This setup provides a foundational starting point for learners to add functionality to the `AcmePay` class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
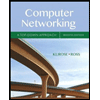
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
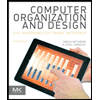
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
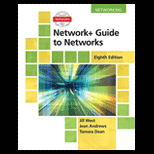
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
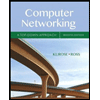
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
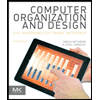
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
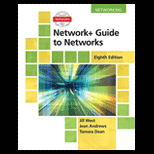
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
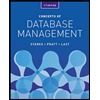
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
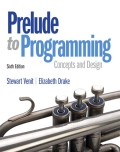
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
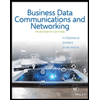
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY